Hello, I wanted some help fixing my code. I want my player class to draw 5 cards and avoid duplicates. I don't plan on using a set, so I want to shuffle the cards and then draw, however, It seems my shuffle isn't working. When i ran the program, i got duplicates as shown in the screenshot. I will paste my code here too if you'd like to see what I did and where i might be wrong. I'd love some help understanding how to fix it with explanations if possible. Or if there's a better solution with the shuffle, I'd be open to that as well. please and thank you. this is my CARD CLASS: public class Card { public static final int ACE = 1; public static final int TWO = 2; public static final int THREE = 3; public static final int FOUR = 4; public static final int FIVE = 5; public static final int SIX = 6; public static final int SEVEN = 7; public static final int EIGHT = 8; public static final int NINE = 9; public static final int TEN = 10; public static final int JACK= 11; public static final int QUEEN = 12; public static final int KING = 13; public static final int CLUBS = 1; public static final int DIAMONDS = 2; public static final int HEARTS = 3; public static final int SPADES = 4; public int face, suit; public String faceName, suitName; Random rand = new Random(); public Card() { int randomFace = rand.nextInt(13) + 1; int randomSuit = rand.nextInt(4) + 1; face = randomFace; suit= randomSuit; } public Card(int face, int suit) { this.face = face; this.suit = suit; } @Override public String toString() { switch(face) { case ACE: faceName = "Ace"; break; case TWO: faceName = "Two"; break; case THREE: faceName = "Three"; break; case FOUR: faceName = "Four"; break; case FIVE: faceName = "Five"; break; case SIX: faceName = "Six"; break; case SEVEN: faceName = "Seven"; break; case EIGHT: faceName = "Eight"; break; case NINE: faceName = "Nine"; break; case TEN: faceName = "Ten"; break; case JACK: faceName = "Eleven"; break; case QUEEN: faceName = "Twelve"; break; case KING: faceName = "Thirteen"; break; } switch(suit) { case CLUBS: suitName = "Clubs"; break; case DIAMONDS: suitName = "Diamonds"; case HEARTS: suitName = "Hearts"; case SPADES: suitName = "Spades"; } return faceName + " of " + suitName; } } this is my PLAYER class: public class Player { private Card[]cards; private int numCards; Random rand = new Random(); public Player() { cards = new Card[5]; } public void deck() { Card[] deckOfCards = new Card[52]; int cardIndex = 0; // index of top card (==next card to deal) for (int face = Card.ACE; face <= Card.KING; face++) for (int suit = Card.CLUBS; suit <= Card.SPADES; suit++) deckOfCards[cardIndex++] = new Card(face, suit); this.numCards = 52; } public void shuffle(Player deck) { // add R. Sedgewick's video code (shown in Class 7) int N = deck.numCards; for (int i = 0; i < N; i++) { int r = rand.nextInt(i + 1); exch(deck, i, r); } } private void exch(Player deck, int i, int r) { Card swap = cards[r]; cards[r] = cards[i]; cards[i] = swap; } public void drawCards() { for (int i=0; i<5; i++) { cards[i] = new Card(); } } @Override public String toString() { String cardsToString = ""; for (int i = 0; i < cards.length; i++) { cardsToString=cardsToString+cards[i].toString()+"\n"; } return cardsToString; } } this is my DRIVER CLASS: public class Driver { public static void main(String[]args) { Player b = new Player(); b.drawCards(); System.out.println(b); } }
Hello, I wanted some help fixing my code. I want my player class to draw 5 cards and avoid duplicates. I don't plan on using a set, so I want to shuffle the cards and then draw, however, It seems my shuffle isn't working. When i ran the program, i got duplicates as shown in the screenshot. I will paste my code here too if you'd like to see what I did and where i might be wrong. I'd love some help understanding how to fix it with explanations if possible. Or if there's a better solution with the shuffle, I'd be open to that as well. please and thank you.
this is my CARD CLASS:
public class Card
{
public static final int ACE = 1;
public static final int TWO = 2;
public static final int THREE = 3;
public static final int FOUR = 4;
public static final int FIVE = 5;
public static final int SIX = 6;
public static final int SEVEN = 7;
public static final int EIGHT = 8;
public static final int NINE = 9;
public static final int TEN = 10;
public static final int JACK= 11;
public static final int QUEEN = 12;
public static final int KING = 13;
public static final int CLUBS = 1;
public static final int DIAMONDS = 2;
public static final int HEARTS = 3;
public static final int SPADES = 4;
public int face, suit;
public String faceName, suitName;
Random rand = new Random();
public Card()
{
int randomFace = rand.nextInt(13) + 1;
int randomSuit = rand.nextInt(4) + 1;
face = randomFace;
suit= randomSuit;
}
public Card(int face, int suit)
{
this.face = face;
this.suit = suit;
}
@Override
public String toString()
{
switch(face)
{
case ACE:
faceName = "Ace";
break;
case TWO:
faceName = "Two";
break;
case THREE:
faceName = "Three";
break;
case FOUR:
faceName = "Four";
break;
case FIVE:
faceName = "Five";
break;
case SIX:
faceName = "Six";
break;
case SEVEN:
faceName = "Seven";
break;
case EIGHT:
faceName = "Eight";
break;
case NINE:
faceName = "Nine";
break;
case TEN:
faceName = "Ten";
break;
case JACK:
faceName = "Eleven";
break;
case QUEEN:
faceName = "Twelve";
break;
case KING:
faceName = "Thirteen";
break;
}
switch(suit)
{
case CLUBS:
suitName = "Clubs";
break;
case DIAMONDS:
suitName = "Diamonds";
case HEARTS:
suitName = "Hearts";
case SPADES:
suitName = "Spades";
}
return faceName + " of " + suitName;
}
}
this is my PLAYER class:
public class Player
{
private Card[]cards;
private int numCards;
Random rand = new Random();
public Player()
{
cards = new Card[5];
}
public void deck()
{
Card[] deckOfCards = new Card[52];
int cardIndex = 0; // index of top card (==next card to deal)
for (int face = Card.ACE; face <= Card.KING; face++)
for (int suit = Card.CLUBS; suit <= Card.SPADES; suit++)
deckOfCards[cardIndex++] = new Card(face, suit);
this.numCards = 52;
}
public void shuffle(Player deck)
{
// add R. Sedgewick's video code (shown in Class 7)
int N = deck.numCards;
for (int i = 0; i < N; i++)
{
int r = rand.nextInt(i + 1);
exch(deck, i, r);
}
}
private void exch(Player deck, int i, int r) {
Card swap = cards[r];
cards[r] = cards[i];
cards[i] = swap;
}
public void drawCards()
{
for (int i=0; i<5; i++)
{
cards[i] = new Card();
}
}
@Override
public String toString()
{
String cardsToString = "";
for (int i = 0; i < cards.length; i++)
{
cardsToString=cardsToString+cards[i].toString()+"\n";
}
return cardsToString;
}
}
this is my DRIVER CLASS:
public class Driver
{
public static void main(String[]args)
{
Player b = new Player();
b.drawCards();
System.out.println(b);
}
}
![5
60
7
8
10
11
12
13 }
14
public static void main(String[] args)
{
}
Player b = new Player ();
b.drawCards ();
System.out.println(b);
Problems @Javadoc
Declaration
Console X
<terminated> Driver (1) [Java Application] C:\Program Files\Java\jdk-17.0.4\bin\javaw.exe (Feb 18, 2023, 8:02:32 PM - 8:02:32 PM)
Eight of Spades
Six of Spades
Eight of Spades
Eleven of Clubs
Twelve of Spades](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd99806c6-42e8-450c-a219-a2d748677dac%2F8e5e9ce5-73af-4f38-97ef-3184ee28b377%2Fuifyuqt_processed.jpeg&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

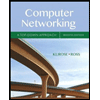
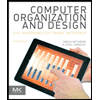
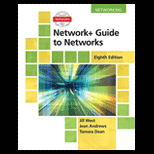
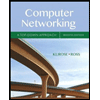
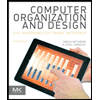
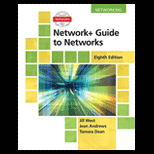
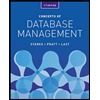
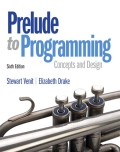
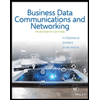