1. Create a properly encapsulated class named Person that has the following: A String instance variable called name. ● ● A correctly overrided equals method which returns true if the name instance variable of the parameter and the reference variable calling the method are equal. (Do not forget the null and class type checks!) Compile your Person class. 2. Create a properly encapsulated class named Employee that inherits from Person and has the following: ● ● ● ● A constructor that takes three Strings as parameters for name, office, and hourlyWage and sets the instance variables of both the parent class and the Employee class. An overridden toString method that returns the concatenation of name and hourlyWage instance variables (separated by a space). A method named paycheck that takes an integer parameter (# of hours) and returns a double. The method should calculate and return the total wage (# of hours * hourlyWage). You can assume that the hourlyWage instance variable will always have a format of a dollar sign followed by at least one digit. Compile your Employee class. 3. Create a class named EmployeeTest that has a main method. The class should do the following: Create an Employee object and pass in "Fred" for name, "RR 205" for office, and "$38.50" for hourlyWage. You may use a reference variable name of your choice. Call the toString method and print the result. ● ● ● ● ● A constructor that takes a String parameter and sets the instance variable. A properly named getter for the instance variable. ● ● A String instance variable called office (e.g. "LWH 2222"). A String instance variable called hourlywage (e.g. "$45.15"). Call the paycheck method, pass in a value of 20 for the parameter; print the result. Create a Person object and pass in "Fred" for name. You may use a reference variable name of your choice. Call the equals method on the "Fred" Employee object and pass in the reference to the "Fred" Person object. Print out the result. Compile and run your Employee Test class. Your output should match the sample output below: Fred $38.50 770.0 false
1. Create a properly encapsulated class named Person that has the following: A String instance variable called name. ● ● A correctly overrided equals method which returns true if the name instance variable of the parameter and the reference variable calling the method are equal. (Do not forget the null and class type checks!) Compile your Person class. 2. Create a properly encapsulated class named Employee that inherits from Person and has the following: ● ● ● ● A constructor that takes three Strings as parameters for name, office, and hourlyWage and sets the instance variables of both the parent class and the Employee class. An overridden toString method that returns the concatenation of name and hourlyWage instance variables (separated by a space). A method named paycheck that takes an integer parameter (# of hours) and returns a double. The method should calculate and return the total wage (# of hours * hourlyWage). You can assume that the hourlyWage instance variable will always have a format of a dollar sign followed by at least one digit. Compile your Employee class. 3. Create a class named EmployeeTest that has a main method. The class should do the following: Create an Employee object and pass in "Fred" for name, "RR 205" for office, and "$38.50" for hourlyWage. You may use a reference variable name of your choice. Call the toString method and print the result. ● ● ● ● ● A constructor that takes a String parameter and sets the instance variable. A properly named getter for the instance variable. ● ● A String instance variable called office (e.g. "LWH 2222"). A String instance variable called hourlywage (e.g. "$45.15"). Call the paycheck method, pass in a value of 20 for the parameter; print the result. Create a Person object and pass in "Fred" for name. You may use a reference variable name of your choice. Call the equals method on the "Fred" Employee object and pass in the reference to the "Fred" Person object. Print out the result. Compile and run your Employee Test class. Your output should match the sample output below: Fred $38.50 770.0 false
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 1GZ
Related questions
Question
100%

Transcribed Image Text:1. Create a properly encapsulated class named Person that has the following:
A String instance variable called name.
A constructor that takes a String parameter and sets the instance variable.
A properly named getter for the instance variable.
A correctly overrided equals method which returns true if the name instance variable of
the parameter and the reference variable calling the method are equal. (Do not forget the
null and class type checks!)
Compile your Person class.
2. Create a properly encapsulated class named Employee that inherits from Person and has the
following:
●
●
● A String instance variable called office (e.g. "LWH 2222").
A String instance variable called hourlywage (e.g. "$45.15").
A constructor that takes three Strings as parameters for name, office, and hourlyWage
and sets the instance variables of both the parent class and the Employee class.
An overridden toString method that returns the concatenation of name and hourlyWage
instance variables (separated by a space).
A method named paycheck that takes an integer parameter (# of hours) and returns a
double. The method should calculate and return the total wage (# of hours *
hourlyWage). You can assume that the hourlywage instance variable will always have a
format of a dollar sign followed by at least one digit.
Compile your Employee class.
3. Create a class named EmployeeTest that has a main method. The class should do the following:
Create an Employee object and pass in "Fred" for name, "RR 205" for office, and
"$38.50" for hourlyWage. You may use a reference variable name of your choice.
Call the toString method and print the result.
Call the paycheck method, pass in a value of 20 for the parameter; print the result.
Create a Person object and pass in "Fred" for name. You may use a reference variable
name of your choice.
●
●
●
●
Call the equals method on the "Fred" Employee object and pass in the reference to the
"Fred" Person object. Print out the result.
Compile and run your Employee Test class. Your output should match the sample output
below:
Fred $38.50
770.0
false
Expert Solution

Step 1
Here's the code for each class:
- Person class:
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return name.equals(person.name);
}
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
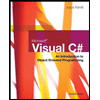
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
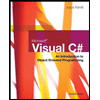
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,