Binary Expression Tree A binary expression tree is a specific kind of a binary tree used to represent expressions. The leaves of the binary expression tree are operands, and the interior nodes contain operators. Assume the possible operators including +, -,,, and % and operands are numerical data. The following figures illustrate binary expression trees for the expressions with different notations: Example 1 Postfix Exp: 14-5/ BXT: [/] [14] [-5] Infix order: 14 / -5 Prefix order: / 14-5 Evaluates to -2.8 Example 2 Postfix Exp: 203-4+* Example 3 Postfix Exp: 23+5/45-* BXT: BXT: [[*] [20] [+] [/1 [-] /\ , . ハ [3] [-4] [+] [5] [4] [5] 1 Infix order: 203-4 Prefix order: 20 + 3-4 Evaluates to -20.0 [2] [3] Infix order: 2 + 3 / 54-5 Prefix order: * / +235-45 Evaluates to -1.0 Your Tasks: For this assignment, you will build a binary expression tree, display it, and evaluate it. You will encapsulate the behavior in a BXT class. The driver class, tree node, and the BXT class are provided. Please implement appropriate methods in BXT class to build, display, and evaluate a tree. Requirements for each method: Build a BXT: You need to change the string into a tree. The argument string is in postfix notation. Display Infix and Prefix orders Infix is characterized by the placement of operators between operands; Prefix expression notation requires that all operators precede the two operands that they work on; Postfix requires that its operators come after the corresponding operands. See following examples: Infix, Prefix, and Postfix Orders Infix Expression Prefix Expression Postfix Expression A+B +AB AB+ A+B˚C + A*BC ABC*+ Evaluating the Expression Do this recursively. If the node is an operator, recursively evaluate the left child and the right child, and return the result. Else the node is a number, so it can be converted into a double, and returned. Requirements for your application: Please design an application to meet the following specific methods: . build Tree(String str): The argument string is in postfix notation. Build the tree as specified in the document-refer to examples 1,2 and 3; • eveluate Tree(): Do this recursively. If the node is an operator, recursively evaluate the left child and the right child, and return the result. Else the node is a number, so it can be converted into a double, and returned. • infix(): Infix is characterized by the placement of operators between operands; prefix(): Prefix expression notation requires that all operators precede the two operands that they work on; posfix(): Postfix requires that its operators come after the corresponding operands
I need help with this Java problem to output as it's explained in the image below:
NodeInterface.java (Don't edit)
interface NodeInterface<T>{
T getData();
NodeInterface<T> getLeft();
NodeInterface<T> getRight();
}
/**
*
* TreeNode.java: TreeNode class. // (Don't edit)
*/
public class TreeNode implements NodeInterface<String>
{
private String value;
private TreeNode left, right;
public TreeNode(String initValue)
{
value = initValue;
left = null;
right = null;
}
public TreeNode(String initValue, TreeNode initLeft, TreeNode initRight)
{
value = initValue;
left = initLeft;
right = initRight;
}
public String getData()
{
return value;
}
public TreeNode getLeft()
{
return left;
}
public TreeNode getRight()
{
return right;
}
public void setValue(String theNewValue)
{
value = theNewValue;
}
public void setLeft(TreeNode theNewLeft)
{
left = theNewLeft;
}
public void setRight(TreeNode theNewRight)
{
right = theNewRight;
}
}
![Binary Expression Tree
A binary expression tree is a specific kind of a binary tree used to represent expressions. The leaves of the binary expression tree are
operands, and the interior nodes contain operators. Assume the possible operators including +, -,,, and % and operands are numerical
data. The following figures illustrate binary expression trees for the expressions with different notations:
Example 1
Postfix Exp: 14-5/
BXT:
[/]
[14] [-5]
Infix order: 14 / -5
Prefix order: / 14-5
Evaluates to -2.8
Example 2
Postfix Exp: 203-4+*
Example 3
Postfix Exp: 23+5/45-*
BXT:
BXT:
[[*]
[20]
[+]
[/1
[-]
/\
,
.
ハ
[3]
[-4]
[+]
[5] [4]
[5]
1
Infix order: 203-4
Prefix order: 20 + 3-4
Evaluates to -20.0
[2]
[3]
Infix order: 2 + 3 / 54-5
Prefix order: * / +235-45
Evaluates to -1.0
Your Tasks:
For this assignment, you will build a binary expression tree, display it, and evaluate it. You will encapsulate the behavior in a BXT class. The
driver class, tree node, and the BXT class are provided. Please implement appropriate methods in BXT class to build, display, and evaluate a
tree.
Requirements for each method:
Build a BXT: You need to change the string into a tree. The argument string is in postfix notation.
Display Infix and Prefix orders
Infix is characterized by the placement of operators between operands;
Prefix expression notation requires that all operators precede the two operands that they work on;
Postfix requires that its operators come after the corresponding operands. See following examples:
Infix, Prefix, and Postfix Orders
Infix Expression Prefix Expression Postfix Expression
A+B
+AB
AB+
A+B˚C
+ A*BC
ABC*+
Evaluating the Expression
Do this recursively. If the node is an operator, recursively evaluate the left child and the right child, and return the result. Else the node is a
number, so it can be converted into a double, and returned.
Requirements for your application:
Please design an application to meet the following specific methods:
.
build Tree(String str): The argument string is in postfix notation. Build the tree as specified in the document-refer to examples 1,2 and
3;
• eveluate Tree(): Do this recursively. If the node is an operator, recursively evaluate the left child and the right child, and return the result.
Else the node is a number, so it can be converted into a double, and returned.
• infix(): Infix is characterized by the placement of operators between operands;
prefix(): Prefix expression notation requires that all operators precede the two operands that they work on;
posfix(): Postfix requires that its operators come after the corresponding operands](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e135d5c-f867-4afd-9f0d-b505f4f19664%2F3ffc3ae3-6f3b-43c1-b73e-263dda7ba806%2F8hsuz1_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

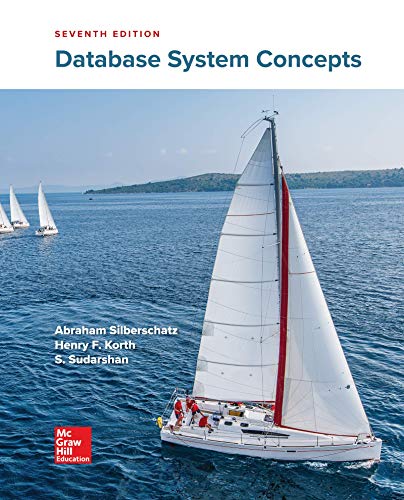
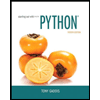
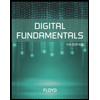
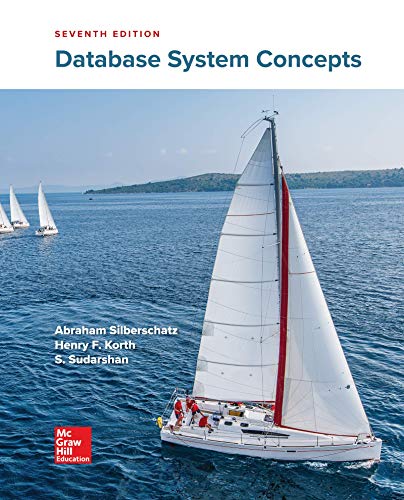
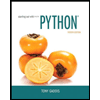
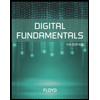
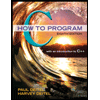
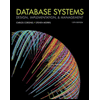
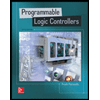