You work for an loan analytics organization have been tasked with writing a program that simulates an analysis of loan applications. Code a modular program that uses parallel arrays to store loan application credit scores (values generated between 300 and 900), score ratings (poor, fair, good, very good, or exceptional based on credit score) and loan status (approved or declined applications based on credit score). The program must do the following: The program must first ask the user for the number of loan applications that will be in the simulation. This must be done by calling the getNumLoanApplications() function (definition below). After the input of the number of accounts, the program must use loops and random numbers to generate the credit score, score rating, and application result for each loan application in parallel arrays. These arrays must not be declared globally (major error). The following arrays must be declared and populated: creditScores[] - an array of type int that holds the credit score for the loan applications. The size of the array is determined by the number of loan applications the user entered in step 1. The values in this array represent the credit scores for each loan application. Credit scores for loan applications range from 300 - 900. scoreRatings[] - an array of type string to hold the credit score rating for the loan applications. The size of the array is determined by the number of loan applications the user entered in step 1. The values in the array represent the credit score rating for the loan application based on the credit score generated. In this simulation, credit scores can be one of the following: "poor" - Credit Score between 300 and 579 "fair" - Credit Score between 580 and 669 "good" - Credit Score between 670 and 739 "very good" - Credit Score between 740 and 799 "exceptional" - Credit Score between 800 and 900 loanStatuses[] - an array of type string to hold the status of each loan application. The size of the array is determined by the number of loan applications the user entered in step 1. The values in the array represent the status of each loan application based on the credit score generated. In this simulation, a loan application is "Approved" if the credit score generated is 630 or higher, otherwise the loan application is "Declined". Populate each of these arrays by using a loop that will execute based on the number of loan applications the user entered in step 1. On each pass, call the generateCreditScore function (definition below) to generate a loan application credit score and store in the creditScores[] array. Then, call the generateScoreRating() function (definition below) using the credit score generated to generate a score rating and store in the scoreRatings[] array. Finally, call the generateStatus() function (definition below) using the credit score generated to generate a loan status and store in the loanStatuses[] array. Once all of the arrays are populated, the program must display the result statistics of the simulation. This includes displaying the status, credit score and the credit score rating in each loan application along with total counts of loans approved, declined, and credit score rating category counts. This must be done by calling the analysis() (defined below) function that accepts all of the arrays as well as the number of loan applications generated and evaluated in the simulation the user entered in step 1. main(). In this simulation, credit scores can be one of the following: "poor" - Credit Score between 300 and 579 "fair" - Credit Score between 580 and 669 "good" - Credit Score between 670 and 739 "very good" - Credit Score between 740 and 799 "exceptional" - Credit Score between 800 and 900 instructions for this in picture output be line Enter the number of Loan Applications to be evaluated (min 50 - max 2000): 5000 Error : Invalid entry Enter the number of Loan Applications to be evaluated (min 50 - max 2000): -99 Error: Invalid entry Enter the number of Loan Applications to be evaluated (min 50 - max 2000): 1500 Application Status: Approved Credit score: 745 Score rating: Very good Application Status: Approved Credit score: 639 Score rating: good Application Status: Declined Credit score: 200 Score rating: poor Application Status: Approved Credit score: 800 Score rating: exceptional Application Status: Declined Credit score: 544 Score rating: poor After 1500 rows --------------------------------------------- Stats:- --------------------------------- Total Applications: 1500 Loans Approved: 700 Loans Declined: 800 ------------------------------------------ Total Score by rating: # "poor" - Credit Score between (300 - 579 ) 2 # "fair" - Credit Score between ( 580 - 669) 399 # "good" - Credit Score between (670 - 739) 299 # "very good" - Credit Score between (740 - 799) 186 # "exceptional" - Credit Score between (800 - 900) 2 ----------
You work for an loan analytics organization have been tasked with writing a program that simulates an analysis of loan applications.
Code a modular program that uses parallel arrays to store loan application credit scores (values generated between 300 and 900), score ratings (poor, fair, good, very good, or exceptional based on credit score) and loan status (approved or declined applications based on credit score).
The program must do the following:
- The program must first ask the user for the number of loan applications that will be in the simulation. This must be done by calling the getNumLoanApplications() function (definition below).
- After the input of the number of accounts, the program must use loops and random numbers to generate the credit score, score rating, and application result for each loan application in parallel arrays. These arrays must not be declared globally (major error).
- The following arrays must be declared and populated:
-
- creditScores[] - an array of type int that holds the credit score for the loan applications. The size of the array is determined by the number of loan applications the user entered in step 1. The values in this array represent the credit scores for each loan application. Credit scores for loan applications range from 300 - 900.
- scoreRatings[] - an array of type string to hold the credit score rating for the loan applications. The size of the array is determined by the number of loan applications the user entered in step 1. The values in the array represent the credit score rating for the loan application based on the credit score generated. In this simulation, credit scores can be one of the following:
- "poor" - Credit Score between 300 and 579
- "fair" - Credit Score between 580 and 669
- "good" - Credit Score between 670 and 739
- "very good" - Credit Score between 740 and 799
- "exceptional" - Credit Score between 800 and 900
- loanStatuses[] - an array of type string to hold the status of each loan application. The size of the array is determined by the number of loan applications the user entered in step 1. The values in the array represent the status of each loan application based on the credit score generated. In this simulation, a loan application is "Approved" if the credit score generated is 630 or higher, otherwise the loan application is "Declined".
- Populate each of these arrays by using a loop that will execute based on the number of loan applications the user entered in step 1. On each pass, call the generateCreditScore function (definition below) to generate a loan application credit score and store in the creditScores[] array. Then, call the generateScoreRating() function (definition below) using the credit score generated to generate a score rating and store in the scoreRatings[] array. Finally, call the generateStatus() function (definition below) using the credit score generated to generate a loan status and store in the loanStatuses[] array.
-
- Once all of the arrays are populated, the program must display the result statistics of the simulation. This includes displaying the status, credit score and the credit score rating in each loan application along with total counts of loans approved, declined, and credit score rating category counts. This must be done by calling the analysis() (defined below) function that accepts all of the arrays as well as the number of loan applications generated and evaluated in the simulation the user entered in step 1.
main(). In this simulation, credit scores can be one of the following:
-
- "poor" - Credit Score between 300 and 579
- "fair" - Credit Score between 580 and 669
- "good" - Credit Score between 670 and 739
- "very good" - Credit Score between 740 and 799
- "exceptional" - Credit Score between 800 and 900
- instructions for this in picture
output be line
Enter the number of Loan Applications to be evaluated (min 50 - max 2000): 5000
Error : Invalid entry
Enter the number of Loan Applications to be evaluated (min 50 - max 2000): -99
Error: Invalid entry
Enter the number of Loan Applications to be evaluated (min 50 - max 2000): 1500
Application Status: Approved Credit score: 745 Score rating: Very good
Application Status: Approved Credit score: 639 Score rating: good
Application Status: Declined Credit score: 200 Score rating: poor
Application Status: Approved Credit score: 800 Score rating: exceptional
Application Status: Declined Credit score: 544 Score rating: poor
After 1500 rows
---------------------------------------------
Stats:-
---------------------------------
Total Applications: 1500
Loans Approved: 700
Loans Declined: 800
------------------------------------------
Total Score by rating:
# "poor" - Credit Score between (300 - 579 ) 2
# "fair" - Credit Score between ( 580 - 669) 399
# "good" - Credit Score between (670 - 739) 299
# "very good" - Credit Score between (740 - 799) 186
# "exceptional" - Credit Score between (800 - 900) 2
----------



Step by step
Solved in 5 steps with 4 images

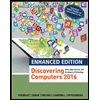
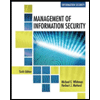
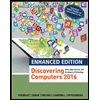
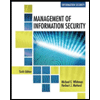