Using the LC-3 simulator, you will construct an assembly-level program that prompts the user for a string (the end of the string will be delimited by the character xOA, the ASCII character "Enter"). You will store the ASCII string representation of this input (including a terminating NULL character) in memory. The "Enter" character should not be considered part of the string and thus should not be stored. For the purposes of demonstration, I would like you to store the string at address x3100 (add the appropriate code to your program) and show that you have successfully stored the string by printing it back to the display using PUTS or PUTSP. Your program will be located in memory at location x3000.
Using the LC-3 simulator, you will construct an assembly-level program that prompts the user for a string (the end of the string will be delimited by the character xOA, the ASCII character "Enter"). You will store the ASCII string representation of this input (including a terminating NULL character) in memory. The "Enter" character should not be considered part of the string and thus should not be stored. For the purposes of demonstration, I would like you to store the string at address x3100 (add the appropriate code to your program) and show that you have successfully stored the string by printing it back to the display using PUTS or PUTSP. Your program will be located in memory at location x3000.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
-
; ************************************************************************************
-
-
; Things in parentheses are explanations, NOT additional steps.
-
-
;-------------------------------------------------------------------------------------
-
; START YOUR CODE HERE
-
; The stack pointer will initially point to xFDFE
-
; Try drawing the below as a picture of a stack using the addresses provided.
-
-
; The steps for the main GETSP subroutine are similar to the GETS subroutine in Part 1
-
-
GETSP ; Decrement R6 by #-1 (to allocate a word to store a return value) (R6: xFDFD)
-
; Decrement R6 by #-1 (this moves to push R7, our return address, onto the stack) (R6: xFDFC)
-
; Store contents of R7 at the address of R6 + #0 (to save a copy of the return address)
-
; Decrement R6 by #-1 again (this action allocates space to push R5, the previous functions's frame pointer, onto the stack) (R6: xFDFB)
-
; Store contents of R5 at the address of R6 + #0 (store previous frame pointer)
-
; Decrement R6 by #-1 and store into R5 (this sets up a new frame pointer) (R5: xFDFA)
-
; Decrement R6 by #-5 (this allocates space for local variables) (R6: xFDF6)
-
; Store contents of R0 at the memory address of R5 + #0 (In memory: xFDFA)
-
; Store contents of R1 at the memory address of R5 + #-1 (In memory: xFDF9)
-
; Store contents of R2 at the memory address of R5 + #-2 (In memory: xFDF8)
-
; Store contents of R3 at the memory address of R5 + #-3 (In memory: xFDF7)
-
; Store contents of R4 at the memory address of R5 + #-4 (In memory: xFDF6)
-
; Load from memory address R5 + #4 and put contents into R2. (This step gets the address parameter.) (In memory: xFDFE)
-
-
; LOOP reads in up to two characters, pack them into a single word, and store them in memory.
-
LOOP ; NOTE: Packed characters are of the form:
-
; (((C2 & 0x00FF) << 8) & 0xFF00) | (C1 & 0x00FF)
-
; Get character using GETC function call
-
; Print the character using the OUT function call
-
-
; Check for a linefeed using the following steps:
-
; Clear R1
-
; Decrement R1 by #-10 (#-13 on UNIX)
-
; Compare R1 and R0 and store the result in R1 (If they are equal, R1 will be 0)
-
; Branch IF NONZERO ONLY to the L2C subroutine
-
; Store contents of R1 at the memory address of R2 + #0 (else, we will have a line break if we do not branch)
-
; Unconditionally branch to DONE
-
-
; L2C (LOOP Second Character) is similar to LOOP, but with 3 new steps at the beginning. It handles the second character in the packed character pair given that the first character is not a line break.
-
L2C
-
; Copy the value in R0 into R4 (R4 serves as a temp register for the first character)
-
; Load CLU variable into R1 (HINT: CLU and CLL are variables so you can load them directly)
-
; Bitwise AND R4 with R1, and place the result into R4. (This will AND the upper half of R4 with zero, and clear them out, while the lower byte will be preserved.)
-
-
; Get character using GETC function call
-
; Print the character using the OUT function call
-
-
; Check for a linefeed using the following steps:
-
; Clear R1
-
; Add R1 and #-10 (#-13 on UNIX) and store the result in R1
-
; Add R1 and R0 and store the result in R1 (Comparison. If they are equal, R1 will be 0)
-
; Branch IF NONZERO ONLY to the LPC subroutine
-
; Store contents of R4 at the memory address of R2 + #0 (This will store the packed form of one character + a null character at our current position)
-
; Unconditionally branch to DONE
-
-
; LPC (LOOP Pack Characters) packs the two characters together, performing the
algorithm shown in the notes (assuming NEITHER is a line break) -
LPC ; Copy the character from R0 into R3
-
; Load CLU variable into R1
-
; Bitwise AND R3 with R1 and store into R3. (This will AND the upper half of R3 with zero, and clear them out, while the lower byte will be preserved.)
-
; Clear R0
-
; Add R0 and #8 and store in R0 (this initializes our "shift counter" to x0008)
-
; Copy R3 back into R1
-
-
; LS (LOOP Shift) is a loop that will repeat 8 times, shifting R1 left by 1 binary place value each iteration
-
LS ; Add R1 to itself (this will LEFT SHIFT the contents of R1 by 1 binary place value; please familiarize yourself with shifting and binary place values)
-
; Decrement R0 by #-1 (this decrements the shift counter by 1)
-
; Branch while positive back to LS (while we still have a positive value in the counter, we need to keep shifting)
-
-
; Copy R1 into R3
-
; Load CLL variable into R1
-
; Bitwise AND R3 with R1 and store into R3. (This will AND the lower half of R3 with zero, and clear them out, while the upper byte will be preserved. Because we've shifted left, we've MOVED the contents from the lower byte to the upper byte!)
-
; Add R3 and R4 and store into R4 (packing the characters together)
-
; Store the contents of R4 at the memory address of R2 + #0 (writes the packed character to the current place in the string)
-
; Increment R2 by #1 (incrementing our string pointer by 1)
-
; Branch unconditionally back to loop
-
-
DONE ;

Transcribed Image Text:In this lab you will construct an assembly routine that puts the ASCII representation of an input string in memory at a
specified location. The LC-3 machine language provides several useful TRAP calls for I/O (Patt 2e Appendix A, p 541). For
example, GETC (TRAP x20) gets one character from the keyboard and places its ASCII code in RO. OUT (TRAP x21)
performs the opposite function - it takes the contents of RO and outputs the specified character (using ASCII encoding)
to the display.
The LC-3 provides two output TRAPS for strings: PUTS (TRAP x22) and PUTSP (TRAP x24). Both of these expect (in RO) a
starting address for a string, and output the encoded string to the display. Your task is to produce two programs that
provide the "opposite" function of PUTS and PUTSP – that is, they take a string as input and place the string into memory
at a location specified in RO. You will create two separate stand-alone programs: one for GETS and one for GETSP. The
programs do not need to be implemented as subroutines or TRAP calls.
Using the LC-3 simulator, you will construct an assembly-level program that prompts the user for a string (the end of the
string will be delimited by the character xOA, the ASCII character “Enter”). You will store the ASCII string representation
of this input (including a terminating NULL character) in memory. The “Enter” character should not be considered part
of the string and thus should not be stored. For the purposes of demonstration, I would like you to store the string at
address x3100 (add the appropriate code to your program) and show that you have successfully stored the string by
printing it back to the display using PUTS or PUTSP. Your program will be located in memory at location x3000.
The following is an example output for both GETS.asm and GETSP.asm:
Enter string to echo: Go Raiders!
Go Raiders!
Halting the processor
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Recommended textbooks for you
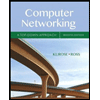
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
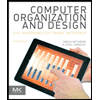
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
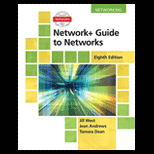
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
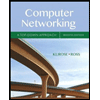
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
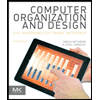
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
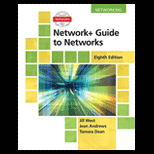
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
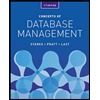
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
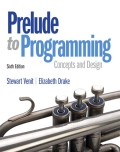
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
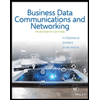
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY