code rectangel
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
code rectangel
#include <iostream>
using namespace std;
class Rectangle{
private:
double width;
double length;
static int numberOfObjects;
public:
Rectangle();
Rectangle(double , double);
~Rectangle(){cout<<"Destructor called "<<endl;}
static void printNumberOfObjects(){
cout<<"number of objects: "<<endl;}
void setDimention(double, double);
double getWidth();
double getLength();
double getArea();
void printRectangle() const ;
double getPerimeter();
};
int Rectangle::numberOfObjects=0;
Rectangle::Rectangle(){
/*width = 0;
length = 0;*/
cout<<"Default constructor called"<<endl;
setDimention(0,0);
numberOfObjects++;
}
Rectangle::Rectangle(double w, double l){
/* if(w>=0)// validation on the input
width= w;
else
width=0;
if(l>=0)
length = l;
else
length= 0;*/
cout<<"constructor with parameters called"<<endl;
setDimention(w,l);
numberOfObjects++;
}
void Rectangle::setDimention(double W, double L){
if(W>=0)// validation on the input
width= W;
else
width=0;
if(L>=0)
length = L;
else
length= 0;
// printRectangle(); we can call the other func directly
}
double Rectangle::getWidth(){
return width;
}
double Rectangle::getLength(){
return length;
}
double Rectangle::getArea(){
return width*length;
}
double Rectangle::getPerimeter(){
return 2*(width+length);
}
void Rectangle::printRectangle()const {
// width = 5; error
cout<<"The rectangle width is :"<<width<<" and the length is : "<<length<<endl;
}
void print(const Rectangle& r){
cout<<"printing from a func:";
r.printRectangle();
}
int main()
{
/*
Rectangle rect1;
// rect1.setDimention(5,10);
rect1.printRectangle();
cout<<"width = "<<rect1.getWidth()<<" length ="<<rect1.getLength()<<endl;
//set the values from the user:
double w,l;
cout<<"Please enter w: ";
cin>>w;
cout<<"Please enter l: ";
cin>>l;
rect1.setDimention(w,l);
rect1.printRectangle();
//set values from other object
Rectangle rect2;
rect2 = rect1;
cout<<"rectangle 2 info:"<<endl;
rect2.printRectangle();
double area = rect1.getArea();
cout<<"the area of the rectangle 1 is:"<<area<<endl;
double perimeter = rect1.getPerimeter();
cout<<"the perimeter of the rectangle 1 is:"<<perimeter<<endl;
print(rect2);
Rectangle rect3(15,16);
rect3.printRectangle();*/
Rectangle arrayRect[5];
double w, l;
for (int i = 0;i< 5;i++){
cout<<"Please enter a value for Rectangle"<<i<<" width & length"<<endl;
cin>>w; cin>>l;
arrayRect[i].setDimention(w,l);
}
for(int i = 0;i<5;i++){
cout<<"Rectangle "<<i<<" info: "<<endl;
arrayRect[i].printRectangle();
}
Rectangle::printNumberOfObjects();
arrayRect[0].printNumberOfObjects();
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

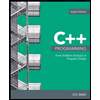
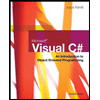
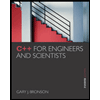
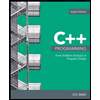
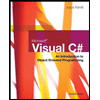
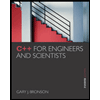
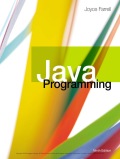