C++ I have a code, but it doesn't work well. Please help me fix it. The code read 2 file, Punchcards.txt as a key (for password) and Tuple.csv as the password. Punchcards.txt -------------------------------------------------------------------------------- Y 00000000000000000000000000000000000000000000000000000000000000000000000000000000 X 00000000000000000000000000000000000000000000000000000000000000000000000000000000 0 10000000000000000000000000000000000000000000000000000000000000000000000000000000 1 01000000000000000000000000000000000000000000000000000000000000000000000000000000 2 00100000000000000000000000000000000000000000000000000000000000000000000000000000 3 00010000000000000000000000000000000000000000000000000000000000000000000000000000 4 00001000000000000000000000000000000000000000000000000000000000000000000000000000 5 00000100000000000000000000000000000000000000000000000000000000000000000000000000 6 00000010000000000000000000000000000000000000000000000000000000000000000000000000 7 00000001000000000000000000000000000000000000000000000000000000000000000000000000 8 00000000100000000000000000000000000000000000000000000000000000000000000000000000 9 00000000010000000000000000000000000000000000000000000000000000000000000000000000 -------------------------------------------------------------------------------- Tuple.csv Y,0,& 0,2,0 1,3,1 2,4,2 3,5,3 4,6,4 5,7,5 6,8,6 7,9,7 8,A,8 9,B,9 Y1,03,A Y2,04,B Y3,05,C Y4,06,D Y5,07,E Y6,08,F Y7,09,G Y8,0A,H Y9,0B,I X1,13,J X2,14,K X3,15,L X4,16,M X5,17,N X6,18,O X7,19,P X8,1A,Q X9,1B,R 01,23,/ 02,24,S 03,25,T 04,26,U 05,27,V 06,28,W 07,29,X 08,2A,Y 09,2B,Z 82,A4,: 83,A5,# 84,A6,@ 85,A7,' 82,A8,= 86,A9,\ 082,A8,= Y82,0A4,¢ Y83,0A5,. Y84,0A6,< Y85,0A7,( Y86,0A8,+ Y87,0A9,| X82,1A4,! X83,1A5,$ X84,1A6,* X85,1A7, X86,1A8,; X87,1A9,¬ 083,2A5,, 084,2A6,% 085,2A7,_ 086,2A8,> 087,2A9,? Mycode.cpp // Y Nhi Tran // Lab 0 - Devcryption #include #include #include #include #include #include #include #include using namespace std; class Decryption { public: virtual string Process(vector> matrix) { } char Translate(string ebdic) { auto it = find_if(tupleVector.begin(), tupleVector.end(), [&ebdic](const auto& t){ return get<1>(t) == ebdic; }); if (it != tupleVector.end()) { return get<2>(*it); } else { return '?'; } } void DecryptFile(string filename) { ifstream inFile(filename); string line; vector decryptedText; while (getline(inFile, line)) { if (line.substr(0, 1) == "-") { continue; } vector> matrix(12, vector(80)); for (int i = 0; i < 12; i++) { getline(inFile, line); for (int j = 0; j < 80; j++) { matrix[i][j] = line[j]; } } decryptedText.push_back(Process(matrix)); } translate = decryptedText; } vector GetDecryptedText() { return translate; } protected: vector> tupleVector; vector translate; }; // Define a struct to represent a tuple template struct tuple { T1 field1; T2 field2; T3 field3; }; // Define a regex callback function for parsing binary files string RemoveSpace(string s) { regex nonSpace(" "); return regex_replace(s, nonSpace, ""); } int main() { // Read the tuple file ifstream tupleFile("Tuple.csv"); string line; vector> tupleVector; while (getline(tupleFile, line)) { // Split the line into three parts separated by commas size_t comma1 = line.find(","); size_t comma2 = line.find(",", comma1+1); string field1 = line.substr(0, comma1); string field2 = line.substr(comma1+1, comma2-comma1-1); char field3 = line[comma2+1]; // Create a tuple from the three fields and add it to the vector tupleVector.push_back(tuple{field1, field2, field3}); } // Read the punch card file ifstream punchCardFile("PunchCards.txt"); vector translate; while (getline(punchCardFile, line)) { // Skip any lines that start with dashes if (line.substr(0, 1) == "-") { continue; } // Read the next 12 lines and store them in a matrix vector> matrix(12, vector(80)); for (int i = 0; i < 12; i++) { getline(punchCardFile, line); for (int j = 0; j < 80; j++) { matrix[i][j] = line[j]; } } // Process the matrix by looking up each EBDIC character in the tuple vector string translated; for (int j = 0; j < 80; j++) { string ebdic; for (int i = 0; i < 12; i++) { ebdic += matrix[i][j]; } auto it = find_if(tupleVector.begin(), tupleVector.end(), [&ebdic](const auto& t){ return get<1>(t) == ebdic; }); if (it != tupleVector.end()) { translated += get<2>(*it); } } translate.push_back(translated); } }
C++
I have a code, but it doesn't work well. Please help me fix it.
The code read 2 file, Punchcards.txt as a key (for password) and Tuple.csv as the password.
Punchcards.txt
--------------------------------------------------------------------------------
Y 00000000000000000000000000000000000000000000000000000000000000000000000000000000
X 00000000000000000000000000000000000000000000000000000000000000000000000000000000
0 10000000000000000000000000000000000000000000000000000000000000000000000000000000
1 01000000000000000000000000000000000000000000000000000000000000000000000000000000
2 00100000000000000000000000000000000000000000000000000000000000000000000000000000
3 00010000000000000000000000000000000000000000000000000000000000000000000000000000
4 00001000000000000000000000000000000000000000000000000000000000000000000000000000
5 00000100000000000000000000000000000000000000000000000000000000000000000000000000
6 00000010000000000000000000000000000000000000000000000000000000000000000000000000
7 00000001000000000000000000000000000000000000000000000000000000000000000000000000
8 00000000100000000000000000000000000000000000000000000000000000000000000000000000
9 00000000010000000000000000000000000000000000000000000000000000000000000000000000
--------------------------------------------------------------------------------
Tuple.csv
Y,0,&
0,2,0
1,3,1
2,4,2
3,5,3
4,6,4
5,7,5
6,8,6
7,9,7
8,A,8
9,B,9
Y1,03,A
Y2,04,B
Y3,05,C
Y4,06,D
Y5,07,E
Y6,08,F
Y7,09,G
Y8,0A,H
Y9,0B,I
X1,13,J
X2,14,K
X3,15,L
X4,16,M
X5,17,N
X6,18,O
X7,19,P
X8,1A,Q
X9,1B,R
01,23,/
02,24,S
03,25,T
04,26,U
05,27,V
06,28,W
07,29,X
08,2A,Y
09,2B,Z
82,A4,:
83,A5,#
84,A6,@
85,A7,'
82,A8,=
86,A9,\
082,A8,=
Y82,0A4,¢
Y83,0A5,.
Y84,0A6,<
Y85,0A7,(
Y86,0A8,+
Y87,0A9,|
X82,1A4,!
X83,1A5,$
X84,1A6,*
X85,1A7,
X86,1A8,;
X87,1A9,¬
083,2A5,,
084,2A6,%
085,2A7,_
086,2A8,>
087,2A9,?
Mycode.cpp
// Y Nhi Tran
// Lab 0 - Devcryption
#include <iostream>
#include <fstream>
#include <string>
#include <functional>
#include <
#include <tuple>
#include <regex>
#include <algorithm>
using namespace std;
class Decryption {
public:
virtual string Process(vector<vector<char>> matrix) {
}
char Translate(string ebdic) {
auto it = find_if(tupleVector.begin(), tupleVector.end(), [&ebdic](const auto& t){ return get<1>(t) == ebdic; });
if (it != tupleVector.end()) {
return get<2>(*it);
} else {
return '?';
}
}
void DecryptFile(string filename) {
ifstream inFile(filename);
string line;
vector<string> decryptedText;
while (getline(inFile, line)) {
if (line.substr(0, 1) == "-") {
continue;
}
vector<vector<char>> matrix(12, vector<char>(80));
for (int i = 0; i < 12; i++) {
getline(inFile, line);
for (int j = 0; j < 80; j++) {
matrix[i][j] = line[j];
}
}
decryptedText.push_back(Process(matrix));
}
translate = decryptedText;
}
vector<string> GetDecryptedText() {
return translate;
}
protected:
vector<tuple<string, string, char>> tupleVector;
vector<string> translate;
};
// Define a struct to represent a tuple
template <typename T1, typename T2, typename T3>
struct tuple {
T1 field1;
T2 field2;
T3 field3;
};
// Define a regex callback function for parsing binary files
string RemoveSpace(string s) {
regex nonSpace(" ");
return regex_replace(s, nonSpace, "");
}
int main() {
// Read the tuple file
ifstream tupleFile("Tuple.csv");
string line;
vector<tuple<string, string, char>> tupleVector;
while (getline(tupleFile, line)) {
// Split the line into three parts separated by commas
size_t comma1 = line.find(",");
size_t comma2 = line.find(",", comma1+1);
string field1 = line.substr(0, comma1);
string field2 = line.substr(comma1+1, comma2-comma1-1);
char field3 = line[comma2+1];
// Create a tuple from the three fields and add it to the vector
tupleVector.push_back(tuple<string, string, char>{field1, field2, field3});
}
// Read the punch card file
ifstream punchCardFile("PunchCards.txt");
vector<string> translate;
while (getline(punchCardFile, line)) {
// Skip any lines that start with dashes
if (line.substr(0, 1) == "-") {
continue;
}
// Read the next 12 lines and store them in a matrix
vector<vector<char>> matrix(12, vector<char>(80));
for (int i = 0; i < 12; i++) {
getline(punchCardFile, line);
for (int j = 0; j < 80; j++) {
matrix[i][j] = line[j];
}
}
// Process the matrix by looking up each EBDIC character in the tuple vector
string translated;
for (int j = 0; j < 80; j++) {
string ebdic;
for (int i = 0; i < 12; i++) {
ebdic += matrix[i][j];
}
auto it = find_if(tupleVector.begin(), tupleVector.end(), [&ebdic](const auto& t){ return get<1>(t) == ebdic; });
if (it != tupleVector.end()) {
translated += get<2>(*it);
}
}
translate.push_back(translated);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 7 steps

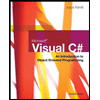
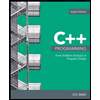
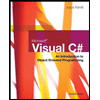
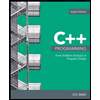