Need help with Python code ASAP - Code doesnt want to run yet gives no errors.My code:import math def getAreaRecs(recs): total_area = sum(rec["length"] * rec["width"] for rec in recs) return total_area def calculate_paint_needed(length, width, height, doors, windows): total_wall_area = 2 * (length + width) * height # Calculate the total wall area total_door_area = getAreaRecs(doors) # Calculate the total area of doors total_window_area = getAreaRecs(windows) # Calculate the total area of windows total_wall_area -= total_door_area + total_window_area # Subtract the areas of doors and windows from the total wall area gallons_needed = total_wall_area / 100.0 # Calculate the amount of paint needed (assuming 1 gallon covers 100 sq. ft.) return gallons_needed def get_valid_input(prompt, validation_func): while True: try: value = input(prompt) value = float(value) if validation_func(value): return value else: print("Invalid input. Please try again.") except ValueError: print("Invalid input. Please enter a valid number.") def validate_positive(value): """ Validate that a value is positive (greater than 0). Args: value (float): The value to validate. Returns: bool: True if the value is positive, False otherwise. """ return value > 0 def main(): # Open output file for writing with open("output.txt", "w") as output_file: # Get room dimensions from the user length = get_valid_input("Enter the length of the room in feet: ", validate_positive) output_file.write(f"Length of the room: {length} feet\n") width = get_valid_input("Enter the width of the room in feet: ", validate_positive) output_file.write(f"Width of the room: {width} feet\n") height = get_valid_input("Enter the height of the room in feet: ", validate_positive) output_file.write(f"Height of the room: {height} feet\n") total_wall_area = 2 * (length + width) * height print("Total wall area before subtracting doors and windows:", total_wall_area) output_file.write(f"Total wall area before subtracting doors and windows: {total_wall_area}\n") print("Excellent, let's get your windows and doors in now.") output_file.write("Excellent, let's get your windows and doors in now.\n") # Get door information from the user num_doors = int(get_valid_input("Enter the number of doors (must be greater than 0): ", validate_positive)) output_file.write(f"Number of doors: {num_doors}\n") doors = [{"length": get_valid_input(f"Enter the length of door {i+1} in feet: ", validate_positive), "width": get_valid_input(f"Enter the width of door {i+1} in feet: ", validate_positive)} for i in range(num_doors)] for i, door in enumerate(doors, start=1): output_file.write(f"Door {i}: Length = {door['length']}, Width = {door['width']}\n") # Get window information from the user num_windows = int(get_valid_input("Enter the number of windows: ", validate_positive)) output_file.write(f"Number of windows: {num_windows}\n") windows = [{"length": get_valid_input(f"Enter the length of window {i+1} in feet: ", validate_positive), "width": get_valid_input(f"Enter the width of window {i+1} in feet: ", validate_positive)} for i in range(num_windows)] for i, window in enumerate(windows, start=1): output_file.write(f"Window {i}: Length = {window['length']}, Width = {window['width']}\n") # Calculate and print the amount of paint needed gallons_needed = calculate_paint_needed(length, width, height, doors, windows) print(f"You will need to buy {math.ceil(gallons_needed)} gallons of paint.") output_file.write(f"You will need to buy {math.ceil(gallons_needed)} gallons of paint.\n") print("You will need exactly", gallons_needed, "gallons of paint for the walls after subtracting the doors and windows.")
Need help with Python code ASAP - Code doesnt want to run yet gives no errors.
My code:
import math
def getAreaRecs(recs):
total_area = sum(rec["length"] * rec["width"] for rec in recs)
return total_area
def calculate_paint_needed(length, width, height, doors, windows):
total_wall_area = 2 * (length + width) * height # Calculate the total wall area
total_door_area = getAreaRecs(doors) # Calculate the total area of doors
total_window_area = getAreaRecs(windows) # Calculate the total area of windows
total_wall_area -= total_door_area + total_window_area # Subtract the areas of doors and windows from the total wall area
gallons_needed = total_wall_area / 100.0 # Calculate the amount of paint needed (assuming 1 gallon covers 100 sq. ft.)
return gallons_needed
def get_valid_input(prompt, validation_func):
while True:
try:
value = input(prompt)
value = float(value)
if validation_func(value):
return value
else:
print("Invalid input. Please try again.")
except ValueError:
print("Invalid input. Please enter a valid number.")
def validate_positive(value):
"""
Validate that a value is positive (greater than 0).
Args:
value (float): The value to validate.
Returns:
bool: True if the value is positive, False otherwise.
"""
return value > 0
def main():
# Open output file for writing
with open("output.txt", "w") as output_file:
# Get room dimensions from the user
length = get_valid_input("Enter the length of the room in feet: ", validate_positive)
output_file.write(f"Length of the room: {length} feet\n")
width = get_valid_input("Enter the width of the room in feet: ", validate_positive)
output_file.write(f"Width of the room: {width} feet\n")
height = get_valid_input("Enter the height of the room in feet: ", validate_positive)
output_file.write(f"Height of the room: {height} feet\n")
total_wall_area = 2 * (length + width) * height
print("Total wall area before subtracting doors and windows:", total_wall_area)
output_file.write(f"Total wall area before subtracting doors and windows: {total_wall_area}\n")
print("Excellent, let's get your windows and doors in now.")
output_file.write("Excellent, let's get your windows and doors in now.\n")
# Get door information from the user
num_doors = int(get_valid_input("Enter the number of doors (must be greater than 0): ", validate_positive))
output_file.write(f"Number of doors: {num_doors}\n")
doors = [{"length": get_valid_input(f"Enter the length of door {i+1} in feet: ", validate_positive),
"width": get_valid_input(f"Enter the width of door {i+1} in feet: ", validate_positive)}
for i in range(num_doors)]
for i, door in enumerate(doors, start=1):
output_file.write(f"Door {i}: Length = {door['length']}, Width = {door['width']}\n")
# Get window information from the user
num_windows = int(get_valid_input("Enter the number of windows: ", validate_positive))
output_file.write(f"Number of windows: {num_windows}\n")
windows = [{"length": get_valid_input(f"Enter the length of window {i+1} in feet: ", validate_positive),
"width": get_valid_input(f"Enter the width of window {i+1} in feet: ", validate_positive)}
for i in range(num_windows)]
for i, window in enumerate(windows, start=1):
output_file.write(f"Window {i}: Length = {window['length']}, Width = {window['width']}\n")
# Calculate and print the amount of paint needed
gallons_needed = calculate_paint_needed(length, width, height, doors, windows)
print(f"You will need to buy {math.ceil(gallons_needed)} gallons of paint.")
output_file.write(f"You will need to buy {math.ceil(gallons_needed)} gallons of paint.\n")
print("You will need exactly", gallons_needed, "gallons of paint for the walls after subtracting the doors and windows.")

Step by step
Solved in 2 steps

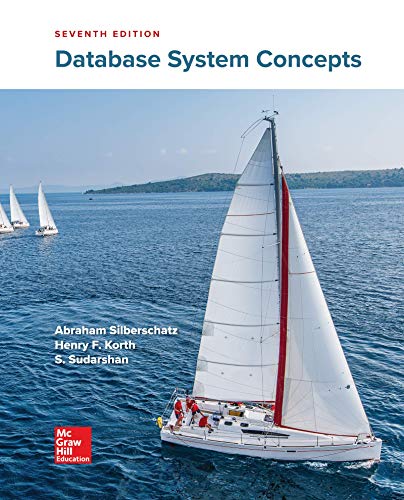
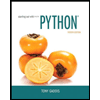
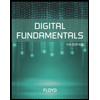
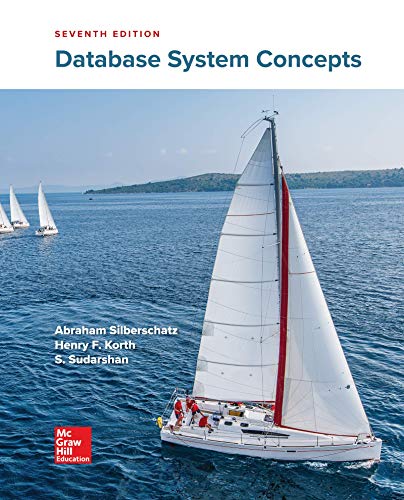
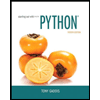
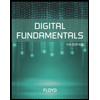
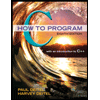
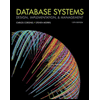
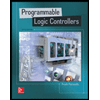