Week2_Design_ender
.pdf
keyboard_arrow_up
School
University of Toronto *
*We aren’t endorsed by this school
Course
106
Subject
Mechanical Engineering
Date
Apr 3, 2024
Type
Pages
14
Uploaded by AmbassadorFang201 on coursehero.com
Week2_Design_ender
January 21, 2022
0.1
APS106 Lecture Notes - Week 2, Lecture 3
1
An Engineering Design Process for Programming
As you have seen in APS111/112, a key part of engineering is the design of objects, processes, and
systems.
From an engineering perspective, programming is the design, implementation, testing,
and documentation of a piece of software that solves a particular problem.
The software might
be part of a larger system (e.g., the avionics software of an aircraft, the accounting or human
resources software of a business), but it represents the solution to a design problem (or part of a
design problem).
We will therefore approach programing as an engineering design process and adapt the process you
have already seen.
1
1.1
An Engineering Design Process (for programming)
1.1.1
1. Define the Problem
Develop a clear and detailed problem statement. Be clear on what needs to be done. Sometimes the
problem will be easy enough (especially as you are learning programming) that the initial problem
statement given by the client/prof is suffcient. More often, the problem is complex enough that
forming a complete, explicit definition is a challenge itself and sometimes (even, often) the client
doesn’t really understand the problem him/herself. In such cases, research and iteration with the
client is necessary.
1.1.2
2. Define Test Cases
Work out specific test cases for which you know the answer.
This will help in the solidifying
the problem definition and provide you with tests once you have working code.
Try to cover a
reasonable span of possible cases that may come up. Think about strange cases that might break
the code. Think about reasonable measures of effciency, speed, and memory size.
1.1.3
3. Generate Many Creative Solutions
Think about solutions and write them down. Try to be as creative as possible.
A “solution” at this stage is two things:
1.
An Algorithm Plan
: a list of a few (from 4 or 5 to a dozen) steps that your algorithm will
execute to solve the problem. These are high-level steps that can correspond to many lines of
code. In real projects, these steps will themselves be subject to the design process (i.e. they
will in turn be broken down into sub-steps perhaps may layers deep).
2.
A Programming Plan
: a list of steps you will take in programming the algorithm. Some-
times this will be the form of programming, testing, and debugging each of the algorithm
steps in order.
But it doesn’t have to be that way.
Especially for larger systems, the al-
gorithm steps may be designed and implemented by different people in parallel or you may
choose to program, test, and debug the hardest step first to make sure you understand the
problem enough. Or you may decide to do the easiest steps first.
The point is that you program not by trying to write all the code at once and then hoping it all
works. Rather, you divide it up into a number of steps and make sure each step is implemented
and works as you proceed.
1.1.4
4. Select a Solution
Evaluate the algorithm and programming plans you have generated. Does it appear that this solu-
tion will truly solve the problem? You may write some prototype code to understand if particular
design ideas will work. Pick the best solution. If it is good enough, continue to Step 5, otherwise
return to an earlier step (maybe even Step 1 as you have uncovered new parts of the problem
definition).
1.1.5
5. Implement the Solution
Follow your chosen programming plan to implement the code. For each step in your programming
plan, you should ensure that the code is working: it runs some “sub-tests” correctly. Even though
2
it doesn’t solve the whole problem, it should produce intermediate results that you can verify are
correct. If it doesn’t, you should debug it before moving onto the next step. Implementation in-
cludes the documentation in the code: functions should have well-written docstrings and comments
should be used – it is better to over-comment than under-comment.
1.1.6
6. Perform Final Testing
Evaluate the solution against the test metrics, ensuring everything is in order. If the solution is not
satisfactory, you need to either return to Step 5 to debug the code or return to Step 1 to develop
a better understanding of the problem.
1.2
Final Remark: Design is Iterative
The above seems very proper and linear.
Real programming isn’t.
Real programming is a but
chaotic because you are creating something that doesn’t yet exist and figuring out how to solve the
problem as you go. Having some structure will help you not get lost.
One of the most essential parts of all engineering design processes is iteration. Programming is no
different. In fact, iteration may be even more important in programming because it is relatively
inexpensive to write prototype code (compared to, say, building a prototype engine). This means
that steps in the process are repeated over and over, in a loop. You might realize that you need
to jump back to an earlier step because you missed a key requirement or because you mistakenly
thought that you understood how to program a particular step. Each iteration brings with it an
increased level of understanding of the problem that deepens your knowledge. Iteration may allow
you to conceive solutions that were not initially apparent.
2
Design Project # 1: Forward Kinematics
2.1
Problem Background
If you have a robotic arm (e.g., the Canadarm) with joints, it is important to be able to calculate
where the end of the arm (i.e., the part usually used for picking something up) will be based on
the characteristics of the arm (e.g., the length of the components) and the angles of its joints.
Forward kinematics is the use of the kinematic equations of a robot to compute the position of the
end of the arm (end-effector) from specified values for the joint parameters. Forward kinematics is
3
used heavily in robotics, computer games, and animation.
2.2
1. Define the Problem
Given a robotic arm with two degrees of freedom (see above diagram), determine the position (x,y)
of the effector given the component-arm lengths and joint angles.
We need to find the
x
and
y
coordinates of the end of the arm. Those coordinates will obviously
depend on the location of the base of the arm. And so a relevant question to the client is if we
can define our own coordinate system or if there is a larger system that this arm is part of. Let’s
assume that we can define our own coordinate system.
Something to think about: how expensive will it be if this assumption is wrong? Will we have to
throw away all our work and start again? Or is there likely to be an easy way to take a solution
with a fixed coordinate system and reuse it in an externally specified coordinate system?
2.3
2. Define Test Cases
2.3.1
Test Case 1
len1 = 1, len2 = 1, ang1 = 60, ang2 = 30
End effector position x = 0.5, y = 1.87
2.3.2
Test Case 2
len1 = 1, len2 = 1, ang1 = 60, ang2 = -30
4
End effector position x = 1.37, y = 1.37
Where do these test cases come from? Either the client gives them to you or you have to figure out
from first principles (or research) how to calculate the answers by hand.
2.4
3. Generate Many Creative Solutions
Based on simple physics and math, we can obtain the (
∆
x
1
,
∆
y
1
) position of the end of the first
component arm.
∆
x
1
=
L
1
cos
(
θ
1
)
(1)
∆
y
1
=
L
1
sin
(
θ
1
)
(2)
Then we can obtain the (
∆
x
2
,
∆
y
2
) position for arm 2.
∆
x
2
=
L
2
cos
(
θ
2
+
θ
1
)
(3)
∆
y
2
=
L
2
sin
(
θ
2
+
θ
1
)
(4)
Finally we can find the (x,y) position by adding up the components.
x
= ∆
x
1
+ ∆
x
2
(5)
y
= ∆
y
1
+ ∆
y
2
(6)
These steps nicely form an Algorithm Plan
1. Get arm lengths and angles from the user.
2. Calculate (x,y) position of the end of arm 1.
3. Calculate the (x,y) position of the end of arm 2.
5
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
https://ethuto.cut.ac.za/webapps x
Content
Take Test: Main assessment link - x
ethuto.cut.ac.za/webapps/assessment/take/launch.jsp?course_assessment_id= 26832 1&course_id3_10885_1&content_id%3 625774 1&step3Dnull
Question Completion Status:
QUESTION 20
A single-stage, single-acting air compressor with runs at speed 600 rpm. It has a polytropic index of
1.26. The induced volume flow rate is 7.2 cubic meters per min and the air must be delivered at a
pressure of 668 kPa. The free air conditions are 101.3 kPa and 18 degrees Celsius. The clearance
volume is 6% of the stroke volume and the stroke/bore ratio is 1.6/1. Calculate the delivery air.
Hint answer usaully in C and no need for °C.
arrow_forward
You are a system engineer for the VRA, a power generation company in Ghana where there has been generation and transmission challenges facing the company ranging from frequent tripping of power lines, system imbalance, and overloading of transformers and to total system shut down of power plants. In a bid to solve the problem, management has requested from you to prepare a technical document of possible solution to fix the challenges. As a system engineer, how would you tackle the issues from the following point of view?
(a) i. Peak Load
ii. Base Load
iii. Energy mix
arrow_forward
The following tools / resources may be useful for you to complete the assignment:a. Chatgpt (You may use it to learn Matlab coding or any other computer language. An example is given here: https://shareg.pt/mXHGne9 ). Please take note that code generated by chatgpt can be directly copied and pasted.b. Matlabi) Useful cheat sheet (https://n.ethz.ch/~marcokre/download/ML-CheatSheet.pdf)ii) Getting started with Matlab (https://matlabacademy.mathworks.com/en/details/gettingstarted )iii) Getting 30-day Matlab trial license (https://www.mathworks.com/campaigns/products/trials.html ) iv) Polyfit (https://www.mathworks.com/help/matlab/ref/polyfit.html )v) Exponential Fit (https://www.mathworks.com/matlabcentral/answers/91159-how-do-i-fit-an-exponential-curve-to-my-data)c. PlotDigitizer (https://plotdigitizer.sourceforge.net/ ) or a free online app that does not requires installation (https://plotdigitizer.com/app )You may use your own engineering judgement to make any assumptions on any…
arrow_forward
Problem 1: You are working in a consulting company that does a lot of hand calculations for designs in
Aerospace Industry for mechanical, thermal, and fluidic systems. You took the Virtual engineering
course, and you want to convince your boss and the team you work to move to modelling and simulation
in computers using a certain software (Ansys, Abaqus, etc). Discuss the benefits and pitfalls of computer
based models used within an industrial environment to solve problems in engineering.
arrow_forward
The following tools / resources can be useda. Chatgptb. Matlab You may use your own engineering judgement to make any assumptions on any “missing” details of your scenario, provided that you state your assumption(s) and provide your justification(s).
arrow_forward
PLTW Engineering
Activity 3.8 Precision and Accuracy of
Measurement
Introduction
This concept of random and systematic errors is related to the precision and accuracy
of measurements. Precision characterizes the system's probability of providing the
same result every time a sample is measured (related to random error). Accuracy
characterizes the system's ability to provide a mean close to the true value when a
sample is measured many times (related to systematic error). We can determine the
precision of a measurement instrument by making repeated measurements of the same
sample and calculating the standard deviation of those measurements. However, we
will not be able to correct any single measurement due to a low precision instrument.
Simply stated, the effects of random uncertainties can be reduced by repeated
measurement, but it is not possible to correct for random errors.
We can determine the accuracy of a measurement instrument by comparing the
experimental mean of a large number…
arrow_forward
Content
WP NWP Assessment Builder UI Appl x
WP NWP Assessment Player UI Appli x
b My Questions | bartleby
bartleby premium - Twitter Searc x
+
i education.wiley.com/was/ui/v2/assessment-player/index.html?launchld=3bd9f9b2-6dae-43e7-a150-c87c490093e0#/question/0
e M1A4-ATTEMPT 1
Question 1 of 6
>
-/2
View Policies
Current Attempt in Progress
A particle which moves with curvilinear motion has coordinates in millimeters which vary with the time t in seconds according to x =
1.9t2 - 3.6t and y = 3.0t2 - t°/4.6. Determine the magnitudes of the velocity v and acceleration a and the angles which these vectors
make with thex-axis when t =7.0 s.
Answers: When t = 7.0 s,
V =
mm/s,
e, =
i
mm/s?, 0x =
i
a =
eTextbook and Media
Save for Later
Attempts: 0 of 1 used
Submit Answer
7:31 PM
O Type here to search
日
DEI
1/24/2022
...
II
arrow_forward
est 2 (copy) (page 4 of 9)
A wiseup.wsu.acza/mod/quiz/attempt.php7attempt=610918cmid 148960&page=3
ops
O YouTube
M Gmail
Maps
O GENERAL MATHEM.
O New Tab
:WSU WiSeUp
1 MONLO GOA
ashboard / My courses / FLM15B2_KF_WS6222 2021 / Tests / Test 2 (copy)
uestion 4
Quz navigation
Gate AB in Figure below is 1.0 m long and 0.9 wide. Calculate force F on the gate and position X of its centre of
Not yet
answered
pressure.
Marked out of
Finish attempt
10,000
Rag question
3m
Oil,
s.g.=Q81
7m
1.0m
B
50
Answer
arrow_forward
Scenario
You are assigned a role as a mechanical engineer for a vehicle design manufacturing company. Your
department has a software to perform numerical differentiation and integration. To be able to verify the
results of using the software and validate these results, your department manager has asked you to
analytically perform some tasks to validate the results generated by the software.
Q: is the last two digits of your student Id number. If your number is (20110092) then Q=92.
P: is the last digit of your student Id Number. If your number is (20110092) then P=2,
If that digit equals zero then use P=1. Example: If your number is (20110040) then P=1.
Task 1
Determine the gradient of following functions at the given points:
a) x(t) = (2t7 + P t-2)² + (6vi – 5) when t = 1
5s+7
b) v(s) =
when s = 3
(s²-P)2
c) i(t) = 5(1 – In(2t – 1) )
when t= 1 sec.
d) V(t) =5sin(100nt + 0.2) Volts , find i(t) = 10 × x10-6 dV©)
Ampere when t= 1ms.
dt
e) y(t) = e¬(t-n) sin(Qt + P)
when t = n radian
f)…
arrow_forward
In the automotive industry, supercars are highly accredited with how they are manufacture; from the
type of car chassis used to the type of materials employed. Often enough, companies like BMW,
Mercedes & Audi produces supercars that exemplifies a better reliability compared to other automotive
manufacturing companies. This is because they pay close attention to the details on how the car is
manufactured; right from raw materials to a finished supercar. The task given to you is to watch the
video link provided below & explain the electrostatic process acquired for the two different models of
BMW vehicles.
https://www.youtube.com/watch?v=sUqKUbmdOr0
Pls watch the video before answering
arrow_forward
ethuto.cut.ac.za/ultra/courses/_9063_1/cl/outline
Question Completion Status:
Moving to another question will save this response.
gestion 9
MECHANICAL AND CIVIL ENGINEERING
Two forces
and 2 are acting on a particle.
20 N
45°
30°
16N
The magnitude of the resultant force is:
O4 287+6.147
O B. 12.37 N
OC 28.67 N
D.
None of the above is correct
arrow_forward
QUESTION 1
In 2020, the world faces a critical health issue called Covid-19. During this period, the
government of Malaysia have implemented Movement Control Order (MCO) where many
sectors have been closed temporarily. This implementation has caused school to shut down and
all class activities conducted through online. Same goes to private sectors. This has caused
electricity usage increased rapidly.
AM
PM
Image courtesy : https://bit.ly/3eAqi7j)
As an owner of house in Figure 1, identify three renewable resources and elaborate how can
you utilise your house, to harvest energy from environment. In your explanation:
a.
Include the location of installation
b.
Any criteria or reason for the selection of (a)
Drawback or side effect of the chosen methods.
с.
d.
If structure need modification, explain the need of the modification (If any)
arrow_forward
Part 1: Do the Analysis of the Ocean Thermal Gradient Power Plant shown
below. Your Analysis will be easier to do in EES but it is up to you. Your
EES program must be well documented and documentation in your code
should reference system sketches. (The cycle and individual components)
These sketches are done on attached engineering or typing paper, unless
you are able to draw them in EES. You must validate your results with hand
calculations on engineering paper that invoke the 1 and 2 Law from the
perspective of the entire cycle, not the individual components. Of course,
a system sketch is required.
nd
1. An ocean thermal gradient power plant using a simple non-ideal Rankine
Cycle operates with a peak boiler temperature of 70 °F and a condenser
temperature of 40 °F. The warm surface water of the ocean is supplying
the thermal energy to the boiler. Assume a high source temperature of 80
°F. The cooler water deeper in the ocean is the sink for heat rejection at
the condenser. Assume a…
arrow_forward
kamihq.com/web/viewer.html?state%=D%7B"ids"%3A%5B"1vSrSXbH_6clkKyVVKKAtzZb_GOMRwrCG"%5D%...
lasses
Gmail
Copy of mom it for..
Маps
OGOld Telephone Ima.
Preview attachmen...
Kami Uploads ►
Sylvanus Gator - Mechanical Advantage Practice Sheet.pdf
rec
Times New Roman
14px
1.5pt
BIUSA
A Xa x* 三三
To find the Mechanical Advantage of ANY simple machine when given the force, use MA = R/E.
1.
An Effort force of 30N is appliled to a screwdriver to pry the lid off of a can of paint. The
screwdriver applies 90N of force to the lid. What is the MA of the screwdriver?
MA =
arrow_forward
.com question/22816263
rch for an answer to any question...
it works
For parents For teachers
Honor code
16 nours agO• Chemistry Hign Scnoo1
You are currently in a stable orbit 9000 km
(1km=1000m) from the center of planet Proxima
Centauri B.
According to the calculations done by NASA, Planet Proxima Centauri B has
a mass of approximately 7.6x10^24kg.
Based on fuel consumption and expected material aboard the ship, your
colony ship lander has a mass of approximately 1.1x10^5 kg.
1. Using Newton's Law of Universal Gravitation, determine what the force of
gravity on your ship at that orbit be using the early measurements
2.Using Newton's second law determine the acceleration of your lander due
to gravity
3.Upon arrival, you measure the actual force of gravity to be 6x10^5 N,which
ic difforent from vourcalclated vauein cuection 1 ldentify 2cnocific
e to search
arrow_forward
EARTHQUAKE ENGINEERING:
REFERENCE BOOK: SEISMIC DESIGN BY BESAVILLA
USE NSCP 2015 IN SOLVING THE PROBLEM BELOW.
arrow_forward
Berserk - Chapter 2- Read Berserk
compressor.
reggienet.illinoisstate.edu
https://reggienet.illinoisstate.edu/access/content/attachment/f6b18576-acf9-...
Hint. Both power-sizing and indexing will be used.
Ć
+
Question 3. The purchase price of a natural gas-fired commercial boiler (capacity X) was $181,000 eight years
ago. Another boiler of the same basic design, except with the capacity 1.42X, is currently being considered for
purchase. If it is purchased, some optional features presently costing $28,000 would be added for your
application. If the cost index was 162 for this type of equipment when the capacity X boiler was purchased and
is 221 now, and the applicable cost capacity factor is 0.8, what is your estimate of the purchase price for the
new boiler?
Hint: Use both indexing and power-sizing methods.
88
Illinois State University: TEC 330 001 FA2022 - Applied Economic Analysis For...
arrow_forward
As an automotive engineer, your role is to design a petrol engine for SUV car with fourstroke and four cylinder petrol engine. Describe the performance criteria need to beconsidered in detailing out the possible outcome of your spark ignition engine? Write asimple essay and an example of your design calculation to describe the prototype ofyour engine.
arrow_forward
Return to
Required information
Sometimes equations can be developed and practical problems solved by knowing nothing more than the dimensions of
the key parameters. For example, consider the heat loss through a window in a building. Window efficiency is rated in
terms of R value, which has the unit of ft2-hr-F/Btu. A certain manufacturer offers a double-pane window with R=2.5 and
also a triple-pane window with R=3.4. Both windows are 3.5 ft by 5 ft. On a given winter day, the temperature difference
between the inside and outside is 45°F.
Assume that a homeowner buys 20 such triple-pane windows for the house. A typical winter equals about 120 heating days at AT =
45°F. Each triple-pane window costs $85 more than a double-pane window. Ignoring interest and inflation, how many years will it take
the homeowner to make up the additional cost of the triple-pane windows from heating bill savings?
9.5 years
S
MacBook Air
!
arrow_forward
DETAILS
CHAPMANML6 1.CQ.008.
MY NOTES
ASK YOUR TEACHER
Complete the following sentence.
Instead of typing commands directly in the Command Window, a series of commands can be placed into a file, and the entire file can be executed by typing its name in the Command
Window. Such files are called
files.
arrow_forward
mechanical engineering works that can be related to algorithm
arrow_forward
permanent-magnet (pm) genera x
Bb Blackboard Learn
L STAND-ALONE.mp4 - Google Dri x
O Google Drive: ülwgjuó jc lis u
O ME526-WindEnergy-L25-Shuja.p x
O File | C:/Users/Administrator/Desktop/KFUPM%20Term%232/ME526/ME526-WindEnergy-L25-Shuja.pdf
(D Page view
A Read aloud
T) Add text
V Draw
Y Highlight
O Erase
17
of 26
Wind Farms
Consider the arrangement of three wind turbines in the following schematic in which wind
turbine C is in the wakes of turbines A and B.
Given the following:
- Uo = 12 m/s
A
-XẠC = 500 m
-XBC = 200 m
- z = 60 m
- Zo = 0.3 m
U.
-r, = 20 m
B
- CT = 0.88
Compute the total velocity deficit, udef(C) and the velocity at wind turbine C, namely Vc.
Activate Windows
Go to Settings to activate Windows.
Wind Farms (Example Answer)
5:43 PM
A 4)) ENG
5/3/2022
I!
arrow_forward
Question 45 is based on the following picture.
Reprinted with permission from Popular Science magazine,
©2000, Times Mirror Magazines, Inc.
45. Stuart Wilkinson, the engineer of "Chew-
Chew" said, "we stole the idea of eating
food from the biological world, but we are
marrying that idea to useful robotic
capabilities." Which of the following
would not be an application of Stuart
Wilkinson's theory?
A. A leaf mulcher that feeds on foliage
B. a trash compactor that feeds on
garbage
dos C. a lawn mower that feeds on grass
bos
9D. a garden cultivator that feeds on soil
clippings
E. a recycling truck that feeds on
petroleum
conjo
arrow_forward
A VẬT LÝ ĐẠI CƯƠ X
A TongHopTailieul x
A DaoHam.pdf - Go X
A Chương 1 - Good x
The position vec
My Tutoring | ba x
QUIZ 1: MOTION X
f (2) Facebook
O X
Slide 1
O YouTube
(66) YouTube
+ → C D
A Không bảo mật e-learning.hcmut.edu.vn/pluginfile.php?file=%2F1499051%2Fmod_resource%2Fcontent%2F8%2FChapter%201-%20Kinematics.pdf
A YouTube to MP3 C.
M Hộp thư đến (41) -...
Khóa học: Soft Skill.
O Audio Cambridge 1.
A TẠI LIEU WRITING.
t Link tải Cam 1- 15..
iot IELTS Simulation Te.
A (HV] Chương trình...
A BTC OISP GALA 202..
A GĐ2: OISP YOUTH...
A Tổng hợp đề writin.
A REPORT DE THI IEL.
>>
QUIZ 1: MOTION IN TWO AND THREE DIMENSIONS
1 / 5
195%
in meters and t is in seconds. At t = 3.00 s, find (a) the position of the particle, (b) its velocity, and
(c) its acceleration.
1.2. A motorist drives south at 20.0 m/s for 3.00 min, then turns west and travels at 25.0 m/s for 2.00
min, and finally travels northwest at 30.0 m/s for 1.00 min. For this 6.00-min trip, find (a) the total
vector displacement,…
arrow_forward
e IY: %VY OLD ?
د ميکانيك شهري 2021.pdf
UNIVERSITY OF BASRAH - COLLEGE OF ENGINEERING
DEPARTMENT OF CIVIL ENGINEERING
Subject: Engineering Mechanics-Static
Class: 1st year, 1st term
Exam. : Semester
Date: 16/3/2021
Examiner: Dr. Mazin, Dr. Aqeel, Dr.Ahid and Dr.Jaffar
Time: 1.5Hours
Q1] (10 Marks)
A- In Fig.A , If the resultant force acting on the bracket is to be 642 N directed
along the positive x axis, determine the magnitude of F.
B- In Fig.B , Determine the magnitude of the resultant force acting on the screw
eye and its direction measured clockwise from the x axis.
C- In Fig.C , Resolve the 100N force into components along the u and v axes, and
determine the magnitude of each of these components.
D- In Fig.D, Determine the moment of the force about point O.
300 N
60N
25°
32
45°
60°
505 N
50°
40N
Fig. A
50 N
Fig. B
400 N
100N
28°
18
2.12 m
Fig. C
2.12 m
Fig.D
Q2] (10 Marks)
A- In Fig.A , Replace the loading system by an equivalent resultant force and
specify where the resultant's…
arrow_forward
dated metncpdf
Thermodynamics An Engineering X
E Module2-chap2propertiesofpure x
O File
C:/Users/DANIEL/Desktop/300L%202ND%20SEMESTER%20MATERIALS/Module2-chap2propertiesofpuresubstances-130703012604 phpap.
ID Page view
A Read aloud
V Draw
H Highlight
O Erase
40
MEC 451 - THERMODYNAMICS
Faculty of Mechanical Engineering, UITM
Supplementary Problems
The pressure in an automobile tire depends on the temperature of the air
in the tire. When the air temperature is 25°C, the pressure gage reads 210
kRa. If the volume of the tire is 0.025 m3, Cetermine the pressure rise in
the tire when the air temperature in the tire rises to 50°C. Also, determine
the amount of air that must be bled off to restore pressure to its original
value at this temperature. Assume the atmospheric pressure is 100 kPa.
[ 26 kPa, 0.007 kg]
1.
A 6 4 2:57 PM
Lucky CORER
144
Tum lock
314
%
8
1/2
24
6
1/4
23
T
K
F
pause
B
arrow_forward
account_circle
Science
PhysicsQ&A LibraryA child’s toy consists of a m = 31 g monkey suspended from a spring of negligible mass and spring constant k. When the toy monkey is first hung on the spring and the system reaches equilibrium, the spring has stretched a distance of x = 17.6 cm, as shown in the diagram. This toy is so adorable you pull the monkey down an additional d = 7.6 cm from equilibrium and release it from rest, and smile with delight as it bounces playfully up and down. 1. Calculate the speed of the monkey, ve, in meters per second, as it passes through equilibrium. 2. Derive an expression for the total mechanical energy of the system as the monkey reaches the top of the motion, Etop, in terms of m, x, d, k, the maximum height above the bottom of the motion, hmax, and the variables available in the palette. 3. Calculate the maximum displacement, h, in centimeters, above the equilibrium position, that the monkey reaches.
A child’s toy consists of a…
arrow_forward
Intro to Transport Processes
TRUE or FALSE. Please provide a quick explanation thanks!
1. Each molecule of a system has a certain quantity of mass, thermal energy, or momentum associated with it.
2. Momentum transport in a fluid depends bulk movement of molecules and not on individual molecule of the system.
3. At same mass, the momentum of a molecule is greater than the other molecules if it has less velocity?
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
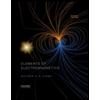
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
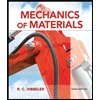
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
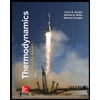
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
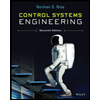
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
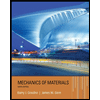
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
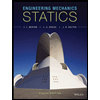
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- https://ethuto.cut.ac.za/webapps x Content Take Test: Main assessment link - x ethuto.cut.ac.za/webapps/assessment/take/launch.jsp?course_assessment_id= 26832 1&course_id3_10885_1&content_id%3 625774 1&step3Dnull Question Completion Status: QUESTION 20 A single-stage, single-acting air compressor with runs at speed 600 rpm. It has a polytropic index of 1.26. The induced volume flow rate is 7.2 cubic meters per min and the air must be delivered at a pressure of 668 kPa. The free air conditions are 101.3 kPa and 18 degrees Celsius. The clearance volume is 6% of the stroke volume and the stroke/bore ratio is 1.6/1. Calculate the delivery air. Hint answer usaully in C and no need for °C.arrow_forwardYou are a system engineer for the VRA, a power generation company in Ghana where there has been generation and transmission challenges facing the company ranging from frequent tripping of power lines, system imbalance, and overloading of transformers and to total system shut down of power plants. In a bid to solve the problem, management has requested from you to prepare a technical document of possible solution to fix the challenges. As a system engineer, how would you tackle the issues from the following point of view? (a) i. Peak Load ii. Base Load iii. Energy mixarrow_forwardThe following tools / resources may be useful for you to complete the assignment:a. Chatgpt (You may use it to learn Matlab coding or any other computer language. An example is given here: https://shareg.pt/mXHGne9 ). Please take note that code generated by chatgpt can be directly copied and pasted.b. Matlabi) Useful cheat sheet (https://n.ethz.ch/~marcokre/download/ML-CheatSheet.pdf)ii) Getting started with Matlab (https://matlabacademy.mathworks.com/en/details/gettingstarted )iii) Getting 30-day Matlab trial license (https://www.mathworks.com/campaigns/products/trials.html ) iv) Polyfit (https://www.mathworks.com/help/matlab/ref/polyfit.html )v) Exponential Fit (https://www.mathworks.com/matlabcentral/answers/91159-how-do-i-fit-an-exponential-curve-to-my-data)c. PlotDigitizer (https://plotdigitizer.sourceforge.net/ ) or a free online app that does not requires installation (https://plotdigitizer.com/app )You may use your own engineering judgement to make any assumptions on any…arrow_forward
- Problem 1: You are working in a consulting company that does a lot of hand calculations for designs in Aerospace Industry for mechanical, thermal, and fluidic systems. You took the Virtual engineering course, and you want to convince your boss and the team you work to move to modelling and simulation in computers using a certain software (Ansys, Abaqus, etc). Discuss the benefits and pitfalls of computer based models used within an industrial environment to solve problems in engineering.arrow_forwardThe following tools / resources can be useda. Chatgptb. Matlab You may use your own engineering judgement to make any assumptions on any “missing” details of your scenario, provided that you state your assumption(s) and provide your justification(s).arrow_forwardPLTW Engineering Activity 3.8 Precision and Accuracy of Measurement Introduction This concept of random and systematic errors is related to the precision and accuracy of measurements. Precision characterizes the system's probability of providing the same result every time a sample is measured (related to random error). Accuracy characterizes the system's ability to provide a mean close to the true value when a sample is measured many times (related to systematic error). We can determine the precision of a measurement instrument by making repeated measurements of the same sample and calculating the standard deviation of those measurements. However, we will not be able to correct any single measurement due to a low precision instrument. Simply stated, the effects of random uncertainties can be reduced by repeated measurement, but it is not possible to correct for random errors. We can determine the accuracy of a measurement instrument by comparing the experimental mean of a large number…arrow_forward
- Content WP NWP Assessment Builder UI Appl x WP NWP Assessment Player UI Appli x b My Questions | bartleby bartleby premium - Twitter Searc x + i education.wiley.com/was/ui/v2/assessment-player/index.html?launchld=3bd9f9b2-6dae-43e7-a150-c87c490093e0#/question/0 e M1A4-ATTEMPT 1 Question 1 of 6 > -/2 View Policies Current Attempt in Progress A particle which moves with curvilinear motion has coordinates in millimeters which vary with the time t in seconds according to x = 1.9t2 - 3.6t and y = 3.0t2 - t°/4.6. Determine the magnitudes of the velocity v and acceleration a and the angles which these vectors make with thex-axis when t =7.0 s. Answers: When t = 7.0 s, V = mm/s, e, = i mm/s?, 0x = i a = eTextbook and Media Save for Later Attempts: 0 of 1 used Submit Answer 7:31 PM O Type here to search 日 DEI 1/24/2022 ... IIarrow_forwardest 2 (copy) (page 4 of 9) A wiseup.wsu.acza/mod/quiz/attempt.php7attempt=610918cmid 148960&page=3 ops O YouTube M Gmail Maps O GENERAL MATHEM. O New Tab :WSU WiSeUp 1 MONLO GOA ashboard / My courses / FLM15B2_KF_WS6222 2021 / Tests / Test 2 (copy) uestion 4 Quz navigation Gate AB in Figure below is 1.0 m long and 0.9 wide. Calculate force F on the gate and position X of its centre of Not yet answered pressure. Marked out of Finish attempt 10,000 Rag question 3m Oil, s.g.=Q81 7m 1.0m B 50 Answerarrow_forwardScenario You are assigned a role as a mechanical engineer for a vehicle design manufacturing company. Your department has a software to perform numerical differentiation and integration. To be able to verify the results of using the software and validate these results, your department manager has asked you to analytically perform some tasks to validate the results generated by the software. Q: is the last two digits of your student Id number. If your number is (20110092) then Q=92. P: is the last digit of your student Id Number. If your number is (20110092) then P=2, If that digit equals zero then use P=1. Example: If your number is (20110040) then P=1. Task 1 Determine the gradient of following functions at the given points: a) x(t) = (2t7 + P t-2)² + (6vi – 5) when t = 1 5s+7 b) v(s) = when s = 3 (s²-P)2 c) i(t) = 5(1 – In(2t – 1) ) when t= 1 sec. d) V(t) =5sin(100nt + 0.2) Volts , find i(t) = 10 × x10-6 dV©) Ampere when t= 1ms. dt e) y(t) = e¬(t-n) sin(Qt + P) when t = n radian f)…arrow_forward
- In the automotive industry, supercars are highly accredited with how they are manufacture; from the type of car chassis used to the type of materials employed. Often enough, companies like BMW, Mercedes & Audi produces supercars that exemplifies a better reliability compared to other automotive manufacturing companies. This is because they pay close attention to the details on how the car is manufactured; right from raw materials to a finished supercar. The task given to you is to watch the video link provided below & explain the electrostatic process acquired for the two different models of BMW vehicles. https://www.youtube.com/watch?v=sUqKUbmdOr0 Pls watch the video before answeringarrow_forwardethuto.cut.ac.za/ultra/courses/_9063_1/cl/outline Question Completion Status: Moving to another question will save this response. gestion 9 MECHANICAL AND CIVIL ENGINEERING Two forces and 2 are acting on a particle. 20 N 45° 30° 16N The magnitude of the resultant force is: O4 287+6.147 O B. 12.37 N OC 28.67 N D. None of the above is correctarrow_forwardQUESTION 1 In 2020, the world faces a critical health issue called Covid-19. During this period, the government of Malaysia have implemented Movement Control Order (MCO) where many sectors have been closed temporarily. This implementation has caused school to shut down and all class activities conducted through online. Same goes to private sectors. This has caused electricity usage increased rapidly. AM PM Image courtesy : https://bit.ly/3eAqi7j) As an owner of house in Figure 1, identify three renewable resources and elaborate how can you utilise your house, to harvest energy from environment. In your explanation: a. Include the location of installation b. Any criteria or reason for the selection of (a) Drawback or side effect of the chosen methods. с. d. If structure need modification, explain the need of the modification (If any)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
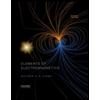
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
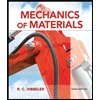
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
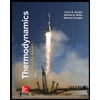
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
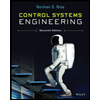
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
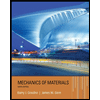
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
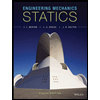
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY