1.1. Test to see if the unsorted set contains the value 10. 1.2. Test to see if the sorted set contains the value 5. 1.3. Remove the element 10 from the unsorted set. 1.4. Test to see if the unsorted set still contains the value 10. 1.5. Add the value 8 to the sorted set. 1.6. Test to see if the sorted set contains the value 8. 1.7. Display the unsorted set. 1.8. Display the sorted set. (The sorted set should now have two more values than the unsorted set.) 1.9. Add all values from the sorted set to the unsorted set by calling unsortedSet.addAll(sortedSet). 1.10. Display both sets and confirm they have the same contents, just in a different order. Sample program output follows. Your code must use the values listed above, even if they differ from the sample output. Does the unsorted set contain 10? true Does the sorted set contain 5? false Removing 10 from unsorted set. Does the unsorted set contain 10? false Adding 5 to sorted set. Does the sorted set in 5? true The unsorted set is: [34, 22, 60, 30] The sorted set is: [5, 10, 22, 30, 34, 60] Adding all from sorted set to unsorted set. The unsorted set is: [34, 5, 22, 10, 60, 30] The sorted set is: [5, 10, 22, 30, 34, 60]
1.1. Test to see if the unsorted set contains the value 10. 1.2. Test to see if the sorted set contains the value 5. 1.3. Remove the element 10 from the unsorted set. 1.4. Test to see if the unsorted set still contains the value 10. 1.5. Add the value 8 to the sorted set. 1.6. Test to see if the sorted set contains the value 8. 1.7. Display the unsorted set. 1.8. Display the sorted set. (The sorted set should now have two more values than the unsorted set.) 1.9. Add all values from the sorted set to the unsorted set by calling unsortedSet.addAll(sortedSet). 1.10. Display both sets and confirm they have the same contents, just in a different order. Sample program output follows. Your code must use the values listed above, even if they differ from the sample output. Does the unsorted set contain 10? true Does the sorted set contain 5? false Removing 10 from unsorted set. Does the unsorted set contain 10? false Adding 5 to sorted set. Does the sorted set in 5? true The unsorted set is: [34, 22, 60, 30] The sorted set is: [5, 10, 22, 30, 34, 60] Adding all from sorted set to unsorted set. The unsorted set is: [34, 5, 22, 10, 60, 30] The sorted set is: [5, 10, 22, 30, 34, 60]
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![1.1. Test to see if the unsorted set contains the value 10.
1.2. Test to see if the sorted set contains the value 5.
1.3. Remove the element 10 from the unsorted set.
1.4. Test to see if the unsorted set still contains the value 10.
1.5. Add the value 8 to the sorted set.
1.6. Test to see if the sorted set contains the value 8.
1.7. Display the unsorted set.
1.8. Display the sorted set. (The sorted set should now have two more values than the
unsorted set.)
1.9. Add all values from the sorted set to the unsorted set by calling
unsortedSet.addAll(sortedSet).
1.10. Display both sets and confirm they have the same contents, just in a different order.
Sample program output follows.
Your code must use the values listed above, even if they differ from the sample output.
Does the unsorted set contain 10? true
Does the sorted set contain 5? false
Removing 10 from unsorted set.
Does the unsorted set contain 10? false
Adding 5 to sorted set.
Does the sorted set contain 5? true
The unsorted set is:
[34, 22, 60, 30]
The sorted set is: [5, 10, 22, 30, 34, 60]
Adding all from sorted set to unsorted set.
The unsorted set is: [34, 5, 22, 10, 60, 30]
The sorted set is: [5, 10, 22, 30, 34, 60]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fca76fac2-1440-4992-8707-39fd3f211841%2Fb3cbb34e-abd5-45b0-b1ca-07d56aec71f9%2Fys5thqh_processed.png&w=3840&q=75)
Transcribed Image Text:1.1. Test to see if the unsorted set contains the value 10.
1.2. Test to see if the sorted set contains the value 5.
1.3. Remove the element 10 from the unsorted set.
1.4. Test to see if the unsorted set still contains the value 10.
1.5. Add the value 8 to the sorted set.
1.6. Test to see if the sorted set contains the value 8.
1.7. Display the unsorted set.
1.8. Display the sorted set. (The sorted set should now have two more values than the
unsorted set.)
1.9. Add all values from the sorted set to the unsorted set by calling
unsortedSet.addAll(sortedSet).
1.10. Display both sets and confirm they have the same contents, just in a different order.
Sample program output follows.
Your code must use the values listed above, even if they differ from the sample output.
Does the unsorted set contain 10? true
Does the sorted set contain 5? false
Removing 10 from unsorted set.
Does the unsorted set contain 10? false
Adding 5 to sorted set.
Does the sorted set contain 5? true
The unsorted set is:
[34, 22, 60, 30]
The sorted set is: [5, 10, 22, 30, 34, 60]
Adding all from sorted set to unsorted set.
The unsorted set is: [34, 5, 22, 10, 60, 30]
The sorted set is: [5, 10, 22, 30, 34, 60]
![Please enter the following code into a Java file.
import java.util.*;
public class SetDemo {
int count[]
public static void main(String args[]) {
{34, 22,10,60,30,22};
Set<Integer> unsortedSet = new HashSet<Integer>();
try {
for(int i = 0; i < count.length; i++) {
unsortedSet.add(count[i]);
}
}
}
=
System.out.println("The unsorted set is: + unsortedSet);
11
TreeSet sorted Set = new TreeSet<Integer>(unsortedSet);
System.out.println("The sorted set is:
System.out.println("The
System.out.println("The
catch(Exception e) {}
+ sorted Set);
first element of the set is: "+ (Integer) sorted Set.first());
last element of the set is: "+ (Integer)sortedSet.last());
Run the program to confirm it works as expected. Then, expand the program to do the
following steps in order. For each test, display the result of the test to the console.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fca76fac2-1440-4992-8707-39fd3f211841%2Fb3cbb34e-abd5-45b0-b1ca-07d56aec71f9%2Fs8d5kt7_processed.png&w=3840&q=75)
Transcribed Image Text:Please enter the following code into a Java file.
import java.util.*;
public class SetDemo {
int count[]
public static void main(String args[]) {
{34, 22,10,60,30,22};
Set<Integer> unsortedSet = new HashSet<Integer>();
try {
for(int i = 0; i < count.length; i++) {
unsortedSet.add(count[i]);
}
}
}
=
System.out.println("The unsorted set is: + unsortedSet);
11
TreeSet sorted Set = new TreeSet<Integer>(unsortedSet);
System.out.println("The sorted set is:
System.out.println("The
System.out.println("The
catch(Exception e) {}
+ sorted Set);
first element of the set is: "+ (Integer) sorted Set.first());
last element of the set is: "+ (Integer)sortedSet.last());
Run the program to confirm it works as expected. Then, expand the program to do the
following steps in order. For each test, display the result of the test to the console.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
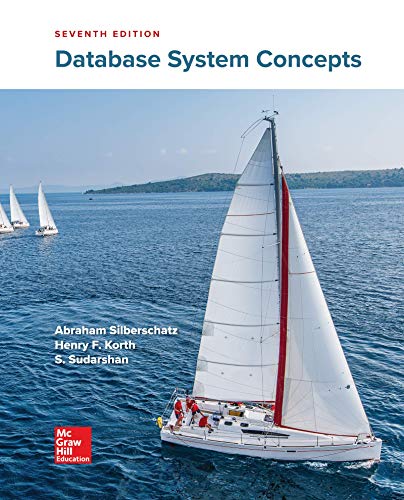
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
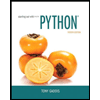
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
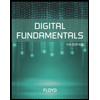
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
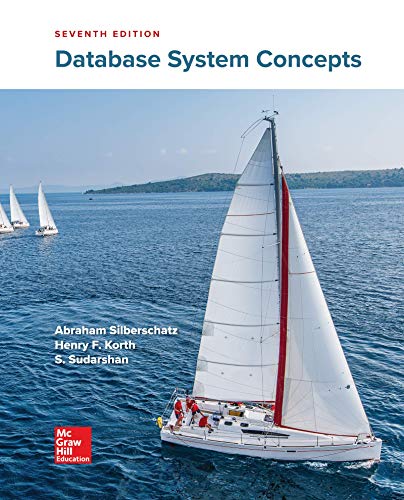
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
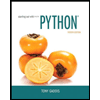
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
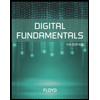
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
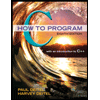
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
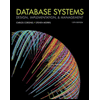
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
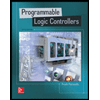
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education