Complete the implementations of the abstract class LinkedList.java, which implements the List.java interface and the class LinkedOrderedList.java, which extends the abstract class LinkedList.java and implements theOrderedList.java interface. LinkedList methods to implement: removeFirst removeLast remove contains LinkedOrderedList method to implement: add Recommended order of development: add to empty list to end of list to front of list to middle of list contains removeFirst removeLast remove Write a test class that tests all of the methods you implemented. Note: You only need to test LinkedOrderedList, because all of the methods are implemented in that class or inherited from LinkedList.
Complete the implementations of
- the abstract class LinkedList.java, which implements the List.java interface and
- the class LinkedOrderedList.java, which extends the abstract class LinkedList.java and implements theOrderedList.java interface.
LinkedList methods to implement:
- removeFirst
- removeLast
- remove
- contains
LinkedOrderedList method to implement:
- add
Recommended order of development:
- add
- to empty list
- to end of list
- to front of list
- to middle of list
- contains
- removeFirst
- removeLast
- remove
Write a test class that tests all of the methods you implemented. Note: You only need to test LinkedOrderedList, because all of the methods are implemented in that class or inherited from LinkedList.
Although you are not required to submit the test class, you might be asked to do so if you are having difficulties getting your implementation to work.
Submit the completed LinkedList.java and LinkedOrderedList.java.
LinkedList.java code
import java.util.NoSuchElementException;
import java.util.StringJoiner;
public abstract class LinkedList<E> implements List<E> {
protected class Node {
E element;
Node next;
Node(E element) {
this(element, null);
}
Node(E element, Node next) {
this.element = element;
this.next = next;
}
}
protected int count;
protected Node head;
protected Node tail;
/**
* Creates an empty list.
*/
public LinkedList() {
count = 0;
head = null;
tail = null;
}
/**
* Removes and returns the first element from the list.
*
* @return the first element of the list
* @throws IllegalStateException if the list is empty
*/
@Override
public E removeFirst() {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return null;
}
/**
* Removes and returns the last element from the list.
*
* @return the last element in the list
* @throws IllegalStateException if the list is empty
*/
@Override
public E removeLast() {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return null;
}
/**
* Removes and returns the specified element from the list.
*
* @param targetElement the element to be removed from the list
* @return the removed element
* @throws IllegalStateException if the list is empty
* @throws NoSuchElementException if the target element is not found
*/
@Override
public E remove(E targetElement) {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return null;
}
/**
* Returns the first element in the list without removing it.
*
* @return the first element in the list
* @throws IllegalStateException if the list is empty
*/
@Override
public E first() {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return head.element;
}
/**
* Returns the last element in the list without removing it.
*
* @return the last element in the list
* @throws IllegalStateException if the list is empty
*/
@Override
public E last() {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return tail.element;
}
/**
* Returns true if the specified element is found in the list.
*
* @param targetElement the element that is sought in the list
* @return true if the element is found in the list
* @throws IllegalStateException if the list is empty
*/
@Override
public boolean contains(E targetElement) {
if (isEmpty()) {
throw new IllegalStateException("The LinkedList is empty.");
}
return false;
}
/**
* Returns true if the list contains no elements.
*
* @return true if the list contains no elements
*/
@Override
public boolean isEmpty() {
return (count == 0);
}
/**
* Returns the number of elements in the list.
*
* @return the number of elements in the list
*/
@Override
public int size() {
return count;
}
/**
* Returns a string representation of the list.
*
* @return a string representation of the list
*/
@Override
public String toString() {
StringJoiner result = new StringJoiner(", ", "[", "]");
Node current = head;
while (current != null) {
result.add(current.element.toString());
current = current.next;
}
return result.toString();
}
}
Thank you for any help/suggestions!



Trending now
This is a popular solution!
Step by step
Solved in 4 steps

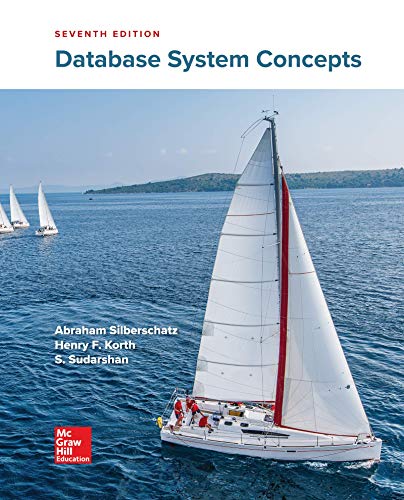
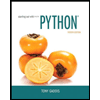
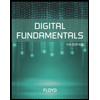
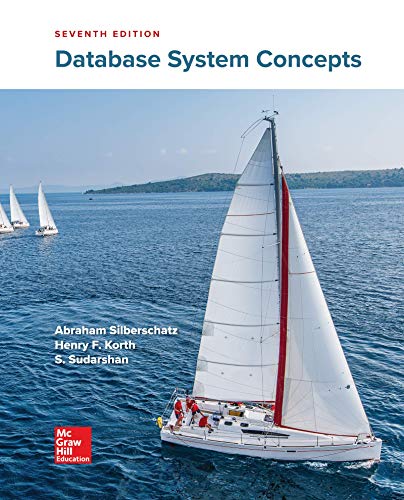
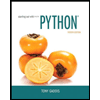
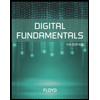
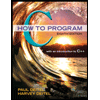
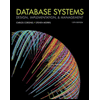
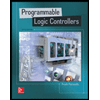