Add a findAndRemove() function which is able the remove the cards from the original hand that the user selected to be thrown away. Inside the play() function add the logic to draw replacement cards from the SAME deck. The number of cards drawn from the deck should be equal to the number of cards that were removed from the original hand (from #1). Inside the play() function combine the cards kept by the user and newly drawn cards (from #2) to create a final 5 card draw hand. Use the _print() function that already exists in cards-app to print out the final hand and the remaining number of cards ------------ NodeJS------------------ const inquirer = require('inquirer'); const deck = require('./deck.js'); // helper functions for printing const _print = (result, remaining) => { result.forEach((card) => { console.log(`${card.value} of ${card.suit}`); }); console.log(`Remaining Cards: ${remaining}`); console.log('- - - - - - - - - - - - - - - - - - - - -'); }; // prompt user to select cards to be thrown away const _discardPrompt = async (cards) => { // return a new array of objects where name is what is displayed to the user // and value is what is returned from the inquirer prompt const displayCards = cards.map((card) => { return { name: `${card.value} of ${card.suit}`, value: card.code }; }); // create an inquirer checkbox prompt return inquirer.prompt([ { type: 'checkbox', name: 'cards', message: 'select cards to throw away', // display the cards to the user for them to select choices: displayCards, // validate that the user picks less than 4 cards validate: (cards) => { if (cards.length > 4) { return 'You may only select up to four cards'; } else { return true; } } } ]); }; // add a findAndRemove function // this function is able to find and remove the cards from the original hand // that the user selected to be thrown away // function for playing 5 card draw // deal a user 5 cards and ask them which they want to throwaway // replace those cards with new ones async function play(shuffle) { // hard code the rules of the game const numberOfCards = 5; // get a deck of cards that are either shuffled or not shuffled depending on the user input const deckOfCards = await deck.buildDeck(shuffle); // use destructuring to get the deck_id const { deck_id } = deckOfCards; // draw 5 cards from the deck const originalHand = await deck.drawCards(deck_id, numberOfCards); _print(originalHand.cards, originalHand.remaining); // prompt the user to select which cards to throwaway const throwaway = await _discardPrompt(originalHand.cards); // find and remove the cards from the original hand that need to be thrown away // draw the same number of cards that were removed/thrown away // using the _print function in this file // print out the final hand 5 cards draw hand and the remaining number of cards in the deck } // export the play function so that it can be used in the cli.js module.exports = { play };
- Add a findAndRemove() function which is able the remove the cards from the original hand that the user selected
to be thrown away.
-
Inside the play() function add the logic to draw replacement cards from the SAME deck. The number of cards drawn from the deck should be equal to the number of cards that were removed from the original hand (from #1).
- Inside the play() function combine the cards kept by the user and newly drawn cards (from #2) to create a final 5 card draw hand. Use the _print() function that already exists in cards-app to print out the final hand and the remaining number of cards
------------ NodeJS------------------
const inquirer = require('inquirer');
const deck = require('./deck.js');
// helper functions for printing
const _print = (result, remaining) => {
result.forEach((card) => {
console.log(`${card.value} of ${card.suit}`);
});
console.log(`Remaining Cards: ${remaining}`);
console.log('- - - - - - - - - - - - - - - - - - - - -');
};
// prompt user to select cards to be thrown away
const _discardPrompt = async (cards) => {
// return a new array of objects where name is what is displayed to the user
// and value is what is returned from the inquirer prompt
const displayCards = cards.map((card) => {
return { name: `${card.value} of ${card.suit}`, value: card.code };
});
// create an inquirer checkbox prompt
return inquirer.prompt([
{
type: 'checkbox',
name: 'cards',
message: 'select cards to throw away',
// display the cards to the user for them to select
choices: displayCards,
// validate that the user picks less than 4 cards
validate: (cards) => {
if (cards.length > 4) {
return 'You may only select up to four cards';
} else {
return true;
}
}
}
]);
};
// add a findAndRemove function
// this function is able to find and remove the cards from the original hand
// that the user selected to be thrown away
// function for playing 5 card draw
// deal a user 5 cards and ask them which they want to throwaway
// replace those cards with new ones
async function play(shuffle) {
// hard code the rules of the game
const numberOfCards = 5;
// get a deck of cards that are either shuffled or not shuffled depending on the user input
const deckOfCards = await deck.buildDeck(shuffle);
// use destructuring to get the deck_id
const { deck_id } = deckOfCards;
// draw 5 cards from the deck
const originalHand = await deck.drawCards(deck_id, numberOfCards);
_print(originalHand.cards, originalHand.remaining);
// prompt the user to select which cards to throwaway
const throwaway = await _discardPrompt(originalHand.cards);
// find and remove the cards from the original hand that need to be thrown away
// draw the same number of cards that were removed/thrown away
// using the _print function in this file
// print out the final hand 5 cards draw hand and the remaining number of cards in the deck
}
// export the play function so that it can be used in the cli.js
module.exports = {
play
};

Step by step
Solved in 2 steps

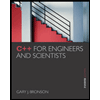
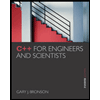