Can you help me how to do this code because I don't know what to do with this, this code has to be in python. I add the question and the functions that need to used. things that I need help with; the provided __init__ methods for the Atom, Not, And, Or, Implies, and Iff expression subclasses, keeping in mind that expressions will be considered immutable for our purposes. In particular, review the *args syntax and frozenset class if needed. The former allows for convenient n-ary conjunctions and disjunctions, and the latter is used to ensure immutability. We use sets rather than lists for conjunctions and disjunctions to guarantee that, e.g., all 24 permutations of the conjuncts in the expression A∧B∧C∧D will be equivalent. Moreover, you may find that certain set functions such as union and set difference will prove useful later on. As a first exercise, implement the __eq__(self, other) methods in each subclass, which will be called when expressions are compared using the == operator. You should check for syntactic equality only, in the sense that two expressions should be considered equal only if they are of the same class and have the same internal structure. No simplification should be performed. As a special case, Iff(a, b) should be equal to Iff(b, a). >>> Atom("a") == Atom("a") True >>> Atom("a") == Atom("b") False >>> And(Atom("a"), Not(Atom("b"))) == \ ... And(Not(Atom("b")), Atom("a")) True Implement the __repr__(self) method in each expression subclass, which should return a string representation of the given expression. Any reasonable choice will suffice for this exercise, as this is primarily intended for debugging purposes. >>> a, b, c = map(Atom, "abc") >>> Implies(a, Iff(b, c)) Implies(Atom(a), Iff(Atom(b), Atom(c))) >>> a, b, c = map(Atom, "abc") >>> And(a, Or(Not(b), c)) And(Atom(a), Or(Not(Atom(b)), Atom(c))) Implement the atom_names(self) method in each expression subclass, which should return the set of names that occur in atoms contained within the given expression. >>> Atom("a").atom_names() set(['a']) >>> Not(Atom("a")).atom_names() set(['a']) >>> a, b, c = map(Atom, "abc") >>> expr = And(a, Implies(b, Iff(a, c))) >>> expr.atom_names() set(['a', 'c', 'b'])
Can you help me how to do this code because I don't know what to do with this, this code has to be in python. I add the question and the functions that need to used.
things that I need help with;
the provided __init__ methods for the Atom, Not, And, Or, Implies, and Iff expression subclasses, keeping in mind that expressions will be considered immutable for our purposes. In particular, review the *args syntax and frozenset class
if needed. The former allows for convenient n-ary conjunctions and disjunctions, and the latter is used to ensure immutability.
We use sets rather than lists for conjunctions and disjunctions to guarantee that, e.g., all 24 permutations of the conjuncts in the expression A∧B∧C∧D will be equivalent. Moreover, you may find that certain set functions such as union and set difference will prove useful later on.
As a first exercise, implement the __eq__(self, other) methods in each subclass, which will be called when expressions are compared using the == operator. You should check for syntactic equality only, in the sense that two expressions should be considered equal only if they are of the same class and have the same internal structure. No simplification should be performed. As a special case, Iff(a, b) should be equal to Iff(b, a).
>>> Atom("a") == Atom("a")
True
>>> Atom("a") == Atom("b")
False
>>> And(Atom("a"), Not(Atom("b"))) == \
... And(Not(Atom("b")), Atom("a"))
True
Implement the __repr__(self) method in each expression subclass, which should return a string representation of the given expression. Any reasonable choice will suffice for this exercise, as this is primarily intended for debugging purposes.
>>> a, b, c = map(Atom, "abc")
>>> Implies(a, Iff(b, c))
Implies(Atom(a), Iff(Atom(b), Atom(c)))
>>> a, b, c = map(Atom, "abc")
>>> And(a, Or(Not(b), c))
And(Atom(a), Or(Not(Atom(b)), Atom(c)))
Implement the atom_names(self) method in each expression subclass, which should return the set of names that occur in atoms contained within the given expression.
>>> Atom("a").atom_names()
set(['a'])
>>> Not(Atom("a")).atom_names()
set(['a'])
>>> a, b, c = map(Atom, "abc")
>>> expr = And(a, Implies(b, Iff(a, c)))
>>> expr.atom_names()
set(['a', 'c', 'b'])


Step by step
Solved in 4 steps with 5 images

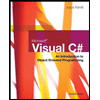
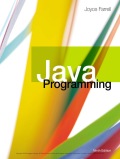
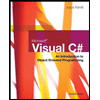
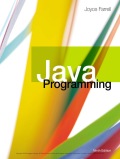