A military academy accepts candidates according to the following height and weight requirements based upon their sex. Sex Min. Height Max. Height Min.Weight Max. Weight Male 65 in. 80 in. 130 lbs. 250 lbs. Female 62 in. 75 in. 110 lbs. 185 lbs. Design and implement a program in C++ that informs the user of the acceptance status of each candidate applying based on the qualifications stated above. The user will input a group of candidates at a time. For each candidate, they will provide sex, height & weight (The sex should be read into a single character variable). Each of the input values should be error-checked. (See below for the minimum and maximum constraints). For each candidate, the program will output whether each candidate was accepted, or rejected based on height, weight, or height & weight. The user will input ‘X’ or ‘x’ for the sex when they are finished entering in all the applicants. The program will output the number of candidates accepted and the percentage of candidates accepted. Run the code 3 times to test it (do not use a for loop for this). • You must first write out a design for the code. It does not need to be created with a tool, but it needs to be readable. You can use pseudocode or a flowchart. Indicate where you will be including functions and design the functions. • The user will input the sex of a single character, height, and weight will be input as whole numbers. • Error check the sex, height, and weight according to the following valid values and ranges: Sex: ‘m’, ‘f’, ‘M’, ‘F’ Height: Between 24” and 110” inclusively (including 24 and 110). Weight: Between 50 lbs. and 1400 lbs. inclusively. * The error messages are specified in the Expected Input / Output section.
A military academy accepts candidates according to the following height and weight requirements based
upon their sex.
Sex Min. Height Max. Height Min.Weight Max. Weight
Male 65 in. 80 in. 130 lbs. 250 lbs.
Female 62 in. 75 in. 110 lbs. 185 lbs.
Design and implement a program in C++ that informs the user of the acceptance status of each candidate
applying based on the qualifications stated above. The user will input a group of candidates at a time. For
each candidate, they will provide sex, height & weight (The sex should be read into a single character
variable). Each of the input values should be error-checked. (See below for the minimum and maximum
constraints). For each candidate, the program will output whether each candidate was accepted, or rejected based
on height, weight, or height & weight. The user will input ‘X’ or ‘x’ for the sex when they are finished entering
in all the applicants. The program will output the number of candidates accepted and the percentage of
candidates accepted. Run the code 3 times to test it (do not use a for loop for this).
• You must first write out a design for the code. It does not need to be created with a tool, but it
needs to be readable. You can use pseudocode or a flowchart. Indicate where you will be including
functions and design the functions.
• The user will input the sex of a single character, height, and weight will be input as whole numbers.
• Error check the sex, height, and weight according to the following valid values and ranges:
Sex: ‘m’, ‘f’, ‘M’, ‘F’
Height: Between 24” and 110” inclusively (including 24 and 110).
Weight: Between 50 lbs. and 1400 lbs. inclusively.
* The error messages are specified in the Expected Input / Output section.
* You cannot use toupper or tolower (or write the code to do the same as the pre-defined function).
Lower & upper case values for sex must be accomplished via compounding Boolean Expressions by
using && or || operators).
• The candidate’s height requirements should be checked. The result should be stored in a Boolean
variable named acceptableHeight. The variable will be true if the candidate meets the requirements
and false if they don’t.
→ this must be accomplished using a single assignment statement that assigns a Boolean expression.
This will be a long compounded expression and you may not break it into multiple expressions or use
any form of selection statement to accomplish this task. In other words, NO selection statements to
assign based on male or female candidates. You will have once in your code:
acceptableHeight = boolean expression;
• The candidate’s weight requirements should be checked. The result should be stored in a Boolean
variable named acceptableWeight.
→ the same restrictions for acceptableHeight apply here
• Using acceptableHeight and acceptableWeight variables, determine whether the candidate’s
acceptance status. Output whether the candidate was accepted, rejected based on height only,
weight only or both height and weight. Output the messages are specified in the Expected
Input/Output section. (You will need to use selection statements to output the correct message).
• At the end of the program output the number of candidates that were accepted and the average
of candidates accepted with respect to the overall number of candidates entered.
• For this program you will need to use a minimum of 4 functions (including a function to output the
class heading). The functions should be designed using good functional design practices discussed in
class. Here are some tips:
- Think about code that is repetitive and create a function for it. Remember that if the repetitive
code uses different values then make those values arguments. If you are cutting and pasting code
or copying code and changing values then you are probably not writing the function properly.
- Make sure that each function manages 1 task (not 1 line of code), but 1 task.
- Functions should be generic when it makes sense to do so. In other words, don’t have 2 functions to
accomplish the same task with different values hard-coded! Pass those values as parameters.
Output needs to look like the image below


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

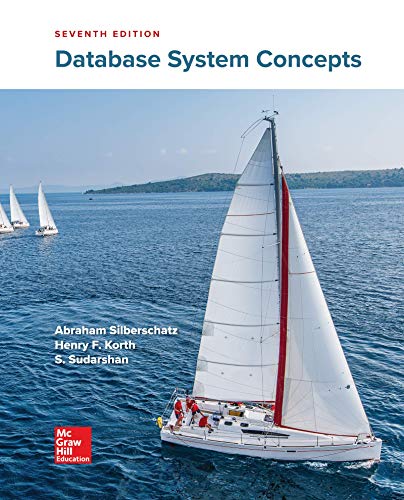
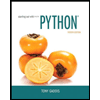
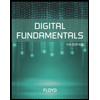
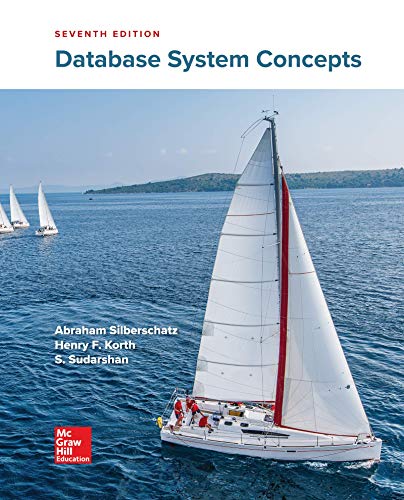
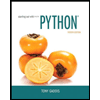
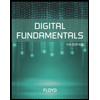
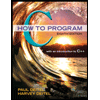
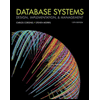
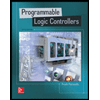