"ALBUM by ARTIST". Finally, computers should be "Public Computer". You may find the OCaML function string_of_int: int -> string helpful. Example Outputs # string_of_item (Book ("Types and Programming Languages", "Benjamin Pierce"));; -: string = "Types and Programming Languages by Benjamin Pierce" # string_of_item (Movie ("The Imitation Game", 2014)) ;; string= "The Imitation Game (2014)" # string_of_item Computer;; - string = "Public Computer" 2.2 string_of_entry checkout_entry -> string The second function you must implement converts checkout_entry to a string. An normal Item shall be rendered just as the wrapped catalog_item would be. A new entry should be rendered as "(NEW) ENTRY", where ENTRY is the wrapped checkout_entry. A pair should be "ENTRY1 and ENTRY2", where ENTRY1 and ENTRY2 are the items. In implementing this function, you may wish to call the string_of_item function you previously wrote. Example Outputs # string_of_entry (Item (Book ("Types and Programming Languages", "Benjamin Pierce"))) ;; - string = "Types and Programming Languages by Benjamin Pierce" #string_of_entry (New (Item (Movie ("The Imitation Game", 2014)))) ;; -: string = "(NEW) The Imitation Game (2014)" 2.3 daily_fine checkout_entry -> float The third function you must implement computes the fines (in dollars) incurred for each day an item is overdue. The fines are as follows: • Books: 0.25 • Movie: 0.50 • CD: 0.50 • Computer: 0.00 • New Items: Double the normal fine • Pair: The sum of the fines for each item 2.4 total fine: checkout list -> float The fourth function you will implement takes a list of checkout and returns the total fine for all items. Remember, if the int option is None, the item is not overdue and thus no fines are due for that item. You may find float_of_int : int -> float helpful in implementing this function.
"ALBUM by ARTIST". Finally, computers should be "Public Computer". You may find the OCaML function string_of_int: int -> string helpful. Example Outputs # string_of_item (Book ("Types and Programming Languages", "Benjamin Pierce"));; -: string = "Types and Programming Languages by Benjamin Pierce" # string_of_item (Movie ("The Imitation Game", 2014)) ;; string= "The Imitation Game (2014)" # string_of_item Computer;; - string = "Public Computer" 2.2 string_of_entry checkout_entry -> string The second function you must implement converts checkout_entry to a string. An normal Item shall be rendered just as the wrapped catalog_item would be. A new entry should be rendered as "(NEW) ENTRY", where ENTRY is the wrapped checkout_entry. A pair should be "ENTRY1 and ENTRY2", where ENTRY1 and ENTRY2 are the items. In implementing this function, you may wish to call the string_of_item function you previously wrote. Example Outputs # string_of_entry (Item (Book ("Types and Programming Languages", "Benjamin Pierce"))) ;; - string = "Types and Programming Languages by Benjamin Pierce" #string_of_entry (New (Item (Movie ("The Imitation Game", 2014)))) ;; -: string = "(NEW) The Imitation Game (2014)" 2.3 daily_fine checkout_entry -> float The third function you must implement computes the fines (in dollars) incurred for each day an item is overdue. The fines are as follows: • Books: 0.25 • Movie: 0.50 • CD: 0.50 • Computer: 0.00 • New Items: Double the normal fine • Pair: The sum of the fines for each item 2.4 total fine: checkout list -> float The fourth function you will implement takes a list of checkout and returns the total fine for all items. Remember, if the int option is None, the item is not overdue and thus no fines are due for that item. You may find float_of_int : int -> float helpful in implementing this function.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
OCaml Code: Make sure to show the full code and take a screenshot of the output. Make sure to use various test cases as well. There must be no error in the code at all. Make sure the code prints out all of the example outputs that is circled in the image attached.

Transcribed Image Text:"ALBUM by ARTIST". Finally, computers should be "Public Computer". You may find the
OCaML function string_of_int: int -> string helpful.
Example Outputs
# string_of_item (Book ("Types and Programming Languages", "Benjamin Pierce")) ;;
- : string = "Types and Programming Languages by Benjamin Pierce"
# string_of_item (Movie ("The Imitation Game", 2014)) ;;
-: string = "The Imitation Game (2014)"
#string_of_item Computer;;
string = "Public Computer"
2.2 string_of_entry checkout_entry -> string
The second function you must implement converts checkout_entry to a string. An normal Item
shall be rendered just as the wrapped catalog_item would be. A new entry should be rendered as
"(NEW) ENTRY", where ENTRY is the wrapped checkout_entry. A pair should be "ENTRY1
and ENTRY2", where ENTRY1 and ENTRY2 are the items. In implementing this function, you
may wish to call the string_of_item function you previously wrote.
Example Outputs
#string_of_entry (Item (Book ("Types and Programming Languages", "Benjamin Pierce"))) ;;
- string = "Types and Programming Languages by Benjamin Pierce"
# string_of_entry (New (Item (Movie ("The Imitation Game", 2014)))) ;;
- string "(NEW) The Imitation Game (2014)"
2.3 daily_fine: checkout_entry -> float
The third function you must implement computes the fines (in dollars) incurred for each day an
item is overdue. The fines are as follows:
• Books: 0.25
• Movie: 0.50
• CD: 0.50
• Computer: 0.00
• New Items: Double the normal fine
• Pair: The sum of the fines for each item
2.4 total fine checkout list -> float
The fourth function you will implement takes a list of checkout and returns the total fine for all
items. Remember, if the int option is None, the item is not overdue and thus no fines are due for
that item.
You may find float_of_int: int -> float helpful in implementing this function.

Transcribed Image Text:In this assignment you are asked to implement portions of the checkout system backend for the
Oakland, California Municipal Library (OCaML). You will be writing functions to handle calcu-
lating fines for overdue items. Your implementations must adhere to a specified behavior and type
(or signature). These functions will operate on a set of three nested data types representing the
library's records.
The data types representing records are defined as follows:
type catalog_item = Book of string * string | Movie of string * int |
CD of string * string | Computer
type checkout_entry = Item of catalog_item | New of checkout_entry |
Pair of checkout_entry * checkout_entry
type checkout Checkout of checkout_entry * int option
These definitions are also provided in the file library.ml which you can use as a basis for your
development.
Note that constructor names must begin with a capital letter, and binding names must begin
with a lowercase letter (or an underscore).
Here a catalog_item represents one physical item in the library. For a book, the parameters
are the title and author. For a movie, the parameters are title and year. For a CD, the parameters
are the album name and artist. Computer represents using a public computer, and thus has no
arguments.
Some items are new or can only be checked out as a pair with another item. A checkout_entry
represents one checked out bundle. Finally, a checkout combines a checkout_entry with an integer
option representing the number of days overdue. If the option is None, the item is not overdue. If
the option is Some n, n is the number of days the item is overdue. You may assume n is always
positive.
2 Function specifications
2.1 string_of_item : catalog_item -> string
The first function you will implement converts a catalog_item to a string. Books shall be rendered
as "TITLE by AUTHOR". A movie shall be "TITLE (YEAR)". A CD shall be rendered as
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
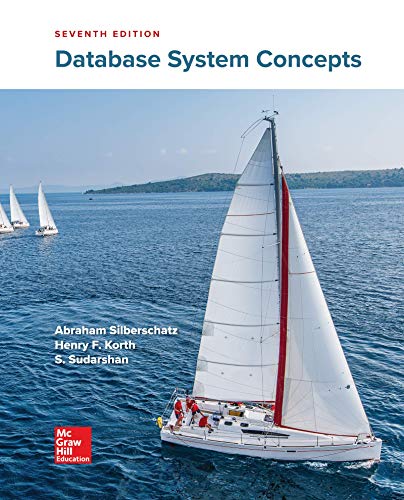
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
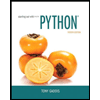
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
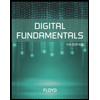
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
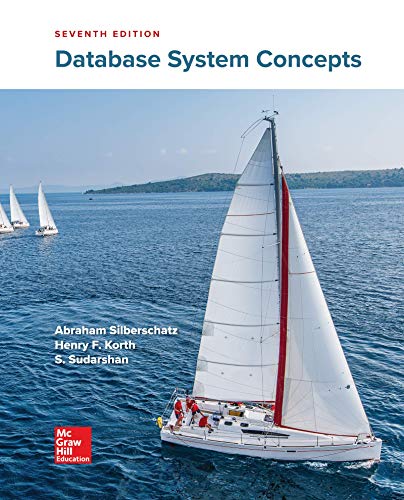
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
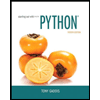
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
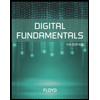
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
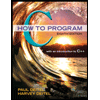
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
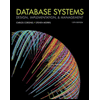
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
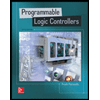
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education