Task: Design and implement an assembly language program using the Hack assembly language that can read input from the keyboard and display it to the simulated screen in the emulated CPU. The program should: Access the RAM address dedicated the keyboard input. Continuously check the keyboard input register for the keystroke. When a key is pressed, read the character. Create a bitmap image and display the character to the simulated screen. Upon pressing the Enter key, terminate the program. Requirements: The program should be written in the Hack assembly language. The program should use the nand2tetris tools to run on the emulated CPU. The program should correctly read input from the keyboard and display it to the simulated screen. The program should handle special keys such as Backspace and Enter appropriately. The program should be able to handle multiple simultaneous key presses. Starting code: // This file is part of www.nand2tetris.org // and the book "The Elements of Computing Systems" // by Nisan and Schocken, MIT Press. // Runs an infinite loop that listens to the keyboard input. // When a key is pressed (any key), the program blackens the screen, // i.e. writes "black" in every pixel; // the screen should remain fully black as long as the key is pressed. // When no key is pressed, the program clears the screen, i.e. writes // "white" in every pixel; // the screen should remain fully clear as long as no key is pressed. // Put your code here. (RESTART) @SCREEN D=A @0 M=D //PUT SCREEN START LOCATION IN RAM0 /////////////////////////// (KBDCHECK) @KBD D=M @BLACK D;JGT //JUMP IF ANY KBD KEYS ARE PRESSED @WHITE D;JEQ //ELSE JUMP TO WHITEN @KBDCHECK 0;JMP /////////////////////////// (BLACK) @1 M=-1 //WHAT TO FILL SCREEN WITH (-1=11111111111111) @CHANGE 0;JMP (WHITE) @1 M=0 //WHAT TO FILL SCREEN WITH @CHANGE 0;JMP ////////////////////////// (CHANGE) @1 //CHECK WHAT TO FILL SCREEN WITH D=M //D CONTAINS BLACK OR WHITE @0 A=M //GET ADRESS OF SCREEN PIXEL TO FILL M=D //FILL IT @0 D=M+1 //INC TO NEXT PIXEL @KBD D=A-D //KBD-SCREEN=A @0 M=M+1 //INC TO NEXT PIXEL A=M @CHANGE D;JGT //IF A=0 EXIT AS THE WHOLE SCREEN IS BLACK ///////////////////////// @RESTART 0;JMP
Task:
Design and implement an assembly language
- Access the RAM address dedicated the keyboard input.
- Continuously check the keyboard input register for the keystroke.
- When a key is pressed, read the character.
- Create a bitmap image and display the character to the simulated screen.
- Upon pressing the Enter key, terminate the program.
Requirements:
- The program should be written in the Hack assembly language.
- The program should use the nand2tetris tools to run on the emulated CPU.
- The program should correctly read input from the keyboard and display it to the simulated screen.
- The program should handle special keys such as Backspace and Enter appropriately.
- The program should be able to handle multiple simultaneous key presses.
Starting code:
// This file is part of www.nand2tetris.org
// and the book "The Elements of Computing Systems"
// by Nisan and Schocken, MIT Press.// Runs an infinite loop that listens to the keyboard input.
// When a key is pressed (any key), the program blackens the screen,
// i.e. writes "black" in every pixel;
// the screen should remain fully black as long as the key is pressed.
// When no key is pressed, the program clears the screen, i.e. writes
// "white" in every pixel;
// the screen should remain fully clear as long as no key is pressed.// Put your code here.
(RESTART)
@SCREEN
D=A
@0
M=D //PUT SCREEN START LOCATION IN RAM0///////////////////////////
(KBDCHECK)@KBD
D=M
@BLACK
D;JGT //JUMP IF ANY KBD KEYS ARE PRESSED
@WHITE
D;JEQ //ELSE JUMP TO WHITEN@KBDCHECK
0;JMP
///////////////////////////
(BLACK)
@1
M=-1 //WHAT TO FILL SCREEN WITH (-1=11111111111111)
@CHANGE
0;JMP(WHITE)
@1
M=0 //WHAT TO FILL SCREEN WITH
@CHANGE
0;JMP
//////////////////////////
(CHANGE)
@1 //CHECK WHAT TO FILL SCREEN WITH
D=M //D CONTAINS BLACK OR WHITE@0
A=M //GET ADRESS OF SCREEN PIXEL TO FILL
M=D //FILL IT@0
D=M+1 //INC TO NEXT PIXEL
@KBD
D=A-D //KBD-SCREEN=A@0
M=M+1 //INC TO NEXT PIXEL
A=M@CHANGE
D;JGT //IF A=0 EXIT AS THE WHOLE SCREEN IS BLACK
/////////////////////////
@RESTART
0;JMP

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

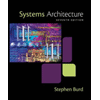
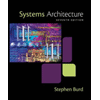