ABC Blood bank wished to welcome and thank all their eligible donors as a symbol of respect. Write a C++ program to welcome and thank all their eligible donors using an explicit call destructor. Strictly adhere to the Object-Oriented Specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. The class Donor has the following public data members. Data Type string Variable Name name age height weight no_of_units_donated int float float int Create a default constructor to display the message "Welcome to the Blood Bank". Check if the donor is eligible to donate the blood using a destructor. • If the age of the donor is above 18 years, then he is eligible, so display the message "Thank you for donating the Blood". • If the age of the donor is less than or equal to 18 years, then he is not eligible, so display the message "You are not eligible to donate the blood". Include the following member function in Donor class Description This method must display the donor details. Display the statement 'Donor details:' inside this method. Member Function void display() In the main method, get the donor details, display the details of the donor by calling display method ane display whether he is eligible or not using destructor.
1) QUESTION PROVIDED IN ATTACH IMAGE KINDLY SEE.
2) IF THERE IS A DECIMAL IN OUTPUT WHETHER IT'S ZERO OR TWO PLACE DECIMAL YOU MUST MATCH AS IT IS WITH QUESTION OUTPUT NO MISMATCH IN OUTPUT ACCEPTED
AND BELOW TEMPLATES PROVIDED CHECK THIS BEFORE MAKING SOLUTION ( main.cpp , donor.cpp )
----------------- TEMPLATES BELOW FOR SOLUTION -----------------------
Main.cpp
#include<iostream> int main()
|
Donor.cpp
#include<iostream> class Donor public:
} |
![ABC Blood bank wished to welcome and thank all their eligible donors as a symbol of respect.
Write a C++ program to welcome and thank all their eligible donors using an explicit call destructor.
Strictly adhere to the Object-Oriented Specifications given in the problem statement. All class
names, member variable names, and function names should be the same as specified in the problem
statement.
The class Donor has the following public data members.
Data Type
string
int
float
Variable Name
name
age
height
weight
no_of_units_donated
float
int
Create a default constructor to display the message "Welcome to the Blood Bank".
Check if the donor is eligible to donate the blood using a destructor.
• If the age of the donor is above 18 years, then he is eligible, so display the message "Thank you for
donating the Blood".
• If the age of the donor is less than or equal to 18 years, then he is not eligible, so display the message
"You are not eligible to donate the blood".
Include the following member function in Donor class
Member Function
Description
void display()
This method must display the donor details.
Display the statement 'Donor details:' inside this method.
In the main method, get the donor details, display the details of the donor by calling display method and
display whether he is eligible or not using destructor.
Input and Output Format:
Refer to the sample input and output for formatting specifications.
[All text in bold are input and the remaining are output]
Sample Input and Output 1:
Welcome to the Blood Bank
Enter the donor details
Enter the Name:
Sowmi
Enter the Age :
22
Enter the height:
156.5
Enter the weight:
47
Enter the No of units to be donated:
1
Donor details:
Sowmi
22
156.5
47
1
Thank you for donating the Blood
Sample Input and Output 2:
Welcome to the Blood Bank
Enter the donor details
Enter the Name:
Aanie
Enter the Age:
4
Enter the height:
90.4
Enter the weight:
25
Enter the No of units to be donated:
1
Donor details:
Aanie
4
90.4
25
1
You are not eligible to donate the blood](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb911f25f-f134-4b9f-b878-c5ce697e6605%2F4636eeb5-2632-446f-9bdc-296fe5228809%2Fy67ez9o_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 4 images

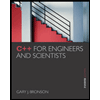
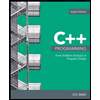
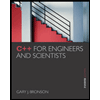
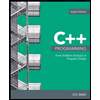