BreakCheck class import java.util.*; public class BreakCheck implements Check { public Optional lint(String line, int lineNumber) { // Match "break" outside of comments or strings if (line.matches("^(?!\\s*//|\\s*/\\*|\\s*\\*).*\\bbreak\\b.*")) { return Optional.of(new Error(2, lineNumber, "Line contains 'break' keyword outside of a comment")); } return Optional.empty(); } }
I need help with this Java program. I got some minor error that I couldn't fix.
Checker Classes
You will have to implement specific checks to highlight errors found in the source files. We provided an interface Check.java that defines a single method public Optional<Error> lint(String line, int lineNumber) . All the checkers you write should implement this interface and hence, you need to implement the lint method.
All of these should return an an Error when one is present with a custom message of your choosing to describe what the error means to the user. If the condition a Check is looking for is not present for a line, should return Optional.empty().
Other class
Error.java
public Error(int code, int lineNumber, String message)
Constructs an Error given the error code, line number and a message.
public String toString()
Returns a String representation of the error with the line number, error code and message. The representation should be formatted as (replace curly braces with the actual values)
public int getLineNumber()
Returns the line number of this error
public int getCode()
Returns the error code of this error
public String getMessage()
Returns the message for this error
Linter.java
public Linter(List<Check> checks)
Constructs a Linter given a list of checks to run through.
public List<Error> lint(String fileName) throws FileNotFoundException
Returns a list of all errors found.
All of these should return an an Error when one is present with a custom message of your choosing to describe what the error means to the user. If the condition a Check is looking for is not present for a line, should return Optional.empty().
BreakCheck class
import java.util.*;
public class BreakCheck implements Check {
public Optional<Error> lint(String line, int lineNumber) {
// Match "break" outside of comments or strings
if (line.matches("^(?!\\s*//|\\s*/\\*|\\s*\\*).*\\bbreak\\b.*")) {
return Optional.of(new Error(2, lineNumber, "Line contains 'break' keyword outside of a comment"));
}
return Optional.empty();
}
}
Error Class:
public class Error {
private final int code;
private final int lineNumber;
private final String message;
public Error(int code, int lineNumber, String message) {
this.code = code;
this.lineNumber = lineNumber;
this.message = message;
}
public String toString() {
return String.format("(Line: %d) has error code %d\n%s", lineNumber, code, message);
}
public int getLineNumber() {
return lineNumber;
}
public int getCode() {
return code;
}
public String getMessage() {
return message;
}
}
TestFile:
public class TestFile {
public static void main(String[] args) {
System.out.println("This is a really really really long line that should fail the long line check");
while (true) {
System.out.println("");
break;
}
}
}
Linter Class
import java.util.*;
import java.io.*;
public class Linter {
private final List<Check> checks;
public Linter(List<Check> checks) {
this.checks = checks;
}
public List<Error> lint(String fileName) throws FileNotFoundException {
List<Error> errors = new ArrayList<>();
File file = new File(fileName);
Scanner scanner = new Scanner(file);
int lineNumber = 0;
while (scanner.hasNextLine()) {
lineNumber++;
String line = scanner.nextLine();
for (Check check : checks) {
Optional<Error> result = check.lint(line, lineNumber);
if (result.isPresent()) {
errors.add(result.get());
}
}
}
scanner.close();
return errors;
}
}
LinterMain :
import java.util.*;
import java.io.*;
public class LinterMain {
public static final String FILE_NAME = "TestFile.java";
public static void main(String[] args) throws FileNotFoundException{
List<Check> checks = new ArrayList<>();
checks.add(new LongLineCheck());
checks.add(new BlankPrintlnCheck());
checks.add(new BreakCheck());
Linter linter = new Linter(checks);
List<Error> errors = linter.lint(FILE_NAME);
for (Error e : errors) {
System.out.println(e);
}
}
}
Check.java
import java.util.*;public interface Check {
public Optional<Error> lint(String line, int lineNumber);
}
I can't pass this check :
BreakCheck correctly tests for break statements:
Failed: Make sure that breakCheck does not only check if a line starts with a comment for the case when 'break' is in a comment. ==> expected: <true> but was: <false>



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

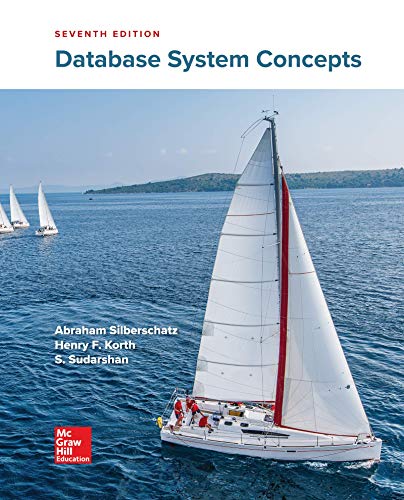
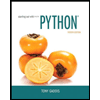
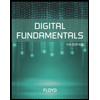
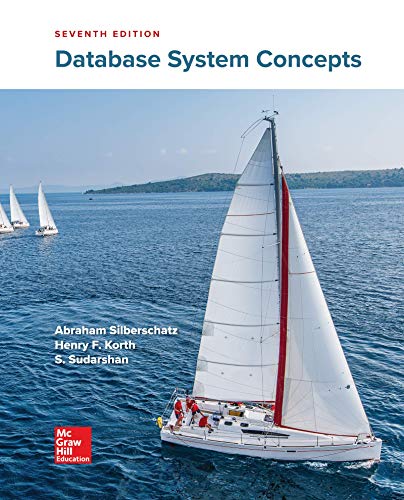
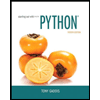
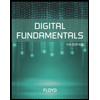
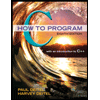
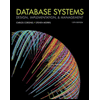
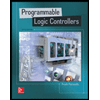