A. The following program is designed to input two numbers and output their sum. It asks the user if he/she would like to run the program. If the answer is Y or y, it prompts the user to enter two numbers. After adding the numbers and displaying the results, it again asks the user if he/she would like to add more numbers. However, the program fails to do so. Correct the C program so that it works properly. #include int main () { char response; double num1, num2; puts(“This program adds two numbers:”); puts(“Would you like to run the program: (Y/y)”); scanf(“%c”, &response); puts(“”); while (response == ‘Y’ && response == ‘y’) { printf(“Enter two numbers: ”); scanf(“%lf %lf”, &num1, &num2); puts(“”); printf(“%.2lf + %.2lf = %.2lf\n”, num1, num2, num1–num2); puts(“Would you like to add again: (Y/y)”); scanf(“%c”, &response); puts(“”); } return 0; } B. The do…while loop in the following program is supposed to read some numbers until it reaches a sentinel (in this case, -1). It is supposed to add all the numbers except for the sentinel. If the data looks like: 12 5 30 48 -1 the program does not add the numbers correctly. Correct the C program so that it adds the numbers correctly #include int main() { int total = 0, count = 0, number; do { scanf(“%d”, &number); total = total + number; count++; } while (number != -1); printf(“The number of data read is %d\n”, count); printf(“The sum of the numbers entered is %d\n”, total); return 0; }
A. The following
like to run the program. If the answer is Y or y, it prompts the user to enter two numbers. After adding the
numbers and displaying the results, it again asks the user if he/she would like to add more numbers. However,
the program fails to do so. Correct the C program so that it works properly.
#include<stdio.h>
int main ()
{
char response;
double num1, num2;
puts(“This program adds two numbers:”);
puts(“Would you like to run the program: (Y/y)”);
scanf(“%c”, &response);
puts(“”);
while (response == ‘Y’ && response == ‘y’)
{
printf(“Enter two numbers: ”);
scanf(“%lf %lf”, &num1, &num2);
puts(“”);
printf(“%.2lf + %.2lf = %.2lf\n”, num1, num2, num1–num2);
puts(“Would you like to add again: (Y/y)”);
scanf(“%c”, &response);
puts(“”);
}
return 0;
}
B. The do…while loop in the following program is supposed to read some numbers until it reaches a sentinel (in this case, -1). It is supposed to add all the numbers except for the sentinel. If the data looks like:
12 5 30 48 -1
the program does not add the numbers correctly. Correct the C program so that it adds the numbers correctly
#include<stdio.h>
int main()
{
int total = 0, count = 0, number;
do
{
scanf(“%d”, &number);
total = total + number;
count++;
} while (number != -1);
printf(“The number of data read is %d\n”, count);
printf(“The sum of the numbers entered is %d\n”, total);
return 0;
}

Step by step
Solved in 3 steps with 4 images

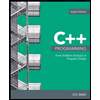
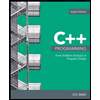