20.13 LAB: Doubly linked list In this lab you are asked to complete the python code so that it: • Accepts integers as inputs from a user and appends them to the doubly linked list until the user enters -1 • Next, the user enters an integer and your code should: o find this in the doubly linked list o remove that node and all the nodes that comes after it in the list o If the node is not found in the doubly-linked-list, it should display: "Node not found", • Display the modified doubly linked list o if the doubly linked list is empty it should print "Empty!" For example, for following input 2
class Node:
def __init__(self, data):
self.data = data
self.next = None
self.prev = None
# Empty Doubly Linked List
class DoublyLinkedList:
def __init__(self):
self.head = None
def append(self, new_data):
if self.head == None:
self.head = new_data
self.tail = new_data
else:
self.tail.next = new_data
new_data.prev = self.tail
self.tail = new_data
def printList(self):
if(self.head == None):
print('Empty!')
else:
node = self.head
while(node is not None):
print(node.data),
node = node.next
def remove(self, current_node):
successor_node = current_node.next
predecessor_node = current_node.prev
if successor_node is not None:
successor_node.prev = predecessor_node
if predecessor_node is not None:
predecessor_node.next = successor_node
if current_node is self.head:
self.head = successor_node
if current_node is self.tail:
self.tail = predecessor_node
def node_search(self,nodeA):
#** Your code goes here **
return self
def chunck_removal(self,nodeA):
#** Your code goes here **
return self
if __name__ == '__main__':
#** Your code goes here **



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

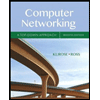
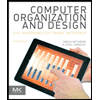
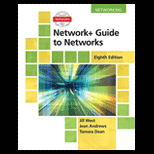
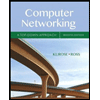
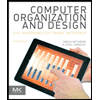
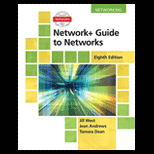
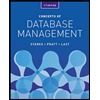
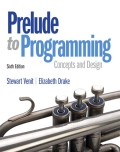
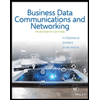