Can i get help with coding this in java. Only this question , you can even create your own AvlTree.java AvlTree.java is below public class AvlTree { private AvlNode root; public AvlTree() { root = null; } public void insert(Book book) { root = insert(book, root); } //Provided insert private AvlNode insert(Book book, AvlNode t) { if (t == null) { t = new AvlNode(book); } else if (book.getId() < t.getBook().getId()) { t.setLeft(insert(book, t.getLeft())); if (height(t.getLeft()) - height(t.getRight()) == 2) { if (book.getId() < t.getLeft().getBook().getId()) { t = rotLeft(t); } else { t = doubleRotLeft(t); } } } else if (book.getId() > t.getBook().getId()) { t.setRight(insert(book, t.getRight())); if (height(t.getRight()) - height(t.getLeft()) == 2) { if (book.getId() > t.getBook().getId()) { t = rotRight(t); } else { t = doubleRotRight(t); } } } else { //if both book ID's overlap do nothing and skip } t.setHeight(max(height(t.getLeft()), height(t.getRight())) + 1); return t; } public void printTree() { if (root == null) { System.out.println("Empty tree"); } else { printTree(root); } } private void printTree(AvlNode t) { if (t != null) { printTree(t.getLeft()); System.out.println(t.getBook().getId()); printTree(t.getRight()); } } private int height(AvlNode t) { return t == null ? -1 : t.getHeight(); } private int max(int lhs, int rhs) { return lhs > rhs ? lhs : rhs; } //For Question 1. private AvlNode rotLeft(AvlNode someNode) { return someNode; } //For Question 2 private AvlNode rotRight(AvlNode someNode) { return someNode; } here's AvlNode.java class AvlNode { private Book book; private int height; private AvlNode left; private AvlNode right; public AvlNode(Book book) { this.book = book; this.height = 1; this.left = null; this.right = null; } public Book getBook() { return book; } public int getHeight() { return height; } public AvlNode getLeft() { return left; } public AvlNode getRight() { return right; } public void setBook(Book book) { this.book = book; } public void setHeight(int height) { this.height = height; } public void setLeft(AvlNode left) { this.left = left; } public void setRight(AvlNode right) { this.right = right; } public AvlNode(Book book, int height, AvlNode left, AvlNode right) { this.book = book; this.height = height; this.left = left; this.right = right; } } Book.java public class Book { private String title; private int id; private String author; private double price; public Book() { this.title = ""; this.id = 0; this.author = ""; this.price = 0.0; } public Book(int id) { this.title = ""; this.id = id; this.author = ""; this.price = 0.0; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public Book(String title, int id, String author, double price) { this.title = title; this.id = id; this.author = author; this.price = price; } @Override public String toString() { return "Book object\n{" + "title= " + title + ", id= " + id + ", author= " + author + ", price= " + price + '}'; } }
Can i get help with coding this in java. Only this question , you can even create your own AvlTree.java
AvlTree.java is below
public class AvlTree {
private AvlNode root;
public AvlTree() {
root = null;
}
public void insert(Book book) {
root = insert(book, root);
}
//Provided insert
private AvlNode insert(Book book, AvlNode t) {
if (t == null) {
t = new AvlNode(book);
} else if (book.getId() < t.getBook().getId()) {
t.setLeft(insert(book, t.getLeft()));
if (height(t.getLeft()) - height(t.getRight()) == 2) {
if (book.getId() < t.getLeft().getBook().getId()) {
t = rotLeft(t);
} else {
t = doubleRotLeft(t);
}
}
} else if (book.getId() > t.getBook().getId()) {
t.setRight(insert(book, t.getRight()));
if (height(t.getRight()) - height(t.getLeft()) == 2) {
if (book.getId() > t.getBook().getId()) {
t = rotRight(t);
} else {
t = doubleRotRight(t);
}
}
} else {
//if both book ID's overlap do nothing and skip
}
t.setHeight(max(height(t.getLeft()), height(t.getRight())) + 1);
return t;
}
public void printTree() {
if (root == null) {
System.out.println("Empty tree");
} else {
printTree(root);
}
}
private void printTree(AvlNode t) {
if (t != null) {
printTree(t.getLeft());
System.out.println(t.getBook().getId());
printTree(t.getRight());
}
}
private int height(AvlNode t) {
return t == null ? -1 : t.getHeight();
}
private int max(int lhs, int rhs) {
return lhs > rhs ? lhs : rhs;
}
//For Question 1.
private AvlNode rotLeft(AvlNode someNode) {
return someNode;
}
//For Question 2
private AvlNode rotRight(AvlNode someNode) {
return someNode;
}
here's AvlNode.java
class AvlNode {
private Book book;
private int height;
private AvlNode left;
private AvlNode right;
public AvlNode(Book book) {
this.book = book;
this.height = 1;
this.left = null;
this.right = null;
}
public Book getBook() {
return book;
}
public int getHeight() {
return height;
}
public AvlNode getLeft() {
return left;
}
public AvlNode getRight() {
return right;
}
public void setBook(Book book) {
this.book = book;
}
public void setHeight(int height) {
this.height = height;
}
public void setLeft(AvlNode left) {
this.left = left;
}
public void setRight(AvlNode right) {
this.right = right;
}
public AvlNode(Book book, int height, AvlNode left, AvlNode right) {
this.book = book;
this.height = height;
this.left = left;
this.right = right;
}
}
Book.java
public class Book {
private String title;
private int id;
private String author;
private double price;
public Book() {
this.title = "";
this.id = 0;
this.author = "";
this.price = 0.0;
}
public Book(int id) {
this.title = "";
this.id = id;
this.author = "";
this.price = 0.0;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public Book(String title, int id, String author, double price) {
this.title = title;
this.id = id;
this.author = author;
this.price = price;
}
@Override
public String toString() {
return "Book object\n{" + "title= " + title + ", id= " + id + ", author= " + author + ", price= " + price + '}';
}
}


Step by step
Solved in 2 steps

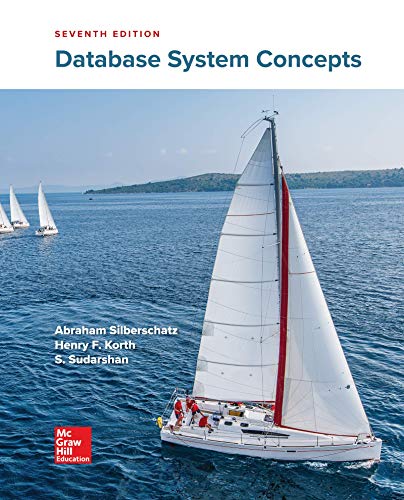
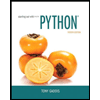
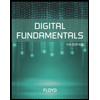
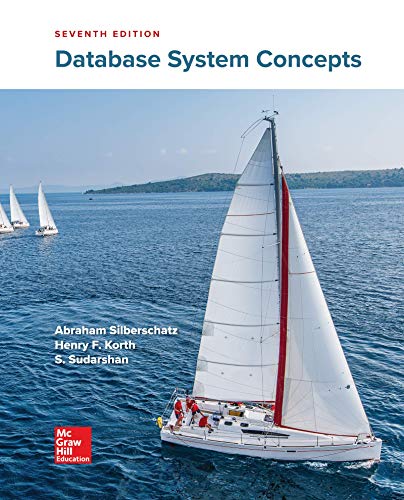
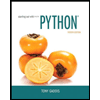
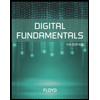
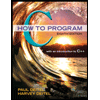
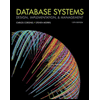
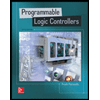