5. Convert the following high-level code into RISC-V assembly language. Assume that the signed integer variables g and ʼn are in registers to and t1, respectively. You can use other temporary registers like t2 and t3 if needed. a) b) if (g % 8 == 3 || g % 8 == 5) h = h * 5.5; else h = h 16; if (g % 16 > 4 && g % 16 < 12) h = (g + 5 * h) % 8; else h = (g+ 8.5 * h) * 9;
5. Convert the following high-level code into RISC-V assembly language. Assume that the signed integer variables g and ʼn are in registers to and t1, respectively. You can use other temporary registers like t2 and t3 if needed. a) b) if (g % 8 == 3 || g % 8 == 5) h = h * 5.5; else h = h 16; if (g % 16 > 4 && g % 16 < 12) h = (g + 5 * h) % 8; else h = (g+ 8.5 * h) * 9;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
DO NOT USE nor, li, etc....ONLY USE RISC-V assembly code (only use instructions from pictures below)
![RISC-V RV321 Instructions Subset
R-type Instructions
ALU performs the required operations on two source registers (rs1 and rs2) and stores ALU result back to the
destination register rd.
I-type ALU Instructions
ALU performs the required operations on the source register rs1 and immediate constant, and stores the ALU result
back to the destination register rd.
Category
Arithmetic
Logical
TABLE 3.5 Basic Identities of Boolean Algebra
Shift
Instruction
Add
Subtract
Add immediate
Set less than
Set less than immediate
Set less than unsigned
Set less than immediate, unsigned
And
Inclusive or
Exclusive or
And immediate
Inclusive or immediate
Exclusive or immediate
Shift left logical
Shift right logical
Shift right arithmetic
Shift left logical immediate
Shift right logical immediate
Shift right arithmetic immediate
Example
add x5, x6, x7
sub x5, x6, x7
addi x5, x6, 20
slt x5, x6, x7
slti x5, x6, 20
sltu x5, x6, x7
sltiu x5, x6, 20
and x5, x6, x7
or x5, x6, x8
xor x5, x6, x9
andi x5, x6, 20
ori x5, x6, 20
xori x5, x6, 20
sll x5, x6, x7
srl x5, x6, x7
sra x5, x6, x7
slli x5, x6, 3
srli x5, x6, 3
srai x5, x6, 3
Meaning
x5 = x6 +x7
x5 = x6 - x7
x5 = x6 + 20
x5 = x6 < x7
x5 = x6 < 20
x5 = x6 <₁ x7
x5 = x6 <₁ 20
x5 = x6 & x7
x5 = x6 | x8
x5 = x6 ^ x9
x5 = x6 & 20
x5 = x6 | 20
x5 = x6 ^ 20
x5 = x6 << x7
x5 = x6 >> x7
x5 = x6 >> x7
x5 = x6 << 3
x5 = x6 >> 3
x5 = x6 >> 3
Comments
Three register operands; add
Three register operands; subtract
Used to add constants
Used to compare two registers as 2's complement numbers
Used to compare a register to a constant as 2's complement numbers
Used to compare two registers as unsigned numbers
Used to compare a register to a constant as unsigned numbers
Three register operands; bit-by-bit AND
Three register operands; bit-by-bit OR
Three register operands; bit-by-bit XOR
Bit-by-bit AND register with constant
Bit-by-bit OR register with constant
Bit-by-bit XOE register with constant
Shift left by register
Shift right by register
Arithmetic shift right by register
Shift left by immediate
Shift right by immediate
Arithmetic shift right by immediate
Data Transfer
Memory Read (I-type Instructions)
Read memory at address (Reg [rsl] + offset) and store to the destination register rd.
Memory Write (S-type Instructions)
Write register content (Reg [rs2]) to memory at address (Reg [rs1] + offset).
Category
Data Transfer
Instruction
lui
Instruction
Example
lb x5, 40(x6)
Ibu x5, 40(x6)
Ih x5, 40(x6)
Ihu x5, 40(x6)
Store byte
Iw x5, 40(x6)
sb x5, 40(x6)
sh x5, 40(x6)
sw x5, 40(x6)
Store halfword
Store word
Load upper immediate
lui x5, 0x12345
Add upper immediate to PC auipc x5, 0x12345
Category
Load byte
Load byte, unsigned
Load halfword
Conditional
branch
Load halfword, unsigned
Load word
U-Type Instructions
Lui: load upper immediate instruction which load 20-bit immediate constant from the instruction and store
to the upper 20 bits of the destination register rd.
x5, 0xAC516
x5= 0xAC516000
Meaning
Instruction
Branch if equal
Branch if not equal
Branch if less than
Branch if greater or
equal
Branch if less, unsigned
Branch if greater or
equal, unsigned
Unconditional Jump and link
branch
Jump and link register
Meaning
x5 = Memory [x6+40]
x5 = Memory [x6+40]
x5 = Memory [x6 + 40]
x5 = Memory [x6+40]
x5 = Memory [x6 + 40]
Memory[x6 +40] = x5
Memory[x6 +40] = x5
Memory[x6 + 40] = x5
x50x12345000
x5 PC + 0x12345000
Example
beq x5, x6, 100
bne x5, x6, 100
blt x5, x6, 100
bge x5, x6, 100
Branch Instructions (B-type and J-type)
Conditional branch instructions: B-type (if branch condition is true, then go to label; otherwise continue to the next
instruction after the branch instruction)
Unconditional branch instructions: J-type (used for function calls and returns, these two instructions are used as a
pair.
bltu x5, x6, 100
bgeu x5, x6, 100
Byte from memory to register
Unsigned byte from memory to register
Halfword from memory to register
Unsigned halfword from memory to register
Word from memory to register
Byte from register to memory
Halfword from register to memory
Word from register to memory
Loads 20-it constant shifted left 12 bits
Used for PC-relative data addressing
jal x1, 100
jalr x1, 100 (x5)
Comments
U-Type Format
00101
1010 1100 0101 0001 0110
Meaning
if (x5 x6) go to PC+100
if (x5 x6) go to PC+100
if (x5 x6) go to PC+100
if (x5 > x6) go to PC+100
if (x5 x6) go to PC+100
if (x5 > x6) go to PC+100
x1 = PC+4; go to PC+100
x1 = PC+4; go to x5+100
0110111
Comments
PC-relative branch if registers equal
PC-relative branch if registers not equal
PC-relative branch if registers less
PC-relative branch if registers greater
or equal
PC-relative branch if registers less,
unsigned
PC-relative branch if registers greater
or equal, unsigned
PC-relative procedure call
Procedure return; indirect call](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe8a4e2aa-1e62-464b-b545-05382b87852d%2F2183a53e-84c3-4d87-8b58-145980ceeced%2Fgq7fsgk_processed.jpeg&w=3840&q=75)
Transcribed Image Text:RISC-V RV321 Instructions Subset
R-type Instructions
ALU performs the required operations on two source registers (rs1 and rs2) and stores ALU result back to the
destination register rd.
I-type ALU Instructions
ALU performs the required operations on the source register rs1 and immediate constant, and stores the ALU result
back to the destination register rd.
Category
Arithmetic
Logical
TABLE 3.5 Basic Identities of Boolean Algebra
Shift
Instruction
Add
Subtract
Add immediate
Set less than
Set less than immediate
Set less than unsigned
Set less than immediate, unsigned
And
Inclusive or
Exclusive or
And immediate
Inclusive or immediate
Exclusive or immediate
Shift left logical
Shift right logical
Shift right arithmetic
Shift left logical immediate
Shift right logical immediate
Shift right arithmetic immediate
Example
add x5, x6, x7
sub x5, x6, x7
addi x5, x6, 20
slt x5, x6, x7
slti x5, x6, 20
sltu x5, x6, x7
sltiu x5, x6, 20
and x5, x6, x7
or x5, x6, x8
xor x5, x6, x9
andi x5, x6, 20
ori x5, x6, 20
xori x5, x6, 20
sll x5, x6, x7
srl x5, x6, x7
sra x5, x6, x7
slli x5, x6, 3
srli x5, x6, 3
srai x5, x6, 3
Meaning
x5 = x6 +x7
x5 = x6 - x7
x5 = x6 + 20
x5 = x6 < x7
x5 = x6 < 20
x5 = x6 <₁ x7
x5 = x6 <₁ 20
x5 = x6 & x7
x5 = x6 | x8
x5 = x6 ^ x9
x5 = x6 & 20
x5 = x6 | 20
x5 = x6 ^ 20
x5 = x6 << x7
x5 = x6 >> x7
x5 = x6 >> x7
x5 = x6 << 3
x5 = x6 >> 3
x5 = x6 >> 3
Comments
Three register operands; add
Three register operands; subtract
Used to add constants
Used to compare two registers as 2's complement numbers
Used to compare a register to a constant as 2's complement numbers
Used to compare two registers as unsigned numbers
Used to compare a register to a constant as unsigned numbers
Three register operands; bit-by-bit AND
Three register operands; bit-by-bit OR
Three register operands; bit-by-bit XOR
Bit-by-bit AND register with constant
Bit-by-bit OR register with constant
Bit-by-bit XOE register with constant
Shift left by register
Shift right by register
Arithmetic shift right by register
Shift left by immediate
Shift right by immediate
Arithmetic shift right by immediate
Data Transfer
Memory Read (I-type Instructions)
Read memory at address (Reg [rsl] + offset) and store to the destination register rd.
Memory Write (S-type Instructions)
Write register content (Reg [rs2]) to memory at address (Reg [rs1] + offset).
Category
Data Transfer
Instruction
lui
Instruction
Example
lb x5, 40(x6)
Ibu x5, 40(x6)
Ih x5, 40(x6)
Ihu x5, 40(x6)
Store byte
Iw x5, 40(x6)
sb x5, 40(x6)
sh x5, 40(x6)
sw x5, 40(x6)
Store halfword
Store word
Load upper immediate
lui x5, 0x12345
Add upper immediate to PC auipc x5, 0x12345
Category
Load byte
Load byte, unsigned
Load halfword
Conditional
branch
Load halfword, unsigned
Load word
U-Type Instructions
Lui: load upper immediate instruction which load 20-bit immediate constant from the instruction and store
to the upper 20 bits of the destination register rd.
x5, 0xAC516
x5= 0xAC516000
Meaning
Instruction
Branch if equal
Branch if not equal
Branch if less than
Branch if greater or
equal
Branch if less, unsigned
Branch if greater or
equal, unsigned
Unconditional Jump and link
branch
Jump and link register
Meaning
x5 = Memory [x6+40]
x5 = Memory [x6+40]
x5 = Memory [x6 + 40]
x5 = Memory [x6+40]
x5 = Memory [x6 + 40]
Memory[x6 +40] = x5
Memory[x6 +40] = x5
Memory[x6 + 40] = x5
x50x12345000
x5 PC + 0x12345000
Example
beq x5, x6, 100
bne x5, x6, 100
blt x5, x6, 100
bge x5, x6, 100
Branch Instructions (B-type and J-type)
Conditional branch instructions: B-type (if branch condition is true, then go to label; otherwise continue to the next
instruction after the branch instruction)
Unconditional branch instructions: J-type (used for function calls and returns, these two instructions are used as a
pair.
bltu x5, x6, 100
bgeu x5, x6, 100
Byte from memory to register
Unsigned byte from memory to register
Halfword from memory to register
Unsigned halfword from memory to register
Word from memory to register
Byte from register to memory
Halfword from register to memory
Word from register to memory
Loads 20-it constant shifted left 12 bits
Used for PC-relative data addressing
jal x1, 100
jalr x1, 100 (x5)
Comments
U-Type Format
00101
1010 1100 0101 0001 0110
Meaning
if (x5 x6) go to PC+100
if (x5 x6) go to PC+100
if (x5 x6) go to PC+100
if (x5 > x6) go to PC+100
if (x5 x6) go to PC+100
if (x5 > x6) go to PC+100
x1 = PC+4; go to PC+100
x1 = PC+4; go to x5+100
0110111
Comments
PC-relative branch if registers equal
PC-relative branch if registers not equal
PC-relative branch if registers less
PC-relative branch if registers greater
or equal
PC-relative branch if registers less,
unsigned
PC-relative branch if registers greater
or equal, unsigned
PC-relative procedure call
Procedure return; indirect call

Transcribed Image Text:5.
Convert the following high-level code into RISC-V assembly language. Assume
that the signed integer variables g and ʼn are in registers to and t1, respectively. You can
use other temporary registers like t2 and t3 if needed.
a)
b)
if (g % 8 == 3 || g % 8 == 5)
h = h * 5.5;
else
h = h 16;
if (g % 16 > 4 && g % 16 < 12)
h = (g + 5 * h) % 8;
else
h = (g + 8.5 * h)
* 9;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
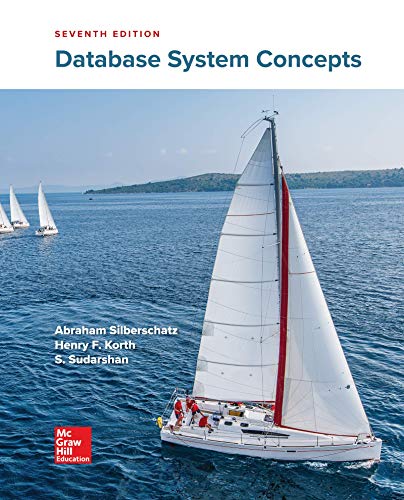
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
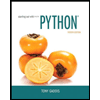
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
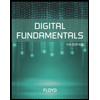
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
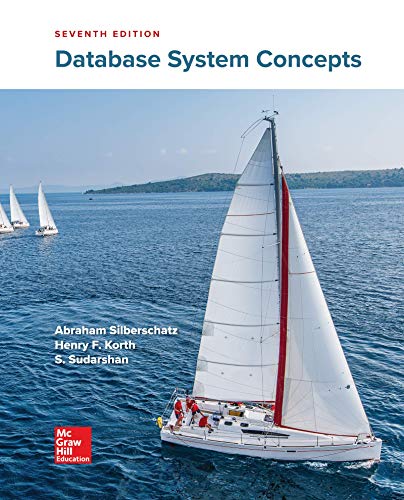
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
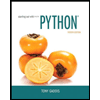
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
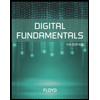
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
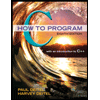
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
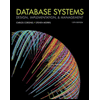
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
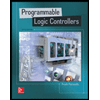
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education