Code in main() takes a space-separated list of numbers and inserts each into a Sorted NumberList. The list is displayed after each insertion. Ex: If input is 77 15 42 63.5 then output is: List after inserting 77: 77 List after inserting 15: 15 77 List after inserting -42: -42 15 77 List after inserting 63.5: -42 15 63.5 77 Try various program inputs, ensuring that each outputs a sorted list.
I need help with this Java problem to output as it's explained in this image below:
Ex: If input is
84 72 19 61
19 84
then output is:
List after inserting 84:
84
List after inserting 72:
72 84
List after inserting 19:
19 72 84
List after inserting 61:
19 61 72 84
List after removing 19:
61 72 84
List after removing 84:
61 72
LabProgram.java code below (Needs editing)
import java.util.Scanner;
import java.io.PrintWriter;
public class LabProgram {
// Prints the SortedNumberList's contents, in order from head to tail
public static void printList(SortedNumberList list) {
Node node = list.head;
while (null != node) {
System.out.print(node.getData() + " ");
node = node.getNext();
}
System.out.println();
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String inputLine;
// Read the line of input numbers
inputLine = scnr.nextLine();
// Split on space character
String[] terms = inputLine.split(" ");
// Insert each value and show the sorted list's contents after each insertion
SortedNumberList list = new SortedNumberList();
for (Object term : terms) {
double number = Double.parseDouble(term.toString());
System.out.println("List after inserting " + number + ": ");
list.insert(number);
printList(list);
}
/*
// Read the input line with numbers to remove
inputLine = scnr.nextLine();
terms = inputLine.split(" ");
for (Object term : terms) {
double number = Double.parseDouble(term.toString());
System.out.println("List after removing " + number + ": ");
list.remove(number);
printList(list);
}
*/
}
}
Node.java code below (Don't edit)
public class Node {
protected double data;
protected Node next;
protected Node previous;
// Constructs this node with the specified numerical data value. References to the next
// and previous nodes are assigned null.
public Node(double initialData) {
data = initialData;
next = null;
previous = null;
}
// Constructs this node with the specified numerical data value, references to the next
// and previous nodes.
public Node(double initialData, Node nextNode, Node previousNode) {
data = initialData;
next = nextNode;
previous = previousNode;
}
// Returns this node's data.
public double getData() {
return data;
}
// Sets this node's data.
public void setData(double newData) {
data = newData;
}
// Gets the reference to the next node.
public Node getNext() {
return next;
}
// Sets the reference to the next node.
public void setNext(Node newNext) {
next = newNext;
}
// Gets the reference to the previous node.
public Node getPrevious() {
return previous;
}
// Sets the reference to the previous node.
public void setPrevious(Node newPrevious) {
previous = newPrevious;
}
}
SortedNumberList.java code below (Needs editing)
public class SortedNumberList {
public Node head;
public Node tail;
public SortedNumberList() {
head = null;
tail = null;
}
// Optional: Add any desired private methods here
// Inserts the number into the list in the correct position such that the
// list remains sorted in ascending order.
public void insert(double number) {
// Your code here
}
// Removes the node with the specified number value from the list. Returns
// true if the node is found and removed, false otherwise.
public boolean remove(double number) {
// Your code here
return false;
}
}
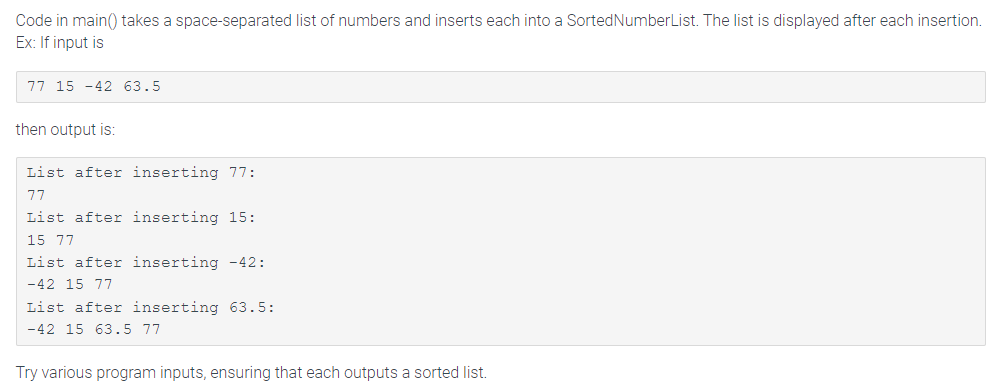

Step by step
Solved in 4 steps with 8 images

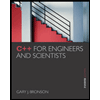
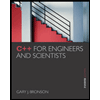