Basic Java Help Each year, the Social Security Administration provides a list of baby names for boy and girls by state. You will write a program to read a file containing a list of baby names and display the top names. To start, implement the following class: baby names util = printTopNames(File, int): void printTopNames Will be a static method. It will accept two parameters, one is a File containing the list of names. The other is an integer that indicates how many names to display (ie, top 10 names, or top 50 names, etc). The integer argument could be any valid integer. You do not have to worry about an integer value large than 5 for the boy/girl names. This method should open the file and parse the file and print the names as shown below: Your program will use the Scanner class to read the data from the file (see your text for examples). You will also need to use a delimiter with the scanner so that the scanner will break the line apart based on the commas. For this file, set the delimiter on the scanner to this regular expression: ",|\\r\\n". This will split the line on commas and end of line. Once you setup your Scanner instance with the delimiter, you should be able to process the line using the typical Scanner method calls like next(), nextInt(). Exceptions If the file that is passed in cannot be found, then the Scanner will throw a FileNotFoundException. Your code should handle this exception and not re-throw it. In your handler, print an error message that the file cannot be found, along with the file name. If this exception happens, then processing should be halter after printing the information. The baby names should separated by commas. Each line has the following structure: STATE,GENDER,YEAR,NAME,RANK Output Your code should use printf() with the proper format specifiers to produce a 3 column output that shows the most popular names for both boys and girls. Column 1 is the ranking, Column 2 is the boy's name, and Column 3 is the girls name. All 3 columns should be left justified. Should look very similar to the sample output. A client might call your class as follows, passing in a file and asking to display the top 6 names. File f = new File("floridaBabyNames2021-2.txt"); BabyNamesUtil.printTopNames(f,6); Your code produce the following output. Baby Name Rankings Boys Girls ---- ----- 1 Liam Olivia 2 Noah Emma 3 Lucas Isabella 4 Elijah Mia 5 Oliver Sophia 6 Benjamin Ava
Basic Java Help
Each year, the Social Security Administration provides a list of baby names for boy and girls by state. You will write a program to read a file containing a list of baby names and display the top names.
To start, implement the following class: baby names util = printTopNames(File, int): void
printTopNames
Will be a static method. It will accept two parameters, one is a File containing the list of names. The other is an integer that indicates how many names to display (ie, top 10 names, or top 50 names, etc). The integer argument could be any valid integer.
You do not have to worry about an integer value large than 5 for the boy/girl names.
This method should open the file and parse the file and print the names as shown below:
Your program will use the Scanner class to read the data from the file (see your text for examples). You will also need to use a delimiter with the scanner so that the scanner will break the line apart based on the commas. For this file, set the delimiter on the scanner to this regular expression: ",|\\r\\n". This will split the line on commas and end of line.
Once you setup your Scanner instance with the delimiter, you should be able to process the line using the typical Scanner method calls like next(), nextInt().
Exceptions
If the file that is passed in cannot be found, then the Scanner will throw a FileNotFoundException. Your code should handle this exception and not re-throw it. In your handler, print an error message that the file cannot be found, along with the file name. If this exception happens, then processing should be halter after printing the information.
The baby names should separated by commas. Each line has the following structure: STATE,GENDER,YEAR,NAME,RANK
Output
Your code should use printf() with the proper format specifiers to produce a 3 column output that shows the most popular names for both boys and girls. Column 1 is the ranking, Column 2 is the boy's name, and Column 3 is the girls name. All 3 columns should be left justified. Should look very similar to the sample output.
A client might call your class as follows, passing in a file and asking to display the top 6 names.
File f = new File("floridaBabyNames2021-2.txt");
BabyNamesUtil.printTopNames(f,6);
Your code produce the following output.
Baby Name Rankings
Boys Girls
---- -----
1 Liam Olivia
2 Noah Emma
3 Lucas Isabella
4 Elijah Mia
5 Oliver Sophia
6 Benjamin Ava

Step by step
Solved in 4 steps with 1 images

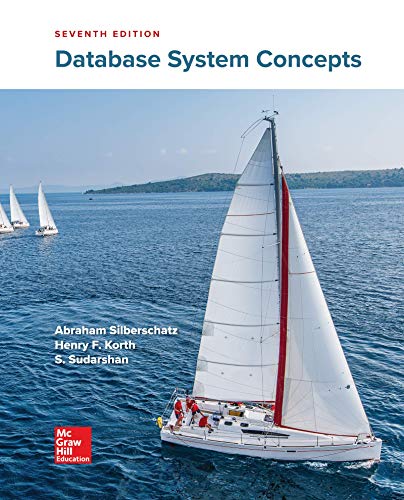
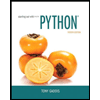
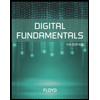
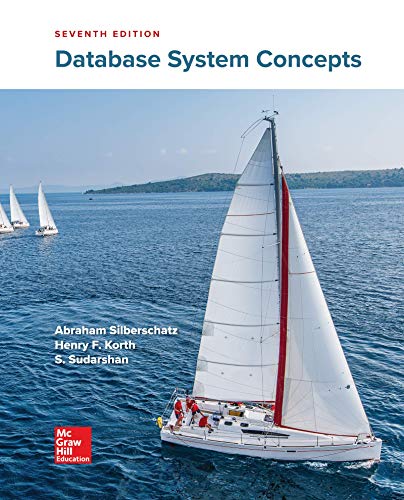
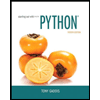
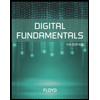
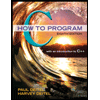
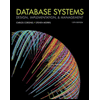
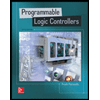