Create three more classes that inherit from Crew. Unless noted otherwise, each of these classes has the same instance variables as Crew, so don’t override the __init__ method.
Create three more classes that inherit from Crew. Unless noted otherwise, each of these classes has the same instance variables as Crew, so don’t override the __init__ method.
Chapter8: Arrays
Section: Chapter Questions
Problem 7PE
Related questions
Question
Create three more classes that inherit from Crew. Unless noted otherwise, each of these classes has the same instance variables as Crew, so don’t override the __init__ method.
- Engineer(Crew):
- The only method that should be overridden in the Engineer class is the repair method.
- repair(self, ship) takes in self and a Starship object, and represents an attempt by the Engineer to repair a section of the ship.
- If the engineer’s current location is damaged (that is, ship.damaged for the location is True), then the value for that key should be changed to False, and a message should print out of the form:
<Name> fixed the damage to <Location>.
- If the engineer’s current location is NOT damaged, then instead the method should print a message of the form:
<Location> isn’t damaged.
- Captain(Crew):
- The only method that should be overridden in the Captain class is the fire_lasers method. This method represents an attempt by the captain to fire lasers at another ship.
- fire_lasers(self, ship, target_ship, target_location) takes in self, two Starship objects ship and target_ship (the first is the one the captain is firing from, and the second is the starship the captain is firing at), and target_location, which is a string representing where on the target ship the captain is aiming the lasers.
- If target_location is not one of the five valid location options ("Bridge", "Medbay", "Engine", "Lasers", or "Sleep Pods"), the method should print out the message:
Not a valid location.
- If the captain is not currently located in the Bridge, then the method should print out the message:
<Name> must be in the Bridge to fire lasers.
- If the Bridge of the captain’s ship is damaged, the method should print out the message:
Bridge is too damaged to order lasers to be fired.
- If the Lasers of the captain’s ship are damaged, the method should print out the message:
Lasers are too damaged to fire.
- If none of the above apply, then the captain successfully fires lasers at the target ship. This should cause the target location on the target ship to be damaged, and should cause any crew members in that location on the target ship to have their status set to "Injured". The method should also print out a message of the form:
<Ship Name> fires lasers at <Target>'s <Location>.
- Doctor(Crew):
- Doctor has one additional instance variable not present in the Crew class: self.medpacs. This is an integer representing the quantity of medical supplies that the doctor is carrying at the moment. This should be initialized to 3.
- Note that because we require an additional instance variable, you will need to override __init__ for the Doctor class. Be sure to call Crew.__init__ from within Doctor.__init__ to avoid re-writing the code to set up the other instance variables.
- Aside from __init__, the only other method Doctor should override is first_aid.
- first_aid(self, ship) takes in self and a Starship object, and represents an attempt by the doctor to heal the injuries of everyone that’s in the same location as the Doctor.
- Using first_aid should reduce the doctor’s supply of medpacs by 1. If the doctor already has 0 medpacs, the method should print out a message of the form:
<Name> has no medpacs left.
- There is an exception to this - if the doctor uses this method while their current location is in the Medbay, AND the Medbay is not damaged, then instead of decreasing the number of medpacs by 1, this should replenish the doctor’s supply of medpacs back to 3. If this happens, then it should print out the message:
<Name>'s supply of medpacs has been replenished.
- Assuming that the doctor has at least one medpac (or is currently in an undamaged Medbay), any crew members in the same location as the doctor with a status of 'Injured' should have their status changed to 'Active', and a message should print out of the form:
<Doctor's Name> healed <Injured Crew Name>'s injuries.
- Note that one medpac is used per call to first_aid (unless the doctor is in an undamaged Medbay), regardless of how many injured crew members are present to heal. Even if the doctor is alone and there is no one to heal, it still uses one medpac.

Transcribed Image Text:hw12.py > Starship
1 class Crew:
2
∞ SAW N
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
def __init__(self, name):
self.name = name
self.status = 'Active'
self.location = 'Sleep Pods'
def ___repr__(self):
return f'{self.name}: {self.status}, at {self.location}'
def move(self, location):
if location in ("Bridge", "Medbay", "Engine", "Lasers", "Sleep Pods"):
self.location = location
else:
print("Not a valid location.")
def repair(self, ship):
print("%s doesn't know how to do that." % self.name)
def first aid (self, ship):
print("%s doesn't know how to do that. % self.name)
def fire_lasers(self, ship, target_ship, target_location):
print("%s doesn't know how to do that.'
." % self.name)
class Starship:
def __init__(self,name, crew_list):
self.name = name
self.crew_list = crew_list
self.damaged {'Bridge': False, 'Medbay': False, 'Engine': False, 'Lasers'
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
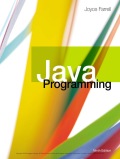
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
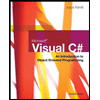
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
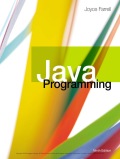
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
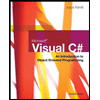
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,