C programming: Use following code and modify in the end to get median. Sorting is not needed as it already sorted. #include #define MAX_SCORE 20 #define MAX_SIZE 1000 int main() { int i = 0 ; // used as index into array int n = 0 ; // number of items in array int r ; // used to ensure user enters an integer double average ; // average of numbers entered by user double num ; // total number of items in array int A[MAX_SIZE] ; // will hold the scores n = 0 ; i = 0 ; // read in the scores into array A[] while (1) { r = scanf("%d", &A[i] ) ; // end of input? if ( r <= 0 ) { break ; } i = i + 1 ; // exceeded array size? if (i >= MAX_SIZE) { break ; } } // Make n the number of items stored in A[] n = i ; // compute the average int sum = 0 ; for (i = 0 ; i < n ; i++) { sum = sum + A[i] ; } num = n ; // num is double average = sum / num ; printf ("The average score is: %f\n", average) ; // ***** // ***** TODO // ***** // Declare a new count[] array. int count[MAX_SCORE + 1] ; // Initialize each element of count[] to 0 using a for loop. for(i = 0; i < (MAX_SCORE + 1); i++) { count[i]=0; } // Iterate through the elements of the A[] array and update count[] in // each iteration. for(i = 0; i < n; i++) { count[A[i]]++; } // // Iterate through the count[] array and print out the number of times // that each score appeared. for(i = 0; i < (MAX_SCORE + 1); i++) { printf("count[%d] is %d. \n",i,count[i]); } // Iterate through the elements of the count[] array to find the maximum // count and the score with the maximum count. int mode = 0; for(i = 0; i < (MAX_SCORE + 1); i++) { if(count[i] > count[mode]) { mode = i; } } // Print out the score that has the highest count and the number of // times that score appeared. printf("The mode of the scores is %d. It occurred %d times.\n",mode,count[mode]); return 0 ; } Example Compilation and Execution: The number of scores is 21. The average score is: 13.047619 count[0] is 0. count[1] is 1. count[2] is 2. count[3] is 0. count[4] is 0. count[5] is 0. count[6] is 0. count[7] is 1. count[8] is 1. count[9] is 1. count[10] is 0. count[11] is 1. count[12] is 2. count[13] is 1. count[14] is 0. count[15] is 1. count[16] is 2. count[17] is 2. count[18] is 1. count[19] is 2. count[20] is 3. The mode of the scores is 20. It occurred 3 times. The median score is 15. Notes: Think about how to calculate the median. You already have the count[ ] array computed. If you iterate through the count[ ] array, you should be able to determine when half of the scores (n/2 when n is even and (n+1)/2 when n is odd) have been encountered. Then you have the median. Do this by hand on a small example first. NOTE: There is no need to modify the A array to find the median. Do NOT modify it!
C programming:
Use following code and modify in the end to get median. Sorting is not needed as it already sorted.
#include <stdio.h>
#define MAX_SCORE 20
#define MAX_SIZE 1000
int main() {
int i = 0 ; // used as index into array
int n = 0 ; // number of items in array
int r ; // used to ensure user enters an integer
double average ; // average of numbers entered by user
double num ; // total number of items in array
int A[MAX_SIZE] ; // will hold the scores
n = 0 ;
i = 0 ;
// read in the scores into array A[]
while (1) {
r = scanf("%d", &A[i] ) ;
// end of input?
if ( r <= 0 ) {
break ;
}
i = i + 1 ;
// exceeded array size?
if (i >= MAX_SIZE) {
break ;
}
}
// Make n the number of items stored in A[]
n = i ;
// compute the average
int sum = 0 ;
for (i = 0 ; i < n ; i++) {
sum = sum + A[i] ;
}
num = n ; // num is double
average = sum / num ;
printf ("The average score is: %f\n", average) ;
// *****
// ***** TODO
// *****
// Declare a new count[] array.
int count[MAX_SCORE + 1] ;
// Initialize each element of count[] to 0 using a for loop.
for(i = 0; i < (MAX_SCORE + 1); i++) {
count[i]=0;
}
// Iterate through the elements of the A[] array and update count[] in
// each iteration.
for(i = 0; i < n; i++) {
count[A[i]]++;
}
//
// Iterate through the count[] array and print out the number of times
// that each score appeared.
for(i = 0; i < (MAX_SCORE + 1); i++) {
printf("count[%d] is %d. \n",i,count[i]);
}
// Iterate through the elements of the count[] array to find the maximum
// count and the score with the maximum count.
int mode = 0;
for(i = 0; i < (MAX_SCORE + 1); i++) {
if(count[i] > count[mode]) {
mode = i;
}
}
// Print out the score that has the highest count and the number of
// times that score appeared.
printf("The mode of the scores is %d. It occurred %d times.\n",mode,count[mode]);
return 0 ;
}
Example Compilation and Execution:
The number of scores is 21.
The average score is: 13.047619
count[0] is 0.
count[1] is 1.
count[2] is 2.
count[3] is 0.
count[4] is 0.
count[5] is 0.
count[6] is 0.
count[7] is 1.
count[8] is 1.
count[9] is 1.
count[10] is 0.
count[11] is 1.
count[12] is 2.
count[13] is 1.
count[14] is 0.
count[15] is 1.
count[16] is 2.
count[17] is 2.
count[18] is 1.
count[19] is 2.
count[20] is 3.
The mode of the scores is 20. It occurred 3 times.
The median score is 15.
Notes:
Think about how to calculate the median. You already have the count[ ] array computed.
If you iterate through the count[ ] array, you should be able to determine when half of the scores (n/2 when n is even and (n+1)/2 when n is odd) have been encountered. Then you have the median. Do this by hand on a small example first.
NOTE: There is no need to modify the A array to find the median. Do NOT modify it!

Step by step
Solved in 3 steps with 4 images

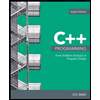
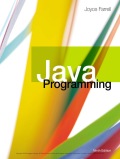
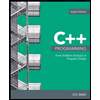
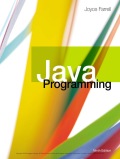