C Programming: Need help in how to create the code instrumentation in c. We will rely on gcc's option -finstrument-functions to execute code to collect data at the start and end of invoking any function in a program. This is also called code profiling. For this, you need to implement the following functions in this unit: void __cyg_profile_func_enter(void *this_fn, void *call_site); void __cyg_profile_func_exit(void *this_fn, void *call_site); When we compile our programs with the above-mentioned option, function__cyg_profile_func_enter() will be called at the beginning of all functions in our program and function __cyg_profile_func_exit() will be called at the end of those functions. Parameters this_fn and call_site indicate pointers to the code segment that refer to the profiled function and the instruction that has invoked the profiled function. We can implement various functionalities using these enter/exit functions to help us analyze the function calls in our program. In this project, you will collect information about stack frames and simple execution time of each function. you need to collect the following information for each call. It is recommended that you maintain a linked list of structures to hold and update the information for each function call. You may choose to store additional information as needed too. Check the test.c program yourself and observe how the executable with code instrumentation behaves differently. Here is the test.c file: #include #include void f1(int x) { for (int i = 0; i < 100000; i++) { // Just a busy loop to waste some CPU time asm(""); } char buffer[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; // Local array that is updated later in the function int local_int = 0x89abcdef; // a recognizable local variable on the stack for (int i = 0; i < sizeof(buffer); i++) { // Update and print the buffer buffer[i] = x; printf("%u ", buffer[i]); } printf("\n"); if (x > 1) { // Recursively call f1() f1(x - 1); } } int main(void) { f1(3); f1(1); return 0; } Please show the code and the full output in the terminal. There must be no error in the code.
C Programming:
Need help in how to create the code instrumentation in c.
We will rely on gcc's option -finstrument-functions to execute code to collect data at the start and end of invoking any function in a program. This is also called code profiling. For this, you need to implement the following functions in this unit:
When we compile our programs with the above-mentioned option, function__cyg_profile_func_enter() will be called at the beginning of all functions in our program and function __cyg_profile_func_exit() will be called at the end of those functions. Parameters this_fn and call_site indicate pointers to the code segment that refer to the profiled function and the instruction that has invoked the profiled function.
We can implement various functionalities using these enter/exit functions to help us analyze the function calls in our program. In this project, you will collect information about stack frames and simple execution time of each function. you need to collect the following information for each call. It is recommended that you maintain a linked list of structures to hold and update the information for each function call. You may choose to store additional information as needed too. Check the test.c program yourself and observe how the executable with code instrumentation behaves differently.
Here is the test.c file:
#include <stdio.h>
#include <unistd.h>
void f1(int x) {
for (int i = 0; i < 100000; i++) { // Just a busy loop to waste some CPU time
asm("");
}
char buffer[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; // Local array that is updated later in the function
int local_int = 0x89abcdef; // a recognizable local variable on the stack
for (int i = 0; i < sizeof(buffer); i++) { // Update and print the buffer
buffer[i] = x;
printf("%u ", buffer[i]);
}
printf("\n");
if (x > 1) { // Recursively call f1()
f1(x - 1);
}
}
int main(void) {
f1(3);
f1(1);
return 0;
}
Please show the code and the full output in the terminal. There must be no error in the code.

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Please write this code in one file and execute the same output. Make sure to show the full code and output in the terminal.
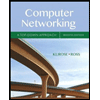
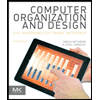
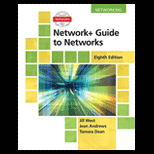
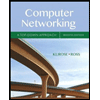
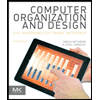
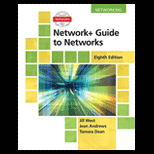
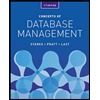
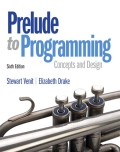
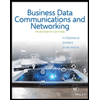