Create a base class called Entity. The Entity class must hold the common attributes and behaviors from UML.
Create a base class called Entity. The Entity class must hold the common attributes and behaviors from UML.
Entity Class UML
Entity
-id: long
-name: String
--------------------------------
-Enity()
+Entity(id: long, name: String)
+getId(): long
+getName(); String
+toString(): String
Complete the code for the Player and Team classes. Each class must derive from the Entity class.
Refactor the Game class to inherit from this new Entity class.
Every team and player must have a unique name by searching for the supplied name prior to adding the new instance. Use the iterator pattern in the addTeam() and addPlayer() methods.
Code that I have, not sure if I have it correct. Thank you
Entity Class
package com.gamingroom;
public class Entity {
//Private attributes
private long id;
private String name;
//Default constructor
public Entity() {
}
//Constructor with an identifier and name
public Entity(long id, String name) {
this();
this.id = id;
this.name = name;
}
//@return the id
public long getId() {
return id;
}
//@return the name
public String getName() {
return name;
}
@Override
public String toString() {
return "Entity [id=" + id + ", name=" + name + "]";
}
}
Game class
package com.gamingroom;
import java.util.ArrayList;
//Extends Entity
public class Game extends Entity{
long id;
String name;
//Hide the default constructor to prevent creating empty instances.
//A list of the active teams
private List<Team> teams = new ArrayList<Team>();
private Game() {
}
//Constructor with an identifier and name
public Game(long id, String name) {
this();
this.id = id;
this.name = name;
}
//@return the id
public long getId() {
return id;
}
//@return the name
public String getName() {
return name;
}
//Uses iterator pattern to find existing team with same name or adds unique named team to list
public Team addTeam(String name) {
// a local team instance
Team team = null;
// Instance iterator
Iterator<Team> teamsIterator = teams.iterator();
// Iterate over teams list
while (teamsIterator.hasNext()) {
// Set local Team var to next item in list
Team teamInstance = teamsIterator.next();
// Does team name already exist?
if (teamInstance.getName().equalsIgnoreCase(name)) {
// If team name already exists, return the team instance
team = teamInstance;
} else {
// else add the team to the teams list
teams.add(team);
}
}
return team;
}
@Override
public String toString() {
return "Game [id=" + id + ", name=" + name + "]";
}
}
Team class
package com.gamingroom;
import java.util.ArrayList;
//Extends Entity
public class Team extends Entity {
long id;
String name;
//List of active teams
private List<Player> players = new ArrayList<Player>();
// Constructor with an identifier and name
public Team(long id, String name) {
this.id = id;
this.name = name;
}
//@return the id
public long getId() {
return id;
}
//@return the name
public String getName() {
return name;
}
//Uses iterator pattern to find existing player with same name or adds unique named player to list
public Player addPlayer(String name) {
// a local teams instance
Player player = null;
// Instance iterator
Iterator<Player> playersIterator = players.iterator();
// Iterate over players list
while (playersIterator.hasNext()) {
// Set local Player var to next item in list
Player playerInstance = playersIterator.next();
// Does player name already exist?
if (playerInstance.getName().equalsIgnoreCase(name)) {
// If player name already exists, return the player instance
player = playerInstance;
} else {
// else add the player to the players list
players.add(player);
}
}
return player;
}
@Override
public String toString() {
return "Team [id=" + id + ", name=" + name + "]";
}
}
Player class
package com.gamingroom;
//Extends Entity
public class Player extends Entity {
long id;
String name;
//Constructor with an identifier and name
public Player(long id, String name) {
this.id = id;
this.name = name;
}
//@return the id
public long getId() {
return id;
}
//@return the name
public String getName() {
return name;
}
@Override
public String toString() {
return "Player [id=" + id + ", name=" + name + "]";
}
}
Program Driver
package com.gamingroom;
//Application start-up program
public class ProgramDriver {
// The one-and-only main() method @param args command line arguments
public static void main(String[] args) {
//obtain reference to the singleton instance
GameService service = GameService.getInstance();
System.out.println("\nAbout to test initializing game data...");
// initialize with some game data
Game game1 = service.addGame("Game #1");
System.out.println(game1);
Game game2 = service.addGame("Game #2");
System.out.println(game2);
// use another class to prove there is only one instance
SingletonTester tester = new SingletonTester();
tester.testSingleton();
}
}
Singleton tester
package com.gamingroom;
//A class to test a singleton's behavior
public class SingletonTester {
public void testSingleton() {
System.out.println("\nAbout to test the singleton...");
//Obtain local reference to the singleton instance
GameService service = GameService.getInstance();
// a simple for loop to print the games
for (int i = 0; i < service.getGameCount(); i++) {
System.out.println(service.getGame(i));
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

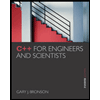
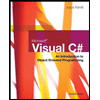
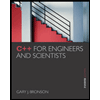
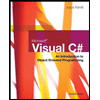