9.24 LAB: Red-black tree Nth largest operation Step 1: Inspect the BSTNode.java and BinarySearch Tree.java files Inspect the BSTNode class declaration for a binary search tree node in BSTNode.java. Access BSTNode.java by clicking on the orange arrow next to LabProgram.java at the top of the coding window. The BSTNode class has private fields for the key, parent reference, left child reference, and right child reference. Accessor methods exist for each. Inspect the BinarySearch Tree class declaration for a binary search tree node in BinarySearch Tree.java. The getNthKey() method is the only abstract method that exists. Step 2: Inspect other files related to the inheritance hierarchy Classes RBTNode and RedBlackTree inherit from BSTNode and BinarySearch Tree, respectively. Each class is implemented in a read only file. Classes Extended RBTNode and Extended Red BlackTree are declared, but implementations are incomplete. Both classes must be implemented in this lab. BinarySearch Tree RedBlackTree BSTNode RBTNode Extended RedBlack Tree Extended RBTNode Read only, completed class implementation Incomplete class implementation Step 3: Understand the purpose of the subtreeKeyCount variable The Extended RBTNode class inherits from RBTNode and adds one integer field, subtreeKeyCount. Each node's subtree key count is the number of keys in the subtree rooted at that node. Ex: 20 7 10 2 42 1 19 30 1 55 2 0 5 77 Subtree key count 1 Extended RBTNode's constructor and getSubtreeKeyCount() methods are already implemented and should not be changed. Additional methods are needed to ensure that subtreeKeyCount remains accurate. Step 4: Implement Extended Red BlackTree and Extended RBTNode Each node in an Extended Red Black Tree must have a correct subtreeKeyCount after an insertion or removal operation. Determine which methods in Red Black Tree and RBTNode must be overridden in Extended Red BlackTree and Extended RBTNode to keep each node's subtreeKeyCount correct. New methods can be added along with overridden methods, if desired. Hint: Consider an updateSubtreeKeyCount() method for the Extended RBTNode class. The method requires each child node's subtreeKeyCount to be correct, and updates the node's subtreeKeyCount appropriately. Overridden methods in both Extended RBTNode and Extended RedBlackTree can call a node's updateSubtreeKeyCount() method as needed. Once determinations are made, complete the implementation of both the Extended Red Black Tree and Extended RBTNode classes. Do not implement Extended Red Black Tree's getNthKey() in this step. getNthKey() requires correct subtree counts at each node. Step 5: Run tests in develop mode and submit mode Tree TestCommand is an abstract base class defined in Tree TestCommand.java. A Tree TestCommand object is an executable command that operates on a binary search tree. Classes inheriting from TreeTestCommand are declared in their respective files: • TreelnsertCommand inserts keys into the tree . • TreeRemoveCommand removes keys from the tree TreeVerifyKeysCommand verifies the tree's keys using an inorder traversal • TreeVerifySubtree Counts Command verifies that each node in the tree has the expected subtree key count • TreeGetNthCommand verifies that getNthKey() returns an expected value Code in LabProgram.java contains three automated test cases. Each test executes an ArrayList of Tree TestCommand objects in sequence. The third test includes TreeGetNth Commands and will not pass until the completion of Step 6. The first two tests should pass after completion of step 4. Before proceeding to Step 6, run code in develop mode and ensure that the first two tests in LabProgram.java pass. Then submit code and ensure that the first two unit tests pass. Step 6: Implement Extended RedBlackTree's getNthKey() method (worst case O(log n)) getNthKey() must return the tree's nth-largest key. The parameter n starts at 0 for the smallest key in the tree. Ex: Suppose a tree has keys: 10, 19, 20, 30, 42, 55, 77 Then getNthKey (0) returns 10, getNthKey (1) returns 19, ..., getNthKey (5) returns 55, and getNthKey (6) returns 77. Determine an algorithm that uses the subtree key counts so that getNthKey() operates in worst case O(log n) time.
9.24 LAB: Red-black tree Nth largest operation Step 1: Inspect the BSTNode.java and BinarySearch Tree.java files Inspect the BSTNode class declaration for a binary search tree node in BSTNode.java. Access BSTNode.java by clicking on the orange arrow next to LabProgram.java at the top of the coding window. The BSTNode class has private fields for the key, parent reference, left child reference, and right child reference. Accessor methods exist for each. Inspect the BinarySearch Tree class declaration for a binary search tree node in BinarySearch Tree.java. The getNthKey() method is the only abstract method that exists. Step 2: Inspect other files related to the inheritance hierarchy Classes RBTNode and RedBlackTree inherit from BSTNode and BinarySearch Tree, respectively. Each class is implemented in a read only file. Classes Extended RBTNode and Extended Red BlackTree are declared, but implementations are incomplete. Both classes must be implemented in this lab. BinarySearch Tree RedBlackTree BSTNode RBTNode Extended RedBlack Tree Extended RBTNode Read only, completed class implementation Incomplete class implementation Step 3: Understand the purpose of the subtreeKeyCount variable The Extended RBTNode class inherits from RBTNode and adds one integer field, subtreeKeyCount. Each node's subtree key count is the number of keys in the subtree rooted at that node. Ex: 20 7 10 2 42 1 19 30 1 55 2 0 5 77 Subtree key count 1 Extended RBTNode's constructor and getSubtreeKeyCount() methods are already implemented and should not be changed. Additional methods are needed to ensure that subtreeKeyCount remains accurate. Step 4: Implement Extended Red BlackTree and Extended RBTNode Each node in an Extended Red Black Tree must have a correct subtreeKeyCount after an insertion or removal operation. Determine which methods in Red Black Tree and RBTNode must be overridden in Extended Red BlackTree and Extended RBTNode to keep each node's subtreeKeyCount correct. New methods can be added along with overridden methods, if desired. Hint: Consider an updateSubtreeKeyCount() method for the Extended RBTNode class. The method requires each child node's subtreeKeyCount to be correct, and updates the node's subtreeKeyCount appropriately. Overridden methods in both Extended RBTNode and Extended RedBlackTree can call a node's updateSubtreeKeyCount() method as needed. Once determinations are made, complete the implementation of both the Extended Red Black Tree and Extended RBTNode classes. Do not implement Extended Red Black Tree's getNthKey() in this step. getNthKey() requires correct subtree counts at each node. Step 5: Run tests in develop mode and submit mode Tree TestCommand is an abstract base class defined in Tree TestCommand.java. A Tree TestCommand object is an executable command that operates on a binary search tree. Classes inheriting from TreeTestCommand are declared in their respective files: • TreelnsertCommand inserts keys into the tree . • TreeRemoveCommand removes keys from the tree TreeVerifyKeysCommand verifies the tree's keys using an inorder traversal • TreeVerifySubtree Counts Command verifies that each node in the tree has the expected subtree key count • TreeGetNthCommand verifies that getNthKey() returns an expected value Code in LabProgram.java contains three automated test cases. Each test executes an ArrayList of Tree TestCommand objects in sequence. The third test includes TreeGetNth Commands and will not pass until the completion of Step 6. The first two tests should pass after completion of step 4. Before proceeding to Step 6, run code in develop mode and ensure that the first two tests in LabProgram.java pass. Then submit code and ensure that the first two unit tests pass. Step 6: Implement Extended RedBlackTree's getNthKey() method (worst case O(log n)) getNthKey() must return the tree's nth-largest key. The parameter n starts at 0 for the smallest key in the tree. Ex: Suppose a tree has keys: 10, 19, 20, 30, 42, 55, 77 Then getNthKey (0) returns 10, getNthKey (1) returns 19, ..., getNthKey (5) returns 55, and getNthKey (6) returns 77. Determine an algorithm that uses the subtree key counts so that getNthKey() operates in worst case O(log n) time.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write the full Java Code for ExtendedRBTNode.Java and the ExtendedRedBlackTree.java

Transcribed Image Text:9.24 LAB: Red-black tree Nth largest operation
Step 1: Inspect the BSTNode.java and BinarySearch Tree.java files
Inspect the BSTNode class declaration for a binary search tree node in BSTNode.java. Access BSTNode.java by clicking on the orange
arrow next to LabProgram.java at the top of the coding window. The BSTNode class has private fields for the key, parent reference, left child
reference, and right child reference. Accessor methods exist for each.
Inspect the BinarySearch Tree class declaration for a binary search tree node in BinarySearch Tree.java. The getNthKey() method is the only
abstract method that exists.
Step 2: Inspect other files related to the inheritance hierarchy
Classes RBTNode and RedBlackTree inherit from BSTNode and BinarySearch Tree, respectively. Each class is implemented in a read only
file.
Classes Extended RBTNode and Extended Red BlackTree are declared, but implementations are incomplete. Both classes must be
implemented in this lab.
BinarySearch Tree
RedBlackTree
BSTNode
RBTNode
Extended RedBlack Tree
Extended RBTNode
Read only, completed class implementation
Incomplete class implementation
Step 3: Understand the purpose of the subtreeKeyCount variable
The Extended RBTNode class inherits from RBTNode and adds one integer field, subtreeKeyCount. Each node's subtree key count is the
number of keys in the subtree rooted at that node. Ex:
20
7
10
2
42
1
19
30 1
55
2
0
5
77
Subtree key count
1
Extended RBTNode's constructor and getSubtreeKeyCount() methods are already implemented and should not be changed. Additional
methods are needed to ensure that subtreeKeyCount remains accurate.

Transcribed Image Text:Step 4: Implement Extended Red BlackTree and Extended RBTNode
Each node in an Extended Red Black Tree must have a correct subtreeKeyCount after an insertion or removal operation. Determine which
methods in Red Black Tree and RBTNode must be overridden in Extended Red BlackTree and Extended RBTNode to keep each node's
subtreeKeyCount correct. New methods can be added along with overridden methods, if desired.
Hint: Consider an updateSubtreeKeyCount() method for the Extended RBTNode class. The method requires each child node's
subtreeKeyCount to be correct, and updates the node's subtreeKeyCount appropriately. Overridden methods in both Extended RBTNode and
Extended RedBlackTree can call a node's updateSubtreeKeyCount() method as needed.
Once determinations are made, complete the implementation of both the Extended Red Black Tree and Extended RBTNode classes. Do not
implement Extended Red Black Tree's getNthKey() in this step. getNthKey() requires correct subtree counts at each node.
Step 5: Run tests in develop mode and submit mode
Tree TestCommand is an abstract base class defined in Tree TestCommand.java. A Tree TestCommand object is an executable command
that operates on a binary search tree. Classes inheriting from TreeTestCommand are declared in their respective files:
• TreelnsertCommand inserts keys into the tree
.
•
TreeRemoveCommand removes keys from the tree
TreeVerifyKeysCommand verifies the tree's keys using an inorder traversal
• TreeVerifySubtree Counts Command verifies that each node in the tree has the expected subtree key count
• TreeGetNthCommand verifies that getNthKey() returns an expected value
Code in LabProgram.java contains three automated test cases. Each test executes an ArrayList of Tree TestCommand objects in sequence.
The third test includes TreeGetNth Commands and will not pass until the completion of Step 6. The first two tests should pass after
completion of step 4.
Before proceeding to Step 6, run code in develop mode and ensure that the first two tests in LabProgram.java pass. Then submit code and
ensure that the first two unit tests pass.
Step 6: Implement Extended RedBlackTree's getNthKey() method (worst case O(log n))
getNthKey() must return the tree's nth-largest key. The parameter n starts at 0 for the smallest key in the tree. Ex: Suppose a tree has keys:
10, 19, 20, 30, 42, 55, 77
Then getNthKey (0) returns 10, getNthKey (1) returns 19, ..., getNthKey (5) returns 55, and getNthKey (6) returns 77.
Determine an algorithm that uses the subtree key counts so that getNthKey() operates in worst case O(log n) time.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
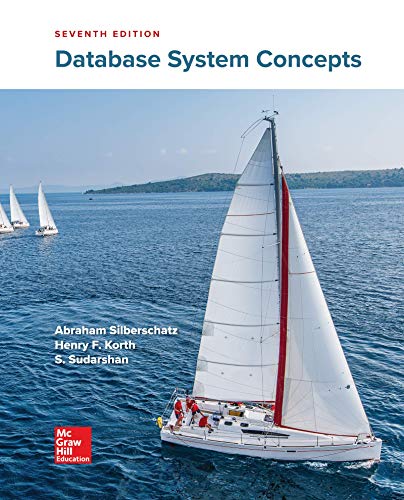
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
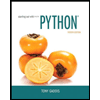
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
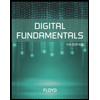
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
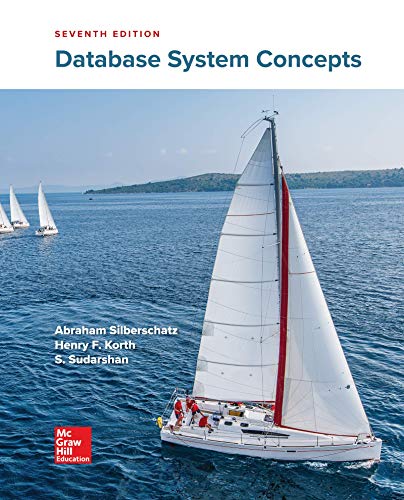
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
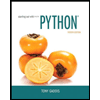
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
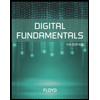
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
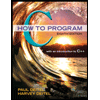
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
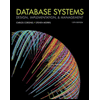
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
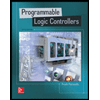
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education